Introduction: Hide Text in Image File : Image Encryption in Matlab
"Are you watching closely?"
Well most people don't and that brings us to this simple instructable. What's better than hiding your secret information like account info, passwords etc in an image that can't be deciphered without a key, which again, is an image!
For this you'll need....
- Matlab
- Some basic knowledge on pixels of images.
- Some basic knowledge of ASCII values of characters.
Let's get going!
Step 1: Understanding Images and Text: Basics
**Skip if you're familiar with pixel values and ASCII
Each pixel in a RGB image is represented by three 8-bit unsigned(non-negative) integers for the red, green and blue intesities. 8 bit means the intensities range from 0 to 255. In a grayscale image, each pixel is given by single integer value. 0 means total black, 255 means total white. The in-betweens are shades.
Each character of text is represented by ASCII values that run from 0 to 128. The extended ASCII is 8-bit and matches with the pixels' intensity range, which is 0 to 255.
So encryption using these two can be done very simply just by treating them as normal integers and doing any operation which limits the encrypted value within 0 to 255, the encrypted result can also be represented as a pixel or as a character.
Here you see the ASCII table for reference.
Step 2: Select a Proper Grayscale PNG Image
Yeah, start with a grayscale. Once you understand that, you can proceed with RGB. You can select BMP format also but it is advisable to avoid JPEG if you are not using Matlab since it is compressed using compressing techniques.
You can select a RGB image and convert it to grayscale using photoshop (open image > select Image > select Mode > select grayscale). Sometimes an image seeming to be grayscale is actually RGB. So do check beforehand.
It's better to select a noisy image since after encrypting, the encrypted part will seem totally noisy. So an image which is already noisy will serve as a better camouflage. I chose this noisy satellite image of an area.
Keep it in mind that this original image will be your password to decrypting the coded image. Keep it safe.
(The attached code will work on an image named 'original.png'. Change the code on your wish.)
Step 3: Select Your Text File
Whatever top secret information you are hiding, put them into a file. Open notepad if you have Windows and save it there. It will be just fine. Just make sure the number of characters in the file is not more than the number of pixels in the image. The maximum number of characters that you can use is (width x height) of your image in pixels.
(The attached code works on a file named 'myfile.txt' so if you use the code, just rename the file)
Step 4: Encrypt the Data Onto the Image
You can use various methods of encryption between the data and image pixels. Here a most basic algorithm is followed. You can easily check and modify the matlab codes attached.
Put all the files (matlab file encrypto.m, text file and original image file) in the same folder and run the encrypto.m script. If matlab asks to change default folder then do so.
When the script is run, an image will be created. If you look closely on the image above you'll see the pixels at the top in which the text is hidden (but one can't decipher it without the original image).
P.S. You can use bitwise XOR for encryption and decryption although I didn't use it here. Also be careful of proper conversion of the variables between 16-bit unsigned integer and 8-bit unsigned integer.
P.P.S. : go here and search for the commands and functions used in the codes if and when you need help.
Attachments
Step 5: Pseudocode
If you want to make your own program, this may help. Here I'm showing the simple bitwise XOR approach.
Encrypter:
1. Open the text and image file.
2. loop while( character count <= total characters)
i. character=convert to 16 bit integer(character) //by default the pixels and characters will be 8-bit in Matlab. This caused me a lot of problems
ii. pixel=convert to 16 bit integer(pixel)
iii. encrypted_pixel= (pixel) bitwise_xor (character)
iv. pixel=next pixel
v. character=next character.
vi. character count= character count + 1
3. end loop
4. rest of encrypted_pixels = pixels of original image
Decrypter:
1. Open the original image and encrypted image.
2. loop while( pixel count <= total pixel)
i. original pixel=convert to 16 bit integer(original pixel)
ii. encrypted_pixel=convert to 16 bit integer(encrypted_pixel)
iii. a= (original pixel) bitwise_xor (encrypted_pixel)
iv. if a=0 then break else decrypted_text=a
v. original pixel=next original pixel
vi. encrypted_pixel=next encrypted_pixel.
3. end loop
Step 6: Now It's Time to Decrypt!
There is no meaning of any lock if there isn't any key. Luckily we have. Here the original image is the key and is used with the decrypting code( attached decrypto.m ). Use the reverse algorithm that you used in the encrypter. You'll get a decrypted.txt file.
**For the next trick how about encrypting an image within another image?
That's it! The end is here! Try with different files and don't forget....
"The secret impresses no one. The trick you use it for is everything."
Attachments
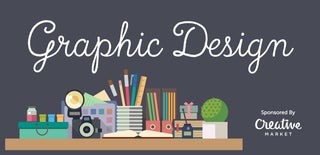
Participated in the
Graphic Design Contest
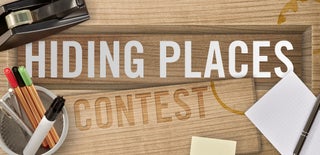
Participated in the
Hiding Places Contest