Introduction: Get Started With Raspberry Pi GUI
So you have a Raspberry Pi and a cool idea, but how do you make it as easy as a smartphone for your user to interact with?
Building a Graphical User Interface (GUI) is actually quite easy, and with some patience you can produce amazing projects.
Step 1: The Broad Overview
One of the most powerful tools that Raspberry Pi provides over other micros, is the rapid rate and ease you can create a Graphical User Interface (GUI) for your project.
One way to achieve this , specialty if you have a full touchscreen (or a standard screen and input device such as a mouse),it became amazing!
For the purpose of this article, we’ll be using Python 3 with Tkinter :
A powerful library for developing graphic user interface (GUI) applications, on the Raspberry Pi where makers are concerned.
Tkinter is probably the most commonly used with Python, and plenty of resources exist on the internet.
Step 2: "Hello World" in Tkinter
We’re using aRaspberry Pi loaded with Raspbian Stretch OS.
To run our Tkinter GUI Applications. we can also use any other operating system that have python installed.
Raspbian comes with both Python 2, Python 3 and the Tkinter library installed.
To check which version you have installed, from the terminal run:
python3 --version
Create a new file called app.py and enter the base code shown below :
#!/usr/bin/python from tkinter import * # imports the Tkinter lib root = Tk() # create the root object root.wm_title("Hello World") # sets title of the window root.mainloop() # starts the GUI loop
If you’re not using an IDE, run the following command in a terminal from the directory containing your Python code to run the program.
python3 app.py
Step 3: Customising the Window
Let’s now look at how to customise this window.
Background colour
root.configure(bg="black") # change the background colour to "black"
or
root.configure(bg="#F9273E") # use the hex colour code
Window dimensions
root.geometry("800x480") # specify the window dimension
or
root.attributes("-fullscreen", True) # set to fullscreen
- Keep in mind that you will get stuck in full-screen mode if you don’t create a way to exit.
# we can exit when we press the escape key def end_fullscreen(event): root.attributes("-fullscreen", False) root.bind("<Escape>", end_fullscreen)
Step 4: Widgets in Tkinter
Tkinter includes many different widgets to help you create the most appropriate user interface. Widgets you can use include:
• text box
• buttons
• check button
• slider
• list box
• radio button
•etc..
Now we can add some widgets such as text, buttons and inputs.
Adding Widgets
- Labels
label_1 = Label(root, text="Hello, World!")
Before it is visible in the window though, we need to set its position. We will use grid positioning.
label_1.grid(row=0, column=0) # set the position
Entry Input
label_1 = Label(root, text="Hello, World!", font="Verdana 26 bold, fg="#000", bg="#99B898") label_2 = Label(root, text="What is your name?", height=3, fg="#000", bg="#99B898") entry_1 = Entry(root) #input entry label_1.grid(row=0, column=0) label_2.grid(row=1, column=0) entry_1.grid(row=1, column=1)
Buttons
#Add a button inside the window Button = Button(root, text="Submit") Button.grid(row=2, column=1)
Step 5: Adding Logic
Now we have a simple form, however clicking on the button does not do anything!!
We will explore how to setup an event on the buttons widget and bind it to a function which executes when clicked.
For this purpose we will update label_1 to display "Hello + the text entered in the input". When you select the submit button.
Download the code below then run it.
Step 6: LED Control
So far we see how to add button to the window and add logic to it in order to perform action.
Now, we will change the code a little bit. So we are going to create a form and add two buttons to it. One to turn the LED on/off, and the other to exit the program.
Note : Make sure you have updated your Raspberry before starting, and that you have the GPIO library install, Open the command window and enter the following
the GPIO library install. Open the command window and enter the following:
$ sudo apt-get update
$ sudo apt-get install python-rpi.gpio python3-rpi.gpio
The Build:
Parts Required:
1 x Raspberry Pi 3
1 x LED
1 x 330Ω Resistor
Building The Circuit:
Follow the photos above.
Pay attention to the LED orientation and the pin where connected (GPIO23).
Step 7: Adding Servo Motor Controller
We will moving to something other than a button we can also use various inputs to control the PWM (Pulse Width Modulation) outputs from the Raspberry Pi.
A servo motor is a great choice it translates a PWM signal into an angle.
The build:
Parts Required:
1 x Raspberry Pi 3
1 x LED
1 x 330Ω Resistor
1 x Servo Motor
Building The Circuit:
Follow the diagram shown above ( LED connected to GPIO 23, Servo Motor connected to GPIO 18).
Check the video if you stuck.
Attachments
Step 8: Conclusion
There you have it! Go forth and conquer some amazing UI ideas!
If you have any question of course you can leave a comment.
To see more about my works please visit my channel
Thanks for reading this instructable ^^ and have a nice day. See ya. Ahmed Nouira.
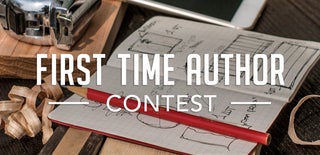
Participated in the
First Time Author