Introduction: Merry Grinchmas Sweater, Thermal Printer + GemmaM0
The Merry Grinchmas sweater is an interactive garment that provides a wide range of personalized printed messages as a complain whenever someone touches the Grinch's hat pompon. Anti-Christmasy messages coming through a thermal printer controlled by Gemma MO, Arduino, and Capacitive Sensing.
Step 1: Material List
- Gemma MO
- Thermal printer Guts - https://www.adafruit.com/product/2753
- Conductive Fabric Tape
- Loose Sweater
- Felt
- Roving + felting kit
- Conductive Fiber - https://www.adafruit.com/product/1088
- Copper thread
- Resistors ( 3.3k + 2.2k)
- Power Supply - 7.5V , 3A
- Li-Po Battery
- Solder
- Fabric Glue
- Arduino Uno, Alligator Clips & breadboard (for testing)
Step 2: Test + Setup Printer
First of all, testing the printer to see if it has enough power, otherwise, when pressing the reset button on the printer to print the test page it will keep printing the same line over and over (picture 1).
When the test print comes out, it's time to check the BaudRate as the Printer Guts work at 9600 and by default Adafruits Library, uses 19200 like other thermal printers that they sell. Check it here: https://learn.adafruit.com/mini-thermal-receipt-printer/first-test
Picture 3 shows specificly how to wire the printer.
The test helps to calibrate the different font styles to create any customized design. (Picture 4).
There is a random number that chooses between 12 different sentences including different textual styles for a heading, body text and signature.
Lastly, to add any picture, it needs to be converted into a Bitmap picture. (Picture 6). Here the choice was the Grinch. (Picture 7)
One last note. Using the printer with Gemma M0, requires some adjustments to use the Hardware serial (Picture 8).
Step 3: Designing the Program
What do I expect about my program?
1) I have an RGB led that gives visual feedback about the printer. Green for ready, red for printing.
As the Gemme M0 has an embedded Neopixel, to code it, the led needs to be addressed as if it was an LED strip.
#define NUMPIXELS 1 // Number of LEDs in stripvoid setup() {
strip.begin(); // Initialize pins for output strip.show(); // Turn all LEDs off ASAP
}
void loop(){
strip.setPixelColor(0, 255, 127, 0);
strip.show();
}
2) A capacitive sensor that triggers the system.
Capacitive sensing requires a library and defining some variables. It uses one Analog Input that needs to be calibrated. I may require some testing to change the values of touch.
#include "Adafruit_FreeTouch.h"
int touch = 1000;
long oldState = 0;
#define CAPTOUCH_PIN A0void checkpress() {
// Get current button state. long newState = qt_1.measure(); Serial.println(qt_1.measure()); if (newState > touch && oldState < touch) { // Short delay to debounce button. delay(20); // Check if button is still low after debounce. long newState = qt_1.measure(); } if (newState < touch ) { // Do nothing } else { //Do this }
3) Print different messages everytime the system is triggered.
The program will randomize a number everytime the program runs
void printChristmas(){
randomSeed(analogRead(0)*analogRead(1)); randomNumber = random(1,12);
printer.inverseOn(); printer.println(F("Xmas Inc. Presents")); printer.inverseOff();
switch (randomNumber) { case 1: // statements printer.println(F("")); // Set text justification (right, center, left) -- accepts 'L', 'C', 'R' printer.justify('L'); printer.println(F("That's what it's all about, isn't it? That's what it's always been *about*. Gifts, gifts... gifts, gifts, gifts, gifts, gifts! You wanna know what happens to your gifts? They all come to me. In your garbage. You see what I'm saying? In your *garbage*. I could hang myself with all the bad Christmas neckties I found at the dump. And the avarice..")); break; case 2: // statements printer.println(F("")); // Set text justification (right, center, left) -- accepts 'L', 'C', 'R' printer.justify('L'); printer.println(F("Oh, the Who-manity.")); case 3: // statements printer.println(F("")); // Set text justification (right, center, left) -- accepts 'L', 'C', 'R' printer.justify('L'); printer.println(F("Oh, no, the sleigh, the presents, they'll be destroyed, and I care!")); break; case 4: // statements printer.println(F("")); // Set text justification (right, center, left) -- accepts 'L', 'C', 'R' printer.justify('L'); printer.println(F("Blast this Christmas music. It'sjoyful and triumphant.")); break; case 5: // statements printer.println(F("")); // Set text justification (right, center, left) -- accepts 'L', 'C', 'R' printer.justify('L'); printer.println(F("Am I just eating because I'm bored?")); break; case 6: // statements printer.println(F("")); // Set text justification (right, center, left) -- accepts 'L', 'C', 'R' printer.justify('L'); printer.println(F("There is, however, one teeny-tiny Christmas tradition I find quite meaningful...[holds up mistletoe]Mistletoe.[puts mistletoe over his butt]Now pucker up and kiss it, Whoville![wiggles mistletoe]Boi-yoi-yoi-yoing!")); break; case 7: // statements printer.println(F("")); // Set text justification (right, center, left) -- accepts 'L', 'C', 'R' printer.justify('L'); printer.println(F("Now you listen to me, young lady! Even if we're *horribly mangled*, there'll be no sad faces on Christmas.")); break; case 8: // statements printer.println(F("")); // Set text justification (right, center, left) -- accepts 'L', 'C', 'R' printer.justify('L'); printer.println(F("Holiday who-be what-ee?")); break; case 9: // statements printer.println(F("")); // Set text justification (right, center, left) -- accepts 'L', 'C', 'R' printer.justify('L'); printer.println(F("Are you having a holly, jolly Christmas?")); break; case 10: // statements printer.println(F("")); // Set text justification (right, center, left) -- accepts 'L', 'C', 'R' printer.justify('L'); printer.println(F("And they'll feast, feast, feast, feast. They'll eat their Who-Pudding and rare Who-Roast Beast. But that's something I just cannot stand in the least. Oh, no. I'M SPEAKING IN RHYME!")); break; case 11: // statements printer.println(F("")); // Set text justification (right, center, left) -- accepts 'L', 'C', 'R' printer.justify('L'); printer.println(F("The avarice never ends!I want golf clubs. I want diamonds. I want a pony so I can ride it twice, get bored and sell it to make glue. Look, I don't wanna make waves, but this *whole* Christmas season is...")); break; }
// Test more styles printer.boldOn(); printer.justify('R'); printer.println(F("Grinch")); printer.boldOff(); printer.println(F("")); printer.justify('L'); // Test character double-height on & off printer.doubleHeightOn(); printer.println(F("Merry Grinchmas!")); printer.doubleHeightOff(); printer.println(F("")); printer.println(F(""));
// Print the 75x75 pixel logo in adalogo.h: printer.printBitmap(grinch_width, grinch_height, grinch_data);
printer.println(F("")); printer.println(F(""));
printer.sleep(); // Tell printer to sleep delay(3000L); // Sleep for 3 seconds printer.wake(); // MUST wake() before printing again, even if reset printer.setDefault(); // Restore printer to defaults }
Step 4: Soft Circuit
As the soft circuit needs to be placed safely in the sweater, I paid special attention to the circuit design (Picture 1) and the component's placement.
After testing the complete circuit using alligator clips and breadboard (Pictures 2 & 3), I jumped into placing temporary components on the felt, before sewing and soldering (Picture 4 &5).
Important note: The wiring including the resistors is essential as this circuit needs a voltage divider to prevent the current damaging the board. --> (Picture 6)
Last step here, testing everything (Picture 7)
Step 5: Putting Everything Together
1) Creating the felt font face (Picture 1 &2)
2) Sew Letters (Picture 3)
3) Create a pouch for the paper roll and a structure for the printer and the Li-po battery holder ( Picture 4 & 5). Everything is sewn to the sweater.
4) Create the Grinch face that works as the printer cover. The circuit continues under the hat with a layer of fabric conductive tape that connects to the pompon. The pompon is made of green wool roving and conductive fiber. (Picture 6).
5) As the power supply requires a cord to be powered I created a cover made of yarn using a french knitter. (Picture 7 Optional).
6) The very last step, make sure that everything is in place and try it on!
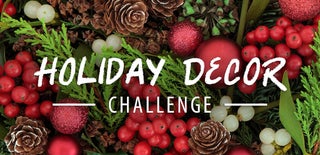
Participated in the
Holiday Decor