Introduction: Pi2uno
Raspberry Pi to Arduino Mega communication using Inter-Integrated circuit or I2C Protocol and blinking an inbuild LED using Raspberry Pi as Master and Arduino as Slave
Supplies
- Raspberry Pi (any version) -1
- Arduino Mega or UNO (here Arduino Mega) -3
- Bread Board -1
- Female to Male Jumpers Cable - 3
- Male to Female Jumpers Cable - 9
Step 1: Step 1: Wiring
We start this project by wiring the connection,
- Take a breadboard,
- Set the connection as follows,
Raspberry Pi SDA (Sky blue color in the picture) –> Shared SDA on breadboard
Raspberry Pi SCL (Sky blue color in the picture)–> Shared SCL on breadboard
Raspberry Pi GND (Black color in the picture)–> Shared GND on breadboard
All Arduino SDA(Gray color in the picture) –> Shared SDA on breadboard
All Arduino SCL (Gray color in the picture)–> Shared SCL on breadboard
All Arduino GND(Black color in the picture) –> Shared GND on breadboard
Step 2: Step 2: Under Standing the Connection
Here the SCL means Serial Clock sends the clock signal for the Slave devices from the Master devices.
SDA means Serial Data sends the Data or Information either from the Master to the Slave or also from the slave to the master.
GND means ground this is the common ground signal for all the microcontroller that is present in this Protocol.
Step 3: Step 3: Arduino Code
This has the Code that needs to be uploaded to the Arduino
If you use more that one arduino Don't forget to comment the slave address because the slave address should be unique.
//Arduino code to receive I2C communication from Raspberry Pi
#include <Wire.h> // Define the slave address of this device. #define SLAVE_ADDRESS 0x04 //Arduino 1 // #define SLAVE_ADDRESS 0x05 //Arduino 2 // #define SLAVE_ADDRESS 0x06 //Arduino 3 // string to store what the RPi sends String str_recieved_from_RPi = ""; void setup() { // setup the LED pinMode(LED_BUILTIN, OUTPUT); // begin running as an I2C slave on the specified address Wire.begin(SLAVE_ADDRESS); // create event for receiving data Wire.onReceive(receiveData); } void loop() { // nothing needed } void receiveData(int byteCount) { while ( Wire.available()) { str_recieved_from_RPi += (char)Wire.read(); } // turn on or off the LED if (str_recieved_from_RPi == "on") { digitalWrite(LED_BUILTIN, HIGH); } if (str_recieved_from_RPi == "off") { digitalWrite(LED_BUILTIN, LOW); } str_recieved_from_RPi = ""; }
Step 4: Step 4: Raspberry Pi Code
Make your you have latest Debian version installed in your Pi,
Else download it from here.
# Raspberry Pi to Arduino I2C Communication
# Python Code import smbus # Slave Addresses for Arduinos ARDUINO_1_ADDRESS = 0x04 # I2C Address of Arduino 1 ARDUINO_2_ADDRESS = 0x05 # I2C Address of Arduino 2 ARDUINO_3_ADDRESS = 0x06 # I2C Address of Arduino 3 # Create the I2C bus I2Cbus = smbus.SMBus(1) aSelect = input("Which Arduino (1-3): ") bSelect = input("On or Off (on/off): ") if aSelect == 1: SlaveAddress = ARDUINO_1_ADDRESS elif aSelect == 2: SlaveAddress = ARDUINO_2_ADDRESS elif aSelect == 3: SlaveAddress = ARDUINO_3_ADDRESS else: # quit if you messed up quit() # also quit if you messed up if bSelect != "on" or bSelect != "off": quit() BytesToSend = ConvertStringsToBytes(bSelect) I2Cbus.write_i2c_block_data(SlaveAddress, 0x00, BytesToSend) print("Sent " + SlaveAddress + " the " + bSelect + " command.") # This function converts a string to an array of bytes. def ConvertStringToBytes(src): converted = [] for b in src: converted.append(ord(b))return converted
Step 5: Step 5: Play
Power Up the Devices
Enter the Arduino address in the Raspberry Pi and watch that Arduino's LED glows.
If you any trouble feel free to comment below.
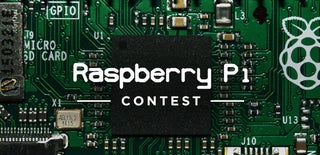
Participated in the
Raspberry Pi Contest 2020