Introduction: Calculator TI 83+/83+SE/84+/84+SE Tutorial Lesson 2: Codeflow
If you haven't visit lesson 1 so far, then do it.
If you have, then continue reading! :)
So, in this lesson I'll teach you how to do simple codeflow controls.
Please keep in mind that later on you rarely use them but it is still VERY important to know first how this is working.
So, have fun with the tutorial!
Be sure to comment, rate and subscribe!
Be also sure to check out my Website!
If you have any questions about programming be sure to check out Omnimaga!
Step 1: Simple If-conditions
:If A=5
:Then
:Disp "A IS 5"
:End
Try to guess first by just looking at the code that that program does.
For a screenie of it in action:
So if, and only if, A is equal to five it will execute that line of code, that Disp command. It executes everything between the Then and the End, no matter what you will add.
Let's say we want to add now a else part, so like "If it is sunny today I'll go swimming else I'll learn programming.".
That is also pretty straight-forward. Here's our example extended:
:If A=5
:Then
:Disp "A IS 5"
:Else
:Disp "A IS NOT 5"
:End
Now, if A is equal to 5 it will execute everything between Then and Else, so in this case it will display "A IS 5".
If A is now NOT equal to 5 it will execute everything between the Else and the End, in this case it will display "A IS NOT 5".
Do you get that? It's in fact very simple, isn't it? :)
So, it's time for a little task!
Make a program that inputs two variables, adds them and if the sum is equal to 99 it displays "IS 99" and else it displays "IS NOT 99"
So, let's start programming!
For my sollution:
Notice that in this case I used the Ans-variable to save a variable.
If you understood this, then let's move on to the next step!
Step 2: Math Operatores
"If it will not be sunny i will learn programming"
:If A≠5
:Then
:Disp "A IS NOT 5"
:End
Now, you want to have something greater than a number.
"If it is hotter than 20°C I'll go swimming."
:If A>5
:Then
:Disp "A IS MORE THAN 5"
:End
It's exactly the same with less than.
"If it is colder than 20°C I'll learn programming."
:If A<5
:Then
:Disp "A IS LESS THAN 5"
:End
Now, we want a variable to be larger or equal to a number.
"If it is hotter or as hot than 20°C I'll go swimming."
:If A≥5
:Then
:Disp "A IS ≥ 5"
:End
Again, exactly the same just with less or equal than.
"If it is colder or as cold than 20°C I'll learn programming."
:If A≤5
:Then
:Disp "A IS ≤ 5"
:End
Now, that you understood all the math operators, we can go on to the next step!
Step 3: Bool Operators
Bool operators are operators for comparison.
Now you probably say: wait a sec, didn't I just learn how to compare a variable with a number?
Well, that's true, but with bool operators you can do even more, like this:
"If it is sunny today and it is hotter than 20°C I'll go swimming."
The big difference is that here is a and in there.
:If A=5 and B=5
:Then
:Disp "A AND B ARE 5"
:End
Now let's look at or:
"If it is sunny or it is hotter than 20°C I'll go swimming"
:If A=5 or B=5
:Then
:Disp "A OR B ARE 5"
:End
Now, there is also exclusive or (xor).
:If A=5 xor B=5
:Then
:Disp "A XOR B ARE 5"
:End
Now, that you understand these operators, let's take a little look closer look at if conditions.
It executes the space between the then and the end/else if the if-condition is true.
So we have now some massive if-condition here:
So, let's take it apart.
The first innermost parenthesis is this: B>5 or B<1. Now, if B is greater than 5 the left part is true, so the hole part would be already true as it is a or condition.
Now we go outwards:
A=B and Part1
so, if Part one, the part we already looked at is true, and A=B is true, then that part is true either.
I hope you get what i mean, that the calculator just looks what is true, and it can be also very important to set parenthesis. Just take a look at these two if-conditions:
:If A=5 or (B=6 and C=7)
Now, we could just compare true stuff, there is also a command that allows us to compare false stuff, the not( command. All it does is if something is true it outputs false and if something is false it outputs true.
Hope you understood this step, so let's go on! :D
Step 4: Shortening the If-conditions
Let's get a code example:
:If A=5
:Disp "A IS 5"
:Disp "END OF PRGM"
It is very important for simple code-flow manipulation to get this difference, why will be discussed in the next step.
Step 5: Labels and Goto
So, what are labels? A label is a marked place in the program. In TI-Basic a name of a label consists out of one to two characters, e.g. "F" or "GK" or "F4". These labels can be called, so that it jumps to the label, no matter if it is in front of it or behind it. For that there is the Goto command. You set a label with the Lbl command. Here's a little example:
:Prompt X
:Goto A
Now, let's take a look at it. At the beginning is Lbl A. That line does nothing at the moment. Now, there is Prompt X. It asks for input, which we don't use at the moment :D. Then there is Goto A. This jumps to the Label A (Lbl A). Hey, wait a sec, now it asks again for X as it is the next line and then jumps up again! Yes, that's a infinity loop.
Now, please note that Labels and variables are not the same, so you can have a label called A and also the variable A.
Example:
:Lbl A
:Disp A
:A+1→A
:Goto A
After understanding Labels and Goto we can go further deep into thinking, so let's move on to the next step!
Step 6: Making Some Loops
:Prompt A
:If A=5
:Goto B
:Goto A
:Lbl B
:Prompt A
:
:
:Goto A
:Goto B
It's important to get this, so here's a little programming task!
Make a program that asks the user for two variables in an infinity loop, if they are both same and greater than 5 it will exit the infinity loop.
For possible sollution: Your solution may look somewhat different, but!
IMPORTANT: When jumping away with an if-condition we had, never EVER use a Then and a End, as that will cause after some time memory errors.
That is because you jump out of the if-condition and so the calculator will be keep searching for the end that isn't there, taking up more and more memory. If you have to do some stuff before you jump then do it like this:
:Then
:<stuff>
:End
:If <condition>
:Goto A
Step 7: The Menu( Command
It makes TI-like menus, so you have a top caption and up to 7 options, that menu doesn't support scrolling or tabs.
To use it you first define your header, then you define each option, you just use as many as you want. After the text of each option you put in the label name where it will goto if you click the menu point, so here's an example!
:Menu("WELCOME","OPTION 1",1,"OPTION 2",2,"OPTION 3",3,"EXIT",EX)
:Lbl 1
:Disp "OPTION 1"
:Pause
:Goto ST
:Lbl 2
:Disp "OPTION 2"
:Pause
:Goto ST
:Lbl 3
:Disp "OPTION 3"
:Pause
:Goto ST
:Lbl EX
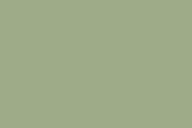
You could also optional add more menu points, as you see, after the menu points is always the label where it shall jump to when selected.
Let's go on to the next step!
Step 8: A Programming Task
Make a program that can check if a number is greater than 5 and smaller than 100, it can check if a number is even (fPart() is to get the fraction part, iPart() is to get the integer part. Well, that's it! Let's start programming!

For my solution:
Step 9: The End
Whew, you finally made it!
You worked yourself through another TI-Basic tutorial.
So, here is another contest for you!
Make a program that can calculate radian into degree and vice versa.
Email me your program or your code within one week.
So, be sure to comment, rate and SUBSCRIBE!
Be also sure to check out my Website!