Introduction: Arduino Soil Moisture Monitor
This instructable lists the steps required in creating an Arduino device that measures soil moisture. This device uses a Sparkfun Soil Moisture Sensor with an Arduino Uno to measure the moisture level of soil and report a dry/wet reading through the serial monitor. When the sensor is placed into soil, it gives a reading of conductivity. If the soil is above or below a certain threshold, the serial monitor will display "Wet soil" or "Dry soil".
Step 1: Materials
For this project you will need:
- Arduino Uno
- SparkFun Soil Moisture Sensor
- Jumper wires
- USB Cable
- Arduino Software
Step 2: Putting It Together
Since the device has a relatively simple job, we're going to wire the sensor to the Arduino directly. The image was pulled from the sparkfun website; the same wiring works for the UNO.
Wire the following pins from the sensor to the board:
VCC -> Digital pin 7
GND -> GND
SIG -> A0
Step 3: Uploading the Code
Next, the code enabling the sensor to read moisture values is written and uploaded.
We're using the code provided by Sparkfun as a base, and adding another layer to our serial monitor output, which will be explained below.
Paste the following code into Arduino IDE (or download the attached .ino file) and save it:
/* Soil Mositure Basic Example
This sketch was written by SparkFun Electronics Joel Bartlett August 31, 2015Basic skecth to print out soil moisture values to the Serial Monitor
Released under the MIT License(http://opensource.org/licenses/MIT) */
int val = 0; //value for storing moisture value int soilPin = A0;//Declare a variable for the soil moisture sensor int soilPower = 7;//Variable for Soil moisture Power
//Rather than powering the sensor through the 3.3V or 5V pins, //we'll use a digital pin to power the sensor. This will //prevent corrosion of the sensor as it sits in the soil.
void setup() { Serial.begin(9600); // open serial over USB
pinMode(soilPower, OUTPUT);//Set D7 as an OUTPUT digitalWrite(soilPower, LOW);//Set to LOW so no power is flowing through the sensor }
void loop() { Serial.print("Soil Moisture = "); //get soil moisture value from the function below and print it Serial.println(readSoil()); if (readSoil()<420){ Serial.print("Dry soil"); } else { Serial.print("Wet soil"); }
//This 1 second timefrme is used so you can test the sensor and see it change in real-time. //For in-plant applications, you will want to take readings much less frequently. delay(1000);//take a reading every second } //This is a function used to get the soil moisture content int readSoil() {
digitalWrite(soilPower, HIGH);//turn D7 "On" delay(10);//wait 10 milliseconds val = analogRead(soilPin);//Read the SIG value form sensor digitalWrite(soilPower, LOW);//turn D7 "Off" return val;//send current moisture value }
Once saved, upload the code to your Arduino board and open the serial monitor.
Attachments
Step 4: Monitoring
Once the sensor is wired up, the code is uploaded, and the serial monitor is opened, you should begin seeing values followed by the message "Wet Soil" or "Dry Soil". After finding the two extremes of the sensor readings, we set the threshold at the half mark - around 420. So if the moisture level is above this, the monitor will read "Wet Soil", and if below, it will read "Dry Soil". This is done with an if else and a serial println command.
There is a lot of room for expansion, mainly in the addition of more levels of moisture rather than our basic binary dry/wet.
You can test the sensor by inserting it into both wet and dry soil as we did.
Step 5: Tidying It Up, Expansions, and Practical Uses
Once you've added any changes or modifications, you can enclose it in some sort of weatherproof box to begin use in an actual soil location.
Other expansions you may consider are the addition of a physical serial monitor display, as the Sparkfun tutorial details: https://learn.sparkfun.com/tutorials/soil-moisture...
Practical uses: this device could possibly be used in conjunction with an automated watering system, to monitor the status of a terrarium, or any scenario where a certain moisture level of a material like soil is necessary.
Thank you for taking a look at my project! This was created as part of a school assignment.
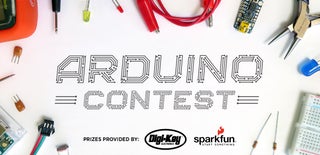
Participated in the
Arduino Contest 2017