Introduction: Let's Make BMP180-LCD (Arduino)
¡Hola!
En este Intructable verás como proyectar los factores de temperatura que brinda el BMP180 en tu pantalla LCD, con el Arduino UNO.
Para hacer esto necesitarás:
-Arduino UNO.
-Cables
-BMP180.
-Protoboard.
Primero, debes hacer el montaje.(En la barra de arriba lo puedes ver) El montaje es el montaje del LCD junto con el de el BMP180.
Ahora debes descargar una librería para hacer que el sensor de temperatura (BMP180) funcione. En este link :https://learn.sparkfun.com/tutorials/bmp180-barome...
Una vez descargada la librería se debe hacer clic en "programa" , ahora se pone el cursor sobre "incluir librería" y se hace clic en "añadir librería.ZIP" y se selecciona la librería descargada (BMP180_Breakout_Arduino_Library-master.zip).
A continuación, se hace clic en "Archivo" y después en "Ejemplos". Se selecciona el ejemplo Sparkfun BMP 180 y posteriormente a "BMP180_altitude_example."
Este código que nos va a aparecer es nuestro código base.
// Your sketch must #include this library, and the Wire library.
// (Wire is a standard library included with Arduino.):
#include #include
// You will need to create an SFE_BMP180 object, here called "pressure":
SFE_BMP180 pressure;
double baseline; // baseline pressure
void setup() { Serial.begin(9600); Serial.println("REBOOT");
// Initialize the sensor (it is important to get calibration values stored on the device).
if (pressure.begin()) Serial.println("BMP180 init success"); else { // Oops, something went wrong, this is usually a connection problem, // see the comments at the top of this sketch for the proper connections.
Serial.println("BMP180 init fail (disconnected?)\n\n"); while(1); // Pause forever. }
// Get the baseline pressure: baseline = getPressure(); Serial.print("baseline pressure: "); Serial.print(baseline); Serial.println(" mb"); }
void loop() { double a,P; // Get a new pressure reading:
P = getPressure();
// Show the relative altitude difference between // the new reading and the baseline reading:
a = pressure.altitude(P,baseline); Serial.print("relative altitude: "); if (a >= 0.0) Serial.print(" "); // add a space for positive numbers Serial.print(a,1); Serial.print(" meters, "); if (a >= 0.0) Serial.print(" "); // add a space for positive numbers Serial.print(a*3.28084,0); Serial.println(" feet"); delay(500); }
double getPressure() { char status; double T,P,p0,a;
// You must first get a temperature measurement to perform a pressure reading. // Start a temperature measurement: // If request is successful, the number of ms to wait is returned. // If request is unsuccessful, 0 is returned.
status = pressure.startTemperature(); if (status != 0) { // Wait for the measurement to complete:
delay(status);
// Retrieve the completed temperature measurement: // Note that the measurement is stored in the variable T. // Use '&T' to provide the address of T to the function. // Function returns 1 if successful, 0 if failure.
status = pressure.getTemperature(T); if (status != 0) { // Start a pressure measurement: // The parameter is the oversampling setting, from 0 to 3 (highest res, longest wait). // If request is successful, the number of ms to wait is returned. // If request is unsuccessful, 0 is returned.
status = pressure.startPressure(3); if (status != 0) { // Wait for the measurement to complete: delay(status);
// Retrieve the completed pressure measurement: // Note that the measurement is stored in the variable P. // Use '&P' to provide the address of P. // Note also that the function requires the previous temperature measurement (T). // (If temperature is stable, you can do one temperature measurement for a number of pressure measurements.) // Function returns 1 if successful, 0 if failure.
status = pressure.getPressure(P,T); if (status != 0) { return(P); } else Serial.println("error retrieving pressure measurement\n"); } else Serial.println("error starting pressure measurement\n"); } else Serial.println("error retrieving temperature measurement\n"); } else Serial.println("error starting temperature measurement\n"); }
Ahora otra vez en ejemplos buscamos el código de "Hello World" del Liquid Crystal Display.
