Introduction: Intel IOT Doorbell
Intel IOT doorbell is basically a smart doorbell that enhances the level of security. Whenever a visitor rings the doorbell, a Wifi Call is placed to all the family members smartphone notifying that someone is on the door. If they wish to accept the call, a live video feed will be launched from the webcam placed outside the house. There is no location restriction so even if the member is outdoors and has an internet connection, he/she can receive the call to check who is at the doorstep. Along with the one way video streaming this doorbell also notifies if there is any unusual activity by sensing motion.
You can add your own ideas to this project like VoIP call facility too.
Step 1: Hardware/Software Required
The main board that we used was Intel Edison board. You can choose the Raspberry Pi or any other board that you are comfortable working with.
Hardware Required
- Intel Edison Board with Arduino Expansion Board
- Push button (Doorbell)
- PIR motion sensor
- Android Mobile
- Webcam/Old Android Phone
Software Required
- Android studio/Android Eclipse
- Putty(For taking terminal access to Edison Board)
Step 2: Firing Up Intel Edison Board
I am not going to cover up full flashing of Linux image on Intel Edison Board. For that, you can refer tutorials given on their official website or just youtube it.
Before starting, I assume you have the OS running on your board. Now to take the terminal access we need to download Putty i.e. an SSH client. Click HERE to Download
Just plug in your Edison board to your laptop/PC and from launch putty. Select Serial as the communication type and enter port number as well as baud rate which is 115200 for Intel Edison. For finding out which port number Edison board is connected to, just go to Device manager -> COM & LPT section -> USB Serial Port COM X(where X is a number). In my case it is COM 7. [REFER IMAGE]
Once you gain the terminal access, we need to write some scripts to:
- Detect when the doorbell is pressed
- Detect motion from the motion sensor
- Send data to Android server
Apart from that, our board will also be a Webcam Video streaming server.
Step 3: Interfacing Button With Intel Edison Board
The first step is to interface a normal push button with Intel Edison Board. I am using the button from the Groove Starter Kit Plus. It has 4 connections that are VCC, GND, NC(no connection), and SIG (signal).
I will be using Python to write scripts, you can use bash or any other.
This is a sample python script to detect if a button is pressed.
<p>import mraa<br>import time</p><p>button=mraa.Gpio(4)</p><p>while 1:</p><p> if button.read()==1: print "Button Pressed" time.sleep(0.2)</p>
Copy this code and save it in a file called button.py
Execute using "python button.py" (without quotes)
Here the button is connected to Digital Pin 4 of the Arduino expansion shield.
Next moving on to detecting motion with the PIR motion sensor.
Step 4: Interfacing PIR Motion Sensor With Intel Edison
Next, we will need a motion sensor for that we choose the PIR motion sensor. It has a good coverage range to detect any unusual activity in front of the doorstep.
Connections
PIR Sensor <--> Arduino Expansion Board
5V <--> 5V
GND <--> GND
Signal <--> Any Digital Pin (E.g. -> 8)
Note that whenever the PIR sensor senses some motion, it will give HIGH on the signal pin for few seconds. To read the signal PIN in python, it is very simple.
import mraa pir=mraa.GPIO(8) while 1: if pir.read()==1: print "Motion Detected"
So this script will print motion detected whenever PIR detects some motion. We will notify the user with a notification whenever a motion is detected. When the user taps on the notification the Live Feed of the webcam will be launched to check that status outdoors.
Step 5: Webcam Server
As Intel Edison has its own version of Linux OS running on it, we can install various third party apps to make a Webcam Video Stream server.
For that first you need to configure Wifi in Intel Edison.
Just type "configure_edison --setup" without quotes and follow the instructions. You need to select the SSID after the scan is completed and enter the password at last to connect.
So basically Intel Edison and the Android device would be on the same network. If you want to access the video stream from outside your house, then you will need a static IP in your home router.
You can refer to this instructable for running Webcam Streaming Server on Intel Edison. Note that only few webcams are supported by Intel Edison. There is a link mentioned in the following instructable to check supported webcams.
https://www.instructables.com/id/Intel-Edison-IP-We...
It is a bit confusing process, so do it step by step. After the server is started you will get a URL where the video will be streaming.
If you plan to use an old Android phone, then you can download a 3rd party app called IP WEBCAM which will make your task whole lot easier. Just download install and then start the server. You will have to first connect the phone to Home Wifi router.
