Introduction: 74LS Series Digital Logic Tester
*Update* Featured on www.freetronics.com (http://www.freetronics.com/blogs/news/11619617-build-a-74ls-series-digital-logic-tester-with-arduino#.UtgAf9JDumE)!
In the following instructable, I will demonstrate how to design a circuit and write a test program, using the Arduino Nano, that will test two input Transistor-Transistor Logic (TTL) gates including AND, OR, NAND, and XOR. The circuit/program designed will implement a basic two input “truth table” applying ALL possible inputs to the gate(s) while reading back the results to the Arduino Serial Monitor and the LEDs. This very simple circuit and code will demonstrate one of the basic building blocks of Digital Logic. I will try to make this instructable as easy to follow as possible, and if you still have some troubles, please feel free to get in contact with me. Continue onto the next step for the hardware and software requirements.
Step 1: Gather Materials
Being a college student means that I am on a tight budget, thus this project was perfect for me because of how economic it was. The most expensive part was the Arduino, but that can always be easily removed and reprogrammed for use on other projects. The total cost for this project should come out to be $15-20.
Hardware List:
• Arduino Nano (any 5v Arduino of your choosing will work).
• 74LS Series ICs (http://www.futurlec.com/IC74LS00Series.shtml).
• LEDs (http://www.ebay.com/itm/100pcs-3mm-LED-Red-Green-Blue-Yellow-White-Ultra-Bright-Light-Bulbs-Lamp-8000mcd-/300913115328?pt=LH_DefaultDomain_0&hash=item460fd1c0c0).
• Breadboard (a mini breadboard was perfect in size).
• Wire
Software List:
• Arduino IDE (my version is 1.0.5).
As you can see the parts list is very minimal. If you have any questions regarding component compatibility, I'd be more than happy to help. Continue onto the next step for the circuit construction.
Step 2: Building the Circuit
As you can see, the circuit is very simple. Make sure you remember the polarity of the LEDs! Also, make sure you understand which pair of bits each LED represents, to help you, I have labeled the inputs to each LED in the schematic. To make the instructable easier to follow, I have also included the two input truth table for each of the gates, as you can see there.
Now, some of you might be wondering why I left out the NOR gate in this instructable. That is because it's I/O is the opposite of the other gates, as you can see in the connection diagrams pictured there. If you have any questions regarding the circuit, please ask. Continue onto the next step for the programming part.
Step 3: Programming
Throughout the code I set inputs as, (Low, Low), (Low, High), (High, Low), and (High, High) just like a truth table. After we assign these values with High or Low we provide an if statement to say if the input is High while under any truth table variation then x, our variable, will have a number added to it. The numbers 1, 2, 4, and 8 will give us different numbers, as the input is High. The diagram that I have pictured there shows directly what I mean. If you have any questions regarding the code, don't hesitate to ask.
* To show the Arduino Serial Monitor just go to Tools > Serial Monitor in your Arduino IDE, as I have pictured there.
THE CODE:
/*
Design a circuit and write a test program, using the Arduino Nano,
that will test two input Transistor-Transistor Logic (TTL) gates
including AND, OR, NAND, and XOR.
By: Zoran M
*/
int gatevalue; //Declare all of the variables
int inputPin = 4;
int Output1Pin = 2;
int Output2Pin = 3;
int led = 13;
int led2 = 12;
int led3 = 11;
int led4 = 10;
int led5 = 9;
//Begin declaring what pin is what
void setup(){
Serial.begin(9600);
pinMode (Output1Pin, OUTPUT);
pinMode (Output2Pin, OUTPUT);
pinMode (inputPin, INPUT);
pinMode(led, OUTPUT);
pinMode(led2, OUTPUT);
pinMode(led3, OUTPUT);
pinMode(led4, OUTPUT);
pinMode(led5, OUTPUT);
gatevalue = 0;
}
void loop(){
gatevalue = gatevalue + (check_Gate(false, false)*8); //When an input is HIGH it will add a value to the gatevalue
gatevalue = gatevalue + (check_Gate(false, true)*4); //and each gate will have a different gatevalue.
gatevalue = gatevalue + (check_Gate(true, false)*2);
gatevalue = gatevalue + (check_Gate(true, true)*1);
switch (gatevalue){
case 1:
Serial.println("The gate is an AND gate."); //When gatevalue is 1 it is an AND gate
digitalWrite(led, LOW); //if gate is in, light is off
digitalWrite(led2, LOW); // AND gate truth table outputs are 0, 0, 0, 1 which is how the LED’s are set up
digitalWrite(led3, LOW);
digitalWrite(led4, LOW);
digitalWrite(led5, HIGH);
break;
case 6:
Serial.println("The gate is an XOR gate.");
digitalWrite(led, LOW); //if gate is in, light is off
digitalWrite(led2, LOW);//Truth table values
digitalWrite(led3, HIGH);
digitalWrite(led4, HIGH);
digitalWrite(led5, LOW);
break;
case 7:
Serial.println("The gate is an OR gate.");
digitalWrite(led, LOW); //if gate is in, light is off
digitalWrite(led2, LOW); //Truth table values
digitalWrite(led3, HIGH);
digitalWrite(led4, HIGH);
digitalWrite(led5, HIGH);
break;
case 14:
Serial.println("The gate is an NAND gate.");
digitalWrite(led, LOW); //if gate is in, light is off
digitalWrite(led2, HIGH); //Truth table values
digitalWrite(led3, HIGH);
digitalWrite(led4, HIGH);
digitalWrite(led5, LOW);
break;
default:
Serial.println("ERROR: Gate Not Present.");
digitalWrite(led, HIGH); //if gate is not in, light is on
digitalWrite(led2, LOW);
digitalWrite(led3, LOW);
digitalWrite(led4, LOW);
digitalWrite(led5, LOW);
}
gatevalue = 0;
delay(1000);
}
int check_Gate(int output1, int output2){
int x;
digitalWrite(Output1Pin, output1);
digitalWrite(Output2Pin, output2);
delay(5); // Make sure the signal has time to propogate through the gate.
x = digitalRead(inputPin);
return x;
}
Upload the code to your Arduino, open the Serial Monitor, and monitor the LEDs. Switch out the ICs and test that they all work properly. Make sure that the Serial Monitor and the LEDs are outputting the correct information. Continue onto the next step for additional pictures and the conclusion.
Step 4: Additional Pictures and Conclusion
Now, if you weren't aware by now, this circuit and code only tests one of the four gates on the ICs. One addittion/change to this project that could be made is to test all four gates on the chip simultaneously. Indicate results from each gate independently (fail/pass/type), and if one gate fails the test, or indicates a different logic function from the rest, indicate the type of IC by majority vote (i.e. if the tester finds three valid AND gates, but the fourth gate indicates something “OR”, identify the chip as an “AND” gate IC with one bad gate). There are many variations and additions that could be made to this project, and I would love to see some of yours; even if you did it just like I did.
01001000 01100001 01110110 01100101 00100000 01100110 01110101 01101110 00100001
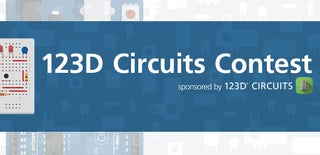
Participated in the
123D Circuits Contest
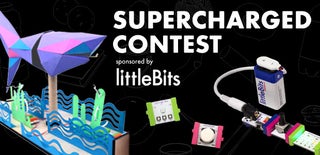
Participated in the
Supercharged Contest