// include the library code:
#include
// initialize the library with the numbers of the interface pins LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
void setup() { // set up the LCD's number of columns and rows: lcd.begin(16, 2); // Print a message to the LCD. lcd.print("hello, world!"); }
void loop() { // set the cursor to column 0, line 1 // (note: line 1 is the second row, since counting begins with 0): lcd.setCursor(0, 1); // print the number of seconds since reset: lcd.print(millis() / 1000); }
Se copian y se pegan las primeras líneas del código de Hello World donde dice "LiquidCrystal" (en anaranjado) y se pegan debajo de las primeras dos líneas del código base. Ahora unimos los dos "void.setup" de ambos códigos , claramente en nuestro código base. Por último, cambiamos todos los "Serial" por lcd (liquid crystal display).
Código final:
#include
#include // include the library code: #include // initialize the library with the numbers of the interface pins LiquidCrystal lcd(12, 11, 5, 4, 3, 2); // You will need to create an SFE_BMP180 object, here called "pressure": SFE_BMP180 pressure; double baseline; // baseline pressure void setup() { // set up the LCD's number of columns and rows: lcd.begin(16, 2); // Print a message to the LCD. lcd.print("hello, world!"); lcd.begin(9600); lcd.println("REBOOT"); // Initialize the sensor (it is important to get calibration values stored on the device). if (pressure.begin()) lcd.println("BMP180 init success"); else { // Oops, something went wrong, this is usually a connection problem, // see the comments at the top of this sketch for the proper connections. lcd.println("BMP180 init fail (disconnected?)\n\n"); while(1); // Pause forever. } // Get the baseline pressure: baseline = getPressure(); lcd.print("baseline pressure: "); lcd.print(baseline); lcd.println(" mb"); } void loop() { double a,P; // Get a new pressure reading: P = getPressure(); // Show the relative altitude difference between // the new reading and the baseline reading: a = pressure.altitude(P,baseline); lcd.print("relative altitude: "); if (a >= 0.0) Serial.print(" "); // add a space for positive numbers lcd.print(a,1); lcd.print(" meters, "); if (a >= 0.0) Serial.print(" "); // add a space for positive numbers lcd.print(a*3.28084,0); lcd.println(" feet"); delay(500); } double getPressure() { char status; double T,P,p0,a; // You must first get a temperature measurement to perform a pressure reading. // Start a temperature measurement: // If request is successful, the number of ms to wait is returned. // If request is unsuccessful, 0 is returned. status = pressure.startTemperature(); if (status != 0) { // Wait for the measurement to complete: delay(status); // Retrieve the completed temperature measurement: // Note that the measurement is stored in the variable T. // Use '&T' to provide the address of T to the function. // Function returns 1 if successful, 0 if failure. status = pressure.getTemperature(T); if (status != 0) { // Start a pressure measurement: // The parameter is the oversampling setting, from 0 to 3 (highest res, longest wait). // If request is successful, the number of ms to wait is returned. // If request is unsuccessful, 0 is returned. status = pressure.startPressure(3); if (status != 0) { // Wait for the measurement to complete: delay(status); // Retrieve the completed pressure measurement: // Note that the measurement is stored in the variable P. // Use '&P' to provide the address of P. // Note also that the function requires the previous temperature measurement (T). // (If temperature is stable, you can do one temperature measurement for a number of pressure measurements.) // Function returns 1 if successful, 0 if failure. status = pressure.getPressure(P,T); if (status != 0) { return(P); } else lcd.println("error retrieving pressure measurement\n"); } else lcd.println("error starting pressure measurement\n"); } else lcd.println("error retrieving temperature measurement\n"); } else lcd.println("error starting temperature measurement\n"); }
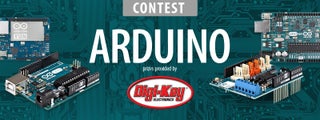
Participated in the
Arduino Contest 2016