Step 6: Socket Connection for Intel Edison (Client)
Now we need to exchange data between Intel Edison Board and the Android app. For that we will use the normal socket connection. You can use other methods too according to your needs.
There is a sample program of socket in python:
import socket <p>def send(data):<br> sock = socket.socket(socket.AF_INET,socket.SOCK_STREAM) try: sock.connect(("192.168.43.233", 8080)) sock.sendall(data + "\n") except socket_error as serr: print "" sock.close() print "Sent: {}".format(data) print "button done" sock= socket.socket(socket.AF_INET,socket.SOCK_STREAM) try: sock.connect(("192.168.43.181",8080)) sock.sendall(data + "\n") except socket_error as serr: print "" sock.close() print "Sent: {}".format(data) print "" return</p>
This is a function to send data to android app. First thing to note is the sock.connect line where the IP and the port number is mentioned. Now this IP is of the Android mobile which is acting as the server in this case.
So to use this function just write "send(data)" where data is the string you want to send. According to the data sent, the android mobile will know whether someone rang the bell or a motion is detected.
Step 7: The Android App
Again I can't teach how to make the whole Android App right from the start. So I will assume that you have the basic knowledge of Android App making.
I can tell you the basic idea of the connections happening and the Caller screen.
In the Android app, there will be a button to view the LIVE video streaming from the webcam. Apart from that, you can add an option to mute the notification or to turn off the calling just like "Do Not Disturb".
I have mentioned various modules with the sample code that will help you build the app. In the end, I have also included the complete Android project files just for your reference.
Socket CONNECTION
We will be using the basic socket connection to send messages across the devices. Here our smartphone will act as a server.
<p>Socket socket = null;<br> DataInputStream dataInputStream = null; DataOutputStream dataOutputStream = null;</p><p> try { serverSocket = new ServerSocket(SocketServerPORT); while (true) { socket = serverSocket.accept(); dataInputStream = new DataInputStream( socket.getInputStream()); </p><p> String messageFromClient = ""; //If no message sent from client, this code will block the program // messageFromClient = dataInputStream.readUTF(); BufferedReader in = new BufferedReader(new InputStreamReader(dataInputStream)); messageFromClient = in.readLine(); /*message += "#" + count + " from " + socket.getInetAddress() + ":" + socket.getPort() + "\n" + "Msg from client: " + messageFromClient + "\n";*/ message = messageFromClient; if(message.equals("ring")){ Intent dialogIntent = new Intent(Server.this, Call.class); dialogIntent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK); startActivity(dialogIntent); }</p>
So in the above code message is the string received from the Intel Edison Board. So if it receives "ring" then a new activity is started, which will bring up the calling screen with the options to accept and end a call.
Calling Screen on top of the Lock screen
We need to bring up the calling screen even when the phone is locked. To do that include the following flags in the Android Activity.
<p>getWindow().addFlags(WindowManager.LayoutParams.FLAG_SHOW_WHEN_LOCKED);<br>getWindow().addFlags(WindowManager.LayoutParams.FLAG_DISMISS_KEYGUARD); <br>getWindow().addFlags(WindowManager.LayoutParams.FLAG_KEEP_SCREEN_ON);<br>getWindow().addFlags(WindowManager.LayoutParams.FLAG_TURN_SCREEN_ON);</p>
Play Ringtone
To play the ringtone we will use MediaPlayer class.
MediaPlayer mediaPlayer; <p>mediaPlayer = MediaPlayer.create(getApplicationContext(),R.raw.a); // a is the media file</p><p>mediaPlayer.start(); //start playing the ringtone</p>mediaPlayer.stop(); //stop playing the ringtone
Live Video Stream
We will be using the web view to view the content from the URL that we got in the previous step. Here is the basic code:
<p>public class LiveCall extends Activity { WebView web;<br> @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); requestWindowFeature(Window.FEATURE_NO_TITLE); getWindow().addFlags(WindowManager.LayoutParams.FLAG_SHOW_WHEN_LOCKED); getWindow().addFlags(WindowManager.LayoutParams.FLAG_DISMISS_KEYGUARD); getWindow().addFlags(WindowManager.LayoutParams.FLAG_KEEP_SCREEN_ON); getWindow().addFlags(WindowManager.LayoutParams.FLAG_TURN_SCREEN_ON); this.getWindow().setFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN,WindowManager.LayoutParams.FLAG_FULLSCREEN); setContentView(R.layout.activity_live_call); web = (WebView) findViewById(R.id.web); web.setWebViewClient(new myWebClient()); web.getSettings().setJavaScriptEnabled(true); web.loadUrl("http://192.168.43.142:8080/flash.html"); web.setBackgroundColor(0); }</p>
The URL IP i.e. 192.168.43.142 will be basically your Intel Edison Local IP and 8080 is the port number. Enter the complete URL that you got in the previous step.
