Introduction: Addressable LED Neopixel Plant
People in today's society all have very busy lives; because of this we all tend to rush by our surroundings without really noticing them. I decided that I wanted to make a little piece of nature stand out and draw attention. I set up a plant with very bright addressable LEDs that would be set off by an ultrasonic proximity sensor. The plant would light up and the passerby would stop for a moment to notice it. To do this it just takes a few easy steps, the hardest part is finding decent coding.
Step 1: Decide Your Media
First off, decide where or what you want to place or attach the LEDs to. In this project I used a small but sturdy plant. Depending on how many LEDs you have you could potentially string the LEDs around even a tree. I went smaller scale here and just used a small plant, but the plant has to be able to hold the weight of the LED strip.
Step 2: Materials
After you decide your media, gather the necessary supplies. Here are the supplies I used for my project:
- 1M RGB Waterproof IP67 LED Strip (18W WS2812B 5050 Individual Addressable DC5V)
- Arduino Uno
- Hc-sr04 Ultrasonic Distance Measuring Sensor Module (Mega R3 Mega2560 Duemilanove Nano Robot)
- Wires (I used jumper wires but you can use pretty much any wires that will work)
- Breadboard (you can get any board and solder materials to it if you so choose)
- 9V Battery
- 9V Battery adapter cord for the arduino
Step 3: Find Some Code
The toughest and most time-consuming portion of this project is finding some good coding for the arduino. You will need to find code for both the LEDs and the sensor. A good coding library for this is adafruit, but I actually used another library called FastLED. Both libraries are good, and just about any library is fine if it has the right code. I will also supply my code in this instructable as well. You can easily find your own code as well if you do not want to use mine.
Step 4: My Code
Make sure to save and upload whatever code you use to the arduino first.
In case my code file at the bottom does not work here it is:
[code]
/* HC-SR04 Sensor (ultrasonic)
https://www.dealextreme.com/p/hc-sr04-ultrasonic-...
This sketch reads a HC-SR04 ultrasonic rangefinder and returns the distance to the closest object in range. To do this, it sends a pulse to the sensor to initiate a reading, then listens for a pulse to return. The length of the returning pulse is proportional to the distance of the object from the sensor.
The circuit:
* VCC connection of the sensor attached to +5V
* GND connection of the sensor attached to ground
* TRIG connection of the sensor attached to digital pin 2
* ECHO connection of the sensor attached to digital pin 4
Original code for Ping))) example was created by David A. Mellis Adapted for HC-SR04 by Tautvidas Sipavicius This example code is in the public domain. */
const int trigPin = 2;
const int echoPin = 4;
//LEDs #include "FastLED.h"
// How many leds in your strip?
#define NUM_LEDS 60
// For led chips like Neopixels, which have a data line, ground, and power, you just // need to define DATA_PIN. For led chipsets that are SPI based (four wires - data, clock, // ground, and power), like the LPD8806 define both DATA_PIN and CLOCK_PIN
#define DATA_PIN 3
#define CLOCK_PIN 13
// Define the array of leds CRGB leds[NUM_LEDS];
void setup() {
FastLED.addLeds(leds, NUM_LEDS);
// ultrasonic sensor
// initialize serial communication:
Serial.begin(9600); }
void loop() {
// ultrasonic sensor
// establish variables for duration of the ping,
// and the distance result in inches and centimeters: long duration, inches, cm;
// The sensor is triggered by a HIGH pulse of 10 or more microseconds.
// Give a short LOW pulse beforehand to ensure a clean HIGH pulse:
pinMode(trigPin, OUTPUT);
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Read the signal from the sensor: a HIGH pulse whose
// duration is the time (in microseconds) from the sending
// of the ping to the reception of its echo off of an object.
pinMode(echoPin, INPUT);
duration = pulseIn(echoPin, HIGH);
// convert the time into a distance
inches = microsecondsToInches(duration);
cm = microsecondsToCentimeters(duration);
Serial.print(inches);
Serial.print("in, ");
Serial.print(cm);
Serial.print("cm");
Serial.println();
delay(100);
//LEDs
// Turn the LED on, then pause
if (cm <= 60){
for (int i = 0; i < 60; i++){
leds[i] = CRGB::Red;
FastLED.show();
delay(50);
// Now turn the LED off, then pause
leds[i] = CRGB::Black;
FastLED.show(); delay(50);
} }
else if(cm > 60 && cm <= 200) {
// Turn the LED on, then pause
for (int i = 0; i < 60; i++){
leds[i] = CRGB::Red;
FastLED.show();
delay(100);
} }
else{
for (int i = 0; i < 60; i++){
// Now turn the LED off, then pause
leds[i] = CRGB::Black;
FastLED.show();
delay(100);
} }
}
long microsecondsToInches(long microseconds) {
// According to Parallax's datasheet for the PING))), there are
// 73.746 microseconds per inch (i.e. sound travels at 1130 feet per
// second). This gives the distance travelled by the ping, outbound
// and return, so we divide by 2 to get the distance of the obstacle.
// See: http://www.parallax.com/dl/docs/prod/acc/28015-PI...
return microseconds / 74 / 2; }
long microsecondsToCentimeters(long microseconds) {
// The speed of sound is 340 m/s or 29 microseconds per centimeter.
// The ping travels out and back, so to find the distance of the
// object we take half of the distance travelled.
return microseconds / 29 / 2; }
[/code]
Attachments
Step 5: Assembling the Materials
After you have your code more or less finished you can begin assembling the materials. In this case I will just give you the breadboard set up and from there you can continue beyond that if you want.
1. Attach the sensor to the breadboard and connect wires to each of the 4 prongs: power(VCC), ground (GND), trig, and echo. Trig was my input and Echo was my output.
2. Make sure to attach the ground wires for both the LEDs and the sensor to the ground pin on the arduino and the power wires to the 5V or 3.3V pin on the arduino. The 5V is usually the best option but if your LEDs or sensor can still draw enough power from the 3.3V without damage or lack of power then that is another option if you do not want to run the power wire on your LEDs through the breadboard. (That is what I did but there are other ways to hook up the wires)
3. Next attach the wires for the LEDs and the sensor to whichever number pin you assigned in your coding. For my LEDs I assigned the wire to pin 3 and for my sensor I assigned trig to pin 2 and echo to pin 4.
4. From there connect the ardunio to a power source. The arduino allows for two different sources of power, one is a cord that attaches to a USB port in a computer and the other is to hook up a battery with a battery adapter cord for the arduino.
Step 6: Presentation
Finally take your plant or show off your tree somewhere that has people to experience it. Display the project as you wish and enjoy!
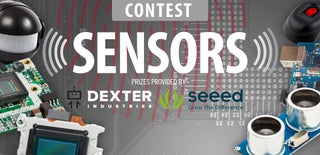
Participated in the
Sensors Contest 2016
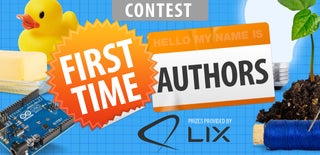
Participated in the
First Time Author Contest 2016