Introduction: Project Frankenstein
"This instructable was created by Howard Kaplan in fulfillment of the project requirement of the Makecourse at the University of South Florida (www.makecourse.com)". 3D Printing was done at the Advanced Visualization Center at the University of South Florida (www.avc.web.usf.edu).
Description:
Parts:
1x AC to DC Power Supply Dual Output 5 Volt 12 Volt 4 Amp 1 Amp 32 Watt
A lot of Wires and Jumper Wires
Step 1: Arduino Code:
/******************************************PURPOSE: Project Frankenstein
Created by Howard Kaplan
DATE: 4.22.2015
This sketch used the HCSR04.h class and HCSR04.cpp to get the sensor input and stores the current and previous distances using the Serial Monitor - the distance of the detected object is displayed and determined as approaching or retracting based on the current and previous distance. Additional components such as LEDs and Servos are used and their respective pins are turned on and off depending on the range sensor values. The sketch also uses the Servo and Pitches libraries.
*******************************************/
// Libs
#include "HCSR04.h"//include calls the header / class file
#include // calls the servo lib files
////MUSIC
#include "pitches.h"
////Range Sensor#define echoPin 13 // This is the echo pin for range sensor
#define triggerPin 12 // This is the trigger pin for range sensorHCSR04ProxSensor distanceSensor(echoPin,triggerPin);//here we call the constructor to instantiate a sensor named "distanceSensor"
///Music Arrays int melody[] = { NOTE_A6,NOTE_C6,NOTE_E6,//3 NOTE_E6,NOTE_A6,//2 NOTE_C6,NOTE_E6,//2 NOTE_E6,NOTE_A6,//2 NOTE_C6, NOTE_E6,//2 NOTE_E6,NOTE_A6,NOTE_C6,//3 NOTE_E6,NOTE_G6,NOTE_B6,//3 NOTE_D6,//1 0, NOTE_E6,NOTE_D6,NOTE_C6,NOTE_B6,//4 NOTE_A5, NOTE_A5//1/23 };// note durations: 4 = quarter note, 8 = eighth note, etc.:
int noteDurations[] = { 4,4,4, 8,4, 4,8, 4,8, 4,8, 4,4,4, 4,4,4, 1, 2, 8,8,8,8, 4,1, }; //// //FAN int fan1 = 4;//Fan on pin 4 //LEDS 2 Pins - 4 Leds int ledReds = 11;//2 red int ledGreens = 10;//2 green //Servos 3 Servo servoEye, servoM; ///servoEye - has 2 servos to 1 pin (right and left eye) int posEye1 = 140; // variable to store the servo position // int musicPin = 9; int pauseBetweenNotes; float distance; //Distance to activate sensor / loop change/***************************setup function****************************************************/
void setup() { Serial.begin(9600);//start serial communication //FAN pinMode(fan1,OUTPUT); //digitalWrite(fan1,LOW); //SERVOs servoEye.attach(5); //eye servoM.attach(3); //mouth //set eye start position servoEye.write(140); //set mouth servoM.write(60); //LEDs pinMode(ledReds, OUTPUT); pinMode(ledGreens, OUTPUT); } /***************************main loop*********************************************************/void loop() {
/******************SENSOR***************************************/ Serial.print("The distance is : "); distance = distanceSensor.readSensor();//here we call the 'readSensor' method to determine the distance Serial.print(distance);// send the measurement to the serial monitor Serial.println(" cm"); if (distanceSensor.getLastValue() - distance > 1) { Serial.println("object is approaching"); }//here we call the 'getLastValue' method to determine the direction of motion if (distanceSensor.getLastValue() - distance < -1) { Serial.println("object is retracting"); }/***************Main Condition Statement******************************************/
////FAN on / off if(distance >= 40.00) { digitalWrite(fan1,LOW); //Leds - Green ON Reds OFF digitalWrite(ledGreens, HIGH); digitalWrite(ledