Introduction: Playback Recorder With Raspberry Pi
Hi everyone,
In this instructable I explain how I made a playback recorder using Raspberry Pi. The device is a Raspberry Pi model B+, with 7 push buttons on top, a speaker connected to one of the Pi's usb ports, and a microphone connected to another usb ports. Each button is associated to a sound, so it can play 7 different sounds. The sounds are played after a brief push of the button. To record a new sound, simply push the button for more than 1 second, record after the beep, and let go the button at the end of the record. It doesn't get any simpler than that!
Step 1: Material Needed
For this project I needed:
- A Raspberry Pi model B+ and micro SD card - 29.95 $ + 9.95 $
- A Raspberry Pi plastic case - 7.95 $
- USB speakers - 12.50 $
- A USB microphone - 5.95 $
- A half-sized perma-proto board - 4.50 $
- 7 momentary push buttons - 2.50 $
I also needed:
- Some electrical wire
- Right-angle female headers
- Some wood, black paint and glue for the button case
- A soldering iron and solder
Step 2: The Buttons
The buttons used are quite tall (6mm) so that they can go through the case thickness.
I placed my 7 buttons on a perma-proto board, which is like a breadboard, except components are soldered on it. This is more robust than a breadboard, and cheaper than printing a pcb. Each button links ground to a GPIO on the Raspberry Pi. I don't have resistors here since the Pi already has internal pull-up/down resistors which will be set in the program. In this case I have set them to pull-up (see program below).
The buttons are placed every 4 rows, or every 0.4 in.
Step 3: The Buttons Case
I made a very simple case for the buttons, with plywood sheets and wooden square dowel. The dowel size has to be big enough to contain the button base and board, but small enough to let the button top out of the case. I used a 1/4 in x 1/4 in dowel.
After making sure the board fits in the case, the dowels are glued to the base sheet. Holes are then drilled on the top sheet (the board can be used to precisely make marks every 0.4 inches). All wood parts are painted, the board placed in the case, and the top sheet glued on top of it.
Step 4: The Raspberry Pi
I did not want to solder the wires directly to the Pi, in case I want to use the Pi for something else in the future. I therefore soldered the wires to right-angle female headers, and plugged the headers on the Pi.
The GPIOs used are 21, 26, 20, 19, 13, 6 and 5. The ground pin is also used.
The microphone and speaker are simply plugged in 2 of the 4 usb ports.
The Pi is powered through the micro-usb outlet
Step 5: Programming
To program the Pi, I connected it to internet using an ethernet cable, and controlled it from a remote computer using VNC viewer. However, you cannot use this setup the first time you connect to the Pi, because the OS is not installed yet and SSH is not unabled. So you will need to connect a screen, keyboard and mouse, at least the first time.
It was quite a hassle to find the commands to record and play a sound on the right sound card. These are the commands that worked for me:
- aplay -D plughw:CARD=Device_1,DEV=0 0.wav
- Plays 0.wav
- arecord 0.wav -D sysdefault:CARD=1 -f cd -d 20
- Records for maximum 20 seconds in file 0.wav, with cd quality
The sound files are located in the default directory (/home/pi). A sound file for the beep is also necessary, placed in the default directory and called beep.wav.
The python code itself is the following:
Step 6: Run the Python Script at Each Startup
To run the python script at each Pi's startup, the following lines are put in a file called playback.desktop in folder /home/pi/.config/autostart/
Step 7: End Note
Please tell me what you think of this project in the comment section, let me know of your recommendations, and vote for me in the Raspberry Pi contest if you liked it.
Looking forward to reading you!
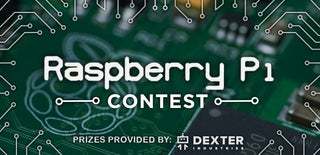
Participated in the
Raspberry Pi Contest 2017