Introduction: LED Morse Code Encoder
Morse code is a old method of communication wherein text information is transmitted as a series of on-off tones, lights, or clicks which represent specific characters.
In this project, we will create a program and device, that takes text as an input serially, then relays this message to the Arduino, where the LED will light up to the morse code equivalent of the text message.
Step 1: Tools and Materials
- Arduino 101 or Arduino Uno
- Red LED
- 100Ω resistor
- Jumper Wires
Step 2: Circuitry
The circuitry is very simple, since all that is needed is to control one LED.
- Connect the negative pin of the LED (flat edge) to ground.
- Connect the positive pin of the LED (rounded side) to a 100Ω resistor.
- Connect the 100Ω resistor in series to pin 9 on the Arduino.
Step 3: Code
The code is rather complex, but certainly is rewarding to see it work!
<p>// declare the pin wehere the LED is plugged in<br>int ledPin = 9; // assign a pointer for the letters represented in morse code char* letters[] = { ".-", "-...", "-.-.", "-..", ".", "..-.", "--.", "....", "..", ".---", "-.-", ".-..", "--", "-.", "---", ".--.", "--.-", ".-.", "...", "-", "..-", "...-", ".--", "-..-", "-.--", "--.." }; // assign a pointer for the numbers represented in morsecode char* numbers[] = {"-----", ".----", "..---", "...--", "....-", ".....", "-....", "--...", "---..", "----."}; // declare how long the delay is in between sequences of dots int dotDelay = 200;</p><p>void setup() { // setup the ledPin to be an output pinMode(ledPin, OUTPUT); // alows us to use serial to communicate with the Arduino Serial.begin(9600); }</p><p>void loop() { char ch; if (Serial.available()) {// is there anything to be read from USB? ch = Serial.read(); // read a single letter through the serial monitor console // if the character is between a to z i.e. it's a small letter if (ch >= 'a' && ch <= 'z'){ flashSequence(letters[ch - 'a']); // is it a capital letter? } else if (ch >= 'A' && ch <= 'Z') { flashSequence(letters[ch - 'A']); // is it a number? } else if (ch >= '0' && ch <= '9') { flashSequence(numbers[ch - '0']); // if it's none of the above, make it a space which is 4 times the delay of a dot sequence } else if (ch == ' ') { delay(dotDelay * 4); }// gap between words } } // determines the sequences to flashed by parsing the seqeunce of letters read by the serial monitor void flashSequence(char* sequence) { int i = 0; // while there are still characters keep going through each one while (sequence[i] != NULL) { flashDotOrDash(sequence[i]); i++; } // increment by one until end of characters delay(dotDelay * 3); // gap between letters } </p><p>// checks if it is a dot or a dash void flashDotOrDash(char dotOrDash) { digitalWrite(ledPin, HIGH); if (dotOrDash == '.') { delay(dotDelay); } else { // must be a - delay(dotDelay * 3); // if it's a dot then we need a longer delay gap relative to a dot. } digitalWrite(ledPin, LOW); delay(dotDelay); // gap between flashes }</p>
Step 4: Demo
To test the program, text without special characters is inputed to the serial monitor console, in this case, the classic programmers "Hello World", and this is lit up in morse code on the LED, after enter is triggered
Step 5: Riddle Me This
I have decided to encode a message via Morse Code. Comment down below if you figure out what the secret message is!
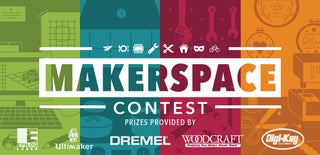
Participated in the
Makerspace Contest 2017