Introduction: Make a Killer Augmented Reality App
This tutorial is going to show you how to use API's in Unity so you can make your augmented reality apps smarter. Everything is going to be geared towards beginners. We will be using the Unity 3D video game engine because this will allow us to make cross platform apps that will work on IOS or Android. The Vuforia plugin will allow us to access the camera stream and Unity's WWW class will allow us to communicate with API's via the http protocol. This is the same mechanism that is used when you access websites in your browser.
I think the real killer application of augmented reality is not gaming, but rather using AR as a tool to actually enhance your current perception of reality. The way to do this is by making connected apps that leverage the power of the internet.
As far as software you will need Unity 3D: https://unity3d.com/
and the Vuforia SDK: https://developer.vuforia.com/downloads/sdk
Step 1: Unity's WWW Class
So, Unity’s WWW class is the first step in doing this stuff because it allows you to make requests via http to a server. When you visit a website you are doing just this, you are typing a url into your browser and making an http request to the server at that address. The web server returns you the html code that your browser uses to render the webpage.
Step 2: Lets Make an HTTP Request
To illustrate this further lets make a request to www.unity.com.
Start a fresh unity project, create a C# script called test and drag it onto your main camera.
First create an Enumerator called request, we want to work with www objects inside a coroutine so we don’t halt the entire program while things are processing. Then lets make sure this code will run by starting the coroutine in the start function.
Inside the coroutine lets create a string and define it as the web address to Unity.
Create a new www object and pass in the url.
Yield return www (so that we don’t continue until that operation is done).
Create a string called html and define it as www.text, which a string of text that was returned from our request.
When we print this out we will see that this is the html of the unity.com
Step 3: Intro to Web Scraping.
Now without getting into api’s just yet, we could parse this data to pull out some useful information from websites to use in our app. This is called web scraping. Say for example we want to extract the title of the webpage.
We know from standard html that the title will be between two title tags.
A start title and end title tag: <title>This Is The Title Of The Webpage</title>
Just know there are better and cleaner ways to do this, but for the sake of time lets just manipulate the string to pull out what we want.
Right now we have got a string separated by individual characters. So when we print html[2] we get the third character (since indices start at 0). Lets instead create a list of strings and split it by the new line character. Now when we print htmlList[2] we get the third line of text.
To find the line that contains “title” we can loop through every line of text using a for loop.
We can find the desired line by checking if the current line contains the word title. Once we have found that line we can replace the first title tag with an empty string, and then replace the second title tag with an empty string. Lets print the result and then we can break here because we don’t need to go any further. The result will now contain the title of the webpage. If you want a better, but slightly more complicated method of achieving this, look into regular expressions or REGEX.
Step 4: API's
Now lets look into making an actual API request. We are going to use the Google Custom Search API. First we need a unique key. So search google custom search api key and go to the first link. Create an account if you don’t already have one and create an app. Enable the custom search API for this app and copy the key to your clipboard.
You can do 100 searches a day for free with this particular API.
Step 5: Hi, I'm JSON, Nice to Meet You.
Go back to Unity and lets start by deleting everything up until we set our html string. Lets rename this "json" because we will be getting back a JSON object. Lets just copy our API key anywhere for now.
Go back to google and click on "Using Rest." If you look around here you will find that the api requires 3 parameters, the api key, a custom search engine id, and a search query. Copy the example request and past it into the URL string back in Unity.
Move your license key to the appropriate location in the url, and since we are not going to create a custom search engine, change the cx parameter to cref and make it an empty string.
Create a private string variable called query and set it equal to whatever you want to search for. Go back to the url and remove everything after "q=" and concatenate the string with the query variable we just defined. Now when we print out the response we will have all the google results organized into neat JSON objects.
Step 6: Now What?
Great, now we have more gibberish.
Well, you could extract what you want using a library called LitJSON.
https://github.com/lbv/litjson
That would allow us to neatly deal with all this information.
For a quick fix you could just parse it just like we did with the html from the last example.
Now to get this visible in the game view, right click in the Unity hierarchy and create a new UI text.
Add this using directive to your script: "UnityEngine.UI;"
Now just create a reference to the UI text by defining a public GameObject yourTextObject;
Drag in the text to the empty slot that was just created in the inspector.
Now you can just set it like this:
yourTextObject.GetComponent<Text>().text = "Whatever you want."
Step 7: Let's Take It a Step Further...
I made this tutorial because I learned a lot trying to find a workaround for the lack of a free reverse image search API. Google allows you to perform a reverse image search in the browser but not via http like all their other services.
I was determined to get this to work so here is what I did:
It turns out the result of a request to the google reverse image search gets redirected multiple times and finally is rendered as html via javascript. So, getting the data that we need is not possible in Unity alone. The only way I could think to get it was to create my own api of sorts in php. I could pass the url of the image I want to search from Unity to a php script I have hosted on a server. The php script follows the redirects from google using cURL and is able to view the javascript rendered html using Node.js, which is a server side javascript environment.
If you are interested in learning more about this check out the video in the intro.
Thanks for looking, and let me know if you have any questions!
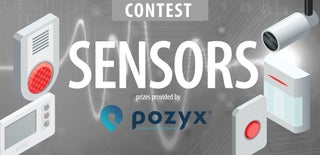
Participated in the
Sensors Contest 2017
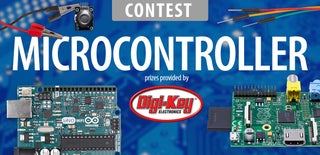
Participated in the
Microcontroller Contest 2017