Introduction: Home Hydroponics Using Quickbird
Quickbird is a automated monitoring and control system powered by Intel Edison. It's built for industrial use and is being used for forest management, hydroponics farming, environmental monitoring and many other data-intensive line-of-business IoT applications. You can learn more about us at quickbird.uk.
For this project, we built a small hydroponics system to demonstrate Quickbird's utility in hydroponics farming.
This tutorial has 5 parts:
- Introduction to hydroponics
- Building the plant bed
- Programming the Edison
- Programming sensors
- Demo
Step 1: What Is Hydroponics?
Hydroponics is a hydroculture technique for growing plants in a water and nutrient solution, without soil. Plants don’t use soil, they use the food and water present in the soil. Soil’s function is to provide nutrients and anchor plant’s roots. In a hydroponic system, you use a complete nutrient solution and an inert growing medium to support the roots. This helps growers avoid many plant diseases caused by soil-borne pathogens. A hydroponics system can produce up to 6-8 times more yield than conventional farming for a given area.
Bypassing soil does have its downsides too. Irrespective of the season, the ground temperature remains constant at around 20-25° C below the surface, protecting the roots from extreme weather conditions. The mineral content in soil also remains largely unchanged over the course of a season, acting as an uninterrupted source of nutrients.
Unfortunately, soil’s stabilizing properties are not available in a hydroponics system. Hence, it’s important to ensure that environmental conditions stay within an optimal zone, as a meagre 4-5% deviation in water acidity or root zone temperature in the absence of natural “auto-correct” can wreak havoc on the crop. Historically farmers have used a myriad of meters - acidity, water temperature, carbon dioxide and many more - scattered around their greenhouses to check and adjust systems manually. The siloed nature of these meters leave a lot to be desired. For instance, often when nutrient solution starts getting diluted (pH meter) you’d want the system to automatically turn the feed pump on to mix in more nutrients (nutrient pump) all the while telling water temperature meter to not fire any alerts for a while (mixing is an exothermic reaction, water temp. usually stays above the optimal values for a few minutes after mixing).
For this demo, we'll be building an ebb & flow hydroponics system. Quickbird will managing the following:
- Nutrient solution
- Electrical conductivity
- Acidity
- Temperature
- Level of solution in the tank
- Ambience
- Light intensity
- Relative humidity
- Temperature
- Carbon dioxide levels
- Water Flow Meter
- Water Pump
- Plant bed moisture
Step 2: Building the Plant Bed
For the planter, we bought a 40 x 30 x 24 cm wooden box from Amazon. You can (and should) make one yourself if you have access to a wood workshop.
Making the box leak proof
Need:
- an adhesive sealant (this is what we got, don't get 300ml it's a single use item)
- for unpainted wood, polyurethane varnish (this is not too bad)
Most off-the-shelf wooden boxes aren't designed to hold water in, since ours is going to see a quite a bit of it we need to make sure the joints are sealed properly. We applied the polyurethane adhesive sealant generously (and rather hastily) to all the joints inside the box. It takes about an 20 minutes to dry to touch and about an hour to cure.
Apply two thin coats of varnish to protect the sides. Each coat takes about an hour to dry completely.
Plant the seeds
Hydroponic media is an inert substance used to anchor plant's roots. We used a 60/60 mixture of coco rusk and clay beads called hydrococo. Plant some seeds (we used coriander, grows really fast) and turn on the hydroponics system.
Step 3: Programming the Edison
Our sense & control box interfaces with sensors through an Arduino which is controlled by the Edison.
We’re using two node modules:
- serialport – to communicate to the Arduino and read Serial commands, and
- socket.io-client – to establish a connection to the Quickbird IoT Visualiser where we can send our data to be visualised in real-time.
Here are the step by step instructions on how to get your data to the Quickbird IoT Visualiser:
- Open a browser and navigate to Quidkbird Signup.
- Create an account or sign-up with Facebook or Google.
- Once you’ve done that, you will get redirected to you Profile page.
- Copy your API Token and store it as you will need it later on to send your data to the visualiser.
- When you're done click on the “Go to IoT Visualiser” button. This is what you will see. At the moment this is just some dummy data and your visualiser is waiting for you to send it some data… and this is what we’ll do next.
- Get the app.js and package.json files from this page and upload them to your Edison. For this you can use free FTP software such as FileZilla.
- Open FileZilla and enter the IP address of your Edison in the Host field. Then enter root in the Username field and your Edison root password in the Password field. Enter 22 in the Port field.
- Click Quickconnect, drag & drop app.js and package.json from your machine onto the Edison.
- Change the myAPIToken value in app.js with the one you copied in step 4. This is how the IoT Visualiser knows that you are allowed to update data.
- Open a Terminal (cmd, on windows) and SSH to your Edison or connect to it via a USB cable plugged into the console port of your Edison dev board. For more info see: SSH-ing into Edison and Intel | Setting up Serial Terminal.
- Once you're in, navigate to the folder where you’ve copied app.js and package.json and run npm install to install the app dependencies. Then run node app to start the program.
- Go to IoT Visualiser and you should see live data coming through from the Edison. If you don't see anything, refresh the page.
Attachments
Step 4: Programming Sensors
Why Arduino?
Before we dive into the communication between Arduino and Edison, let us consider, why use an Arduino at all?
Although the first incarnation of this system is pretty basic, we intend our system to be used in serious applications which need to be reliable. To reduce the amount of headache, it’s good to separate concerns – the MCU code is the one that needs to be very carefully written and reliable. You might spend a lot of time (and money, if you are a business) for each line of code on the Arduino. The application code does not need to meet the same standard, and we can improve it and add features much quicker. Now, you might say hang on, Edison includes an MCU!
It does, but unfortunately the MCU SDK comes with a serious list of limitations. https://software.intel.com/en-us/node/545142 The most problematic ones are:
- No watchdog time– that’s the ‘processor death-watch’, that re-boots the MCU should it get stuck for any reason. For us this is literally half the reason of putting control application on an MCU
- The MCU does not start executing immediately after Edison starts, instead the will be a delay of 20-25 seconds until Linux kernel boots up, before MCU inside Edison will start running code. MCU can start executing code within milliseconds of starting, which is great, and makes restarts irrelevant.
- Lack of floating pint support makes writing code a pain.
- Lack of threading, and poor interrupt performance
- As a bonus Arduino comes with a lot of code for working with various sensors.
As an extra, note that when you use the Arduino SDK to program Edison, that code does not run on the MCU within Edison – it executes as a normal Linux process, subject to all the issues listed above.
That being said, Edison is great as an embedded system- low power, high performance, and with full GPIO control.
Communication
Arduino communicates with Intel Edison through the serial port. Using the large Arduino adapter board for Edison is probably the simplest way to connect them.
You can use the full size serial A port on Edison and Serial-B port on Arduino to connect the two with a normal USB cable. In that case, you only need to power the Edison, as it will supply up to 1A of 5V power to the Arduino – way more than most Arduino boards can source. In turn, the Arduino can’t power the Edison.
We prefer to use the hardware serial port on the Edison. There are two of them, and we use the first one. By default, our app sets all ports to a baud rate of 115200 bits/sec. For that we use this small adapter board from Dfrobot. It’s cheap, compact and includes the level-shifters from Edison’s annoying-but-power saving 1.8V to Arduino’s obsolete-but-trusty 5V. Unlike the large board, the Dfrobot one does not have any configurable logic or multiplexers that you have to deal with. We make a straight connection between power, ground, TX and RX pins on that board and the Arduino.
Data Format
The Edison application will accept data in the following format:
{ "data":[0, 40, 19, 20], "labels":["time","Humidity","Air Temp","Water Temp”], "units":["time","%","Deg C"," Deg C "], "upperLimits":[0,70,50,30], "lowerLimits":[0, 30, 0, 0] }
Note that it includes time. If you have an RTC connected to the Arduino, you can insert the timestamp when the reading was taken. If you don’t, put a value of zero and our application will timestamp it with the time at which it received the message.
Example Code
To help you get started, we put together two Arduino sketches. The first one will cause Arduino to spam random values through the serial port – use it to very that you connected everything correctly.
https://gist.github.com/natan-morar/39c5fb661d9aba41d4e2aaf43ec172b6
We recommend you use ArduinoJson – an excellent library that will write perfect and compact JSON for you. It might take a bit of effort to learn, but is absolutely worth it as you move on to more complex projects, and is way better that pushing around literals.
The second one read the two most common sensors in the maker universe – the DS18B20 temperature probe and DHT22 humidity sensors. If you are halfway serious Arduino enthusiast, you will have them somewhere in your drawer.
https://gist.github.com/natan-morar/8cd54bd971dd65cd11ba5273ca48385d
You may notice our article features a lot of DFrobot kit- they do not sponsor us (They should!), we just like their kit.
Step 5: Demo
You may notice our system has a lot more sensors, some of them are more expensive than the DHT22 everyone uses. Whether you want to use them depends on your application – for us it’s worth it:
- We use a waterproof SHT10 humidity and temperature probe in a metal casing – it’s more accurate and is protected from environment.
- We added a CO2 sensor – many greenhouses add CO2 to boost plant growth.
- We use a PH and EC sensors to monitor the solution used to supply plants with nutrients – see (https://blog.quickbird.uk/ec-and-ph-sensors-for-arduino-98bb70c756b9)
- A garden variety light sensor
- An submersible water pump and a relay to control it. Beware with relays – they have a tendency to add noise to your circuit and cause you analog sensors to go haywire.
- Water flow meter to know how much we are watering
- Soil capacitance sensor, for measuring moisture content
- A float switch to making sure that we still have water left in the tank
- A fan to pull in the air for the CO2 meter
- A button! A glowing button is a great finishing touch for your project! < https://www.dfrobot.com/index.php?route=product/p...>
Check out the video to find out about the cool physical setup we’ve made, and how we discovered that audio jacks are a good way of connecting up sensors. Beware of 4-pin jacks, they are an absolute pain to solder!
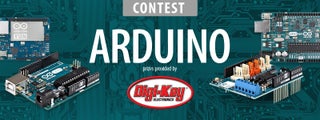
Participated in the
Arduino Contest 2016
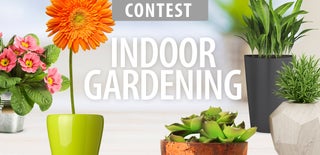
Participated in the
Indoor Gardening Contest 2016