Introduction: New Year's Eve LED Sparkle Dress
The idea for this project sprang from my partner's desire to wear a sparkly dress on New Years Eve. It was further fueled by a friends statement: "My dress will be more sparkly than yours." More sparkly? How could I make an already very sparkly dress more sparkly? Technology was the answer! Technology will triumph! This was a challenge I could not refuse!
So what technology could add sparkle to…well, to anything? Ultra bright, individually addressable RGB LEDs of course! Combine them with an Ardunio-compatible microcontroller and accelerometer and you've got the perfect flash as you move setup. A large helping of inspiration and construction techniques (and well-documented Arduino code) came from Becky Stern at Adafruit and her excellent Sparkle Skirt tutorial.
I had several goals in mind while planning to tackle this challenge. I was looking for a subtle but stunning, non-geeky effect that did not detract from the elegance of the dress. I wanted the LEDs and micro controller to be removable and reusable and their removal should be easy while still leaving the dress in a wearable, unaltered state. At the same time I wanted them to be securely attached, comfortable to wear and undetectable when not in use...a seamless experience for both the user and her onlookers.
My apologies for the quality of the video. It was very difficult to find a light setup that showed both the sparkle of the sequins and the flash of the LEDs. I hope that you can see enough of both to get an idea of how cool it looks in real life.
Step 1: Tools and Supplies
Parts
- Sequined or other dress/skirt with a sheer outer skirt, preferably with a metallic finish and opaque underskirt
- 1 x Adafruit Flora microcontroller
- 1 x Adafruit FLORA Accelerometer/Compass Sensor
- 12 x Adafruit Flora RGB Smart Neopixel v2
- 1 x Lithium Ion Polymer Battery - 3.7v 150mAh
- 1 x Adafruit Micro Lipo - USB LiIon/LiPoly charger - v1
- 2 x Stainless Medium Conductive Thread - 3 ply - 18 meter/60 ft
- Cotton thread
- Scrap material
- Clear nail polish
- Straight pins
Tools:
- Sewing needles
- Embroidery hoop
- Measuring tape
- Multimeter
Suggested prerequisites:
- A basic knowledge of Arduino programming and electronics
- Basic sewing skills
Recommended reading:
Step 2: Pick the Dress
For me, the dress (Spectacular Sparkle Dress from French Connection) had already been purchased for this occasion and some of the ideas for this project were developed based on the construction of this particular dress. When choosing a dress I recommend something that has a sheer outer skirt with sequins, glitter or a metallic effect applied to it and a sturdy, opaque underskirt on which the electronics can be attached. This will allow the light of the LEDs to shine through from beneath the outer skirt and mingle with the sparkle of ambient lighting reflecting off the sequins. If your dress has pleats (as this one does) it will create a variable surface that can add randomness to an LEDs position, enhancing the illusion.
Step 3: Plan the Placement*
You will need to decide how and where to attach all the circuitry. The Flora should attach somewhere below the waist to one side and should not interfere with normal movement of the legs or torso (you can test this by dancing, bending over at the waist and deep knee bending). LED placement should be well spaced over the entire area of the skirt while considering the path the conductive threads will need to take (more on this in step 6). If your dress has pleats it is important to consider how a folded pleat may cause the conductive threads to touch and potentially short out. I found that laying the dress out flat and measuring from seam to seam (along the path the LEDs will take) makes it easier to calculate the spacing of the LEDs.
*It was hard to decide if this step should go before or after construction. If you find this step a bit confusing have a quick read through the next two steps...it may help clear things up.
Step 4: Cloth "circuit Board" for Flora and Accelerometer
Take your piece of scrap material and fix into your embroidery hoop. This material will hold the Flora, accelerometer and the conductive thread traces that link the two. It will also provide a straightforward way to attach these components without having to sew them directly to the dress. Sew the four traces between the Flora and the accelerometer connecting the following pads together:
Flora ----- Accelerometer
GND <-> GND
SCL <-> SCL
SDA <-> SDA
3.3V <-> 3V
See the attached picture and wiring diagram for reference.
When you finish each knot, trim the end (leaving almost no tail) and seal the knot using clear nail polish. Use regular cotton thread to help secure the components to the material. I strongly recommend reading through the Adafruit tutorial if this is your first time using conductive thread.
I used double the length of the material so I could fold it over and sew down three sides to form a pouch. This keeps the Flora and accelerometer from rubbing on the outer skirt, hides the status LEDs, and reduces the potential of accidental short circuits. I also used a scrap of material to fashion a small pocket to hold the rechargeable battery.
Step 5: LED Strips
To make it easier to reuse both the Neopixels and the dress I needed a way to make a flexible LED strip that would not interrupt the flow or fit of the dress, add much bulk or be easily distressed or damaged. My partner, while at a craft show, picked up some bias tape thinking it was ribbon (which I asked her to look for). Unknowingly, she had found the perfect material for creating our LED strip. Bias tape (generally used for finishing edges and binding seams) is made from fabric that is cut on the bias (with the fibers running at 45 degrees) which makes it slightly stretchy and causes it to flow and drape more naturally. Single fold bias tape has each raw edge folded in towards the center and pressed flat which made a perfect channel to run the three conductive thread traces between each Neopixel. Use the measurements you made in step 4 to determine how much bias tape you will need for your dress and mark, in chalk or washable fabric pen, the desired placement for each Neopixel. Using your conductive thread, sew the data trace in the center of the bias tape and sew the two power rails on either side between the fold and the data trace. This will allow the flaps of the bias tape to cover and protect the power traces to minimize the risk of short circuits. You should check your work every few pixels by connecting the traces with alligator clips, first to your multimeter to check continuity on the power traces and then to your Flora to make sure you have a consistent connection over the data trace. Be very sure you have no short circuits before connecting your Flora.