Motion Detection Notification
When there is motion detected at the doorstep, a message is sent from the Intel Edison board to Android app notifying this activity. Here is the code for notification along with an option to view the live view of the video stream.
<p>if(message.equals("motion")){<br> String strtitle = getString(R.string.app_name); // Set Notification Text String strtext = "Some one is on door";</p><p> // Open NotificationView Class on Notification Click Intent intent = new Intent(Server.this, LiveCall.class); // Send data to NotificationView Class intent.putExtra("title", strtitle); intent.putExtra("text", strtext); PendingIntent pIntent = PendingIntent.getActivity(Server.this, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT); Vibrator v = (Vibrator)getSystemService(VIBRATOR_SERVICE); // Vibrate for 1 seconds v.vibrate(2000); NotificationCompat.Builder builder = new NotificationCompat.Builder(Server.this) // Set Icon .setSmallIcon(R.drawable.ic_launcher) // Set Ticker Message .setTicker("Door Bell") // Set Title .setContentTitle("Intel Iot Door Bell") // Set Text .setContentText("Someone is at the Door!") // Add an Action Button below Notification .addAction(R.drawable.live, "GO LIVE", pIntent) // Set PendingIntent into Notification .setContentIntent(pIntent) // Dismiss Notification .setAutoCancel(true);</p><p> // Create Notification Manager NotificationManager notificationmanager = (NotificationManager) getSystemService(NOTIFICATION_SERVICE); // Build Notification with Notification Manager notificationmanager.notify(0, builder.build()); } }</p>
Here the message string received is "motion". After that we create a notification displaying message 'Someone is at the Door!' along with an option to GO LIVE and watch the video stream.
So almost all the important aspects of the Android app are covered in this step. Now the last thing that remain is adding up all the code to make it look like a system as whole.
Attachments
Step 8: Merging Everything
This final step includes merging all the steps codes which we wrote previously. You can design the layout of the Android App as per your requirements.
Coming back to Intel Edison board, we have two conditions now. The first is when someone presses the doorbell, then we will send a string "Ring" and if motion is detected we will send "Motion"
This was the simple logic running on the Intel Edison Board. Once the string is received by the Android app it will act accordingly by either displaying the calling screen or notifying the user about the motion.
If the user chooses to accept the call then a live video feed from the webcam is launched using the WebView in Android app. The video streaming server is running on the Intel Edison Board.
So that is it for the project INTEL IOT doorbell. We made this all in one night at the Intel IOT hackathon/roadshow 2016. You can also add two VoIP(sound) calling feature so that the member can communicate with the visitor.
This project is open to a whole lot of improvements and new ideas. You can add your version of things to make it much better. I have included a small DEMO video showing all this in action.
The main script file CLIENT.PY is also included. Refer that and make your own changes. Note that few lines may differ in comparison to what I have explained here. It is just for your reference.
Do share the project with your friends and post the doubts in the comment section below. You can connect with me on Facebook to discuss things in detail- http://facebook.com/karan.makharia
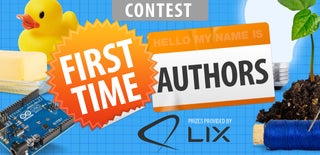
Participated in the
First Time Author Contest 2016
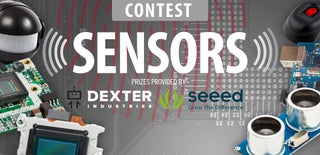
Participated in the
Sensors Contest 2016
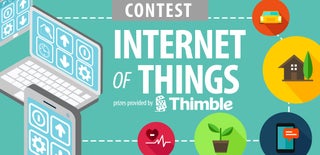
Participated in the
Internet of Things Contest 2016