Step 6: Code for the Flora
I have used a modified version of the code written for the Adafruit Sparkle Skirt project. This code is well documented and annotated which makes it fairly straightforward to modify. I have tried to annotate my modifications which include adding favorite colors, altering flash duration and timing, and extra randomness for flash patterns.
#include
#include #include // Parameter 1 = number of pixels in strip // Parameter 2 = pin number (most are valid) // Parameter 3 = pixel type flags, add together as needed: // NEO_RGB Pixels are wired for RGB bitstream // NEO_GRB Pixels are wired for GRB bitstream // NEO_KHZ400 400 KHz bitstream (e.g. FLORA pixels) // NEO_KHZ800 800 KHz bitstream (e.g. High Density LED strip) Adafruit_NeoPixel strip = Adafruit_NeoPixel(12, 6, NEO_GRB + NEO_KHZ800); Adafruit_LSM303 lsm; // Here is where you can put in your favorite colors that will appear! // just add new {nnn, nnn, nnn}, lines. They will be picked out randomly // * Changed and added colors // R G B uint8_t myFavoriteColors[][5] = {{175, 0, 175}, // purple {0, 175, 175}, // yellow {175, 175, 175}, // white { 0, 0, 175}, // blue {175, 75, 75}, // pink }; // don't edit the line below #define FAVCOLORS sizeof(myFavoriteColors) / 3 // mess with this number to adjust TWINklitude :) // lower number = more sensitive #define MOVE_THRESHOLD 125 void setup() { Serial.begin(9600); // Try to initialise and warn if we couldn't detect the chip if (!lsm.begin()) { Serial.println("Oops ... unable to initialize the LSM303. Check your wiring!"); while (1); } strip.begin(); strip.show(); // Initialize all pixels to 'off' } void loop() { // Take a reading of accellerometer data lsm.read(); Serial.print("Accel X: "); Serial.print(lsm.accelData.x); Serial.print(" "); Serial.print("Y: "); Serial.print(lsm.accelData.y); Serial.print(" "); Serial.print("Z: "); Serial.print(lsm.accelData.z); Serial.print(" "); // Get the magnitude (length) of the 3 axis vector // http://en.wikipedia.org/wiki/Euclidean_vector#Length double storedVector = lsm.accelData.x*lsm.accelData.x; storedVector += lsm.accelData.y*lsm.accelData.y; storedVector += lsm.accelData.z*lsm.accelData.z; storedVector = sqrt(storedVector); Serial.print("Len: "); Serial.println(storedVector); // wait a bit delay(100); // get new data! lsm.read(); double newVector = lsm.accelData.x*lsm.accelData.x; newVector += lsm.accelData.y*lsm.accelData.y; newVector += lsm.accelData.z*lsm.accelData.z; newVector = sqrt(newVector); Serial.print("New Len: "); Serial.println(newVector); // are we moving if (abs(newVector - storedVector) > MOVE_THRESHOLD) { Serial.println("Twinkle!"); // 'twink' is 'wait' delay, shorter num == shorter twinkle // 'numPix' is how many neopixels to simultaneously light up // Added a simple for loop with several random variables to increase // the variation in flash patterns and simultaneous pixels int numLoop = random(3)+2; for(int t=0; tvoid flashRandom(int wait, uint8_t howmany) { for(uint16_t i=0; i= 0; x--) { int r = red * x; r /= 5; int g = green * x; g /= 5; int b = blue * x; b /= 5; strip.setPixelColor(j, strip.Color(r, g, b)); strip.show(); delay(wait); } } // LEDs will be off when done (they are faded to 0) }
Attachments
Step 7: Attach Flora and LEDs
Once you have everything tested and working to your satisfaction it’s time to attach the LED strip to the Flora circuit and attach it all to the dress. I recommend pinning everything to the dress before you start sewing. This allows you to check placement, drape and comfort before committing it with needle and thread. Pin the Flora pouch to one side of the underskirt and pin the beginning of the LED strip near the D6 pad of the Flora Place pins so that the bias tape sits naturally and follows the flow of the underskirt, pinning down any point that buckles or pulls away from it. When you have everything pinned up you should sew the three LED traces into the Flora and run one final test...make sure your pins are not shorting any of your traces. If everything is working you are now ready to sew! Stitching a tack or two in each of the pinned points should create an anchor strong enough to withstand even the most violent of party twerking sessions…although with this much action you may want to carry a spare battery.
Step 8: SPARKLE!
Thank you for taking the time to look through my very first Instructable. If you have any questions about this project or any constructive feedback on how I could improve this tutorial please let me know.
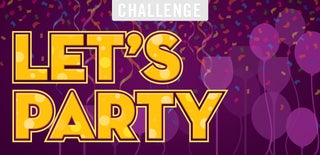
First Prize in the
Let's Party! Challenge
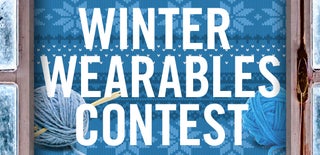
Participated in the
Winter Wearables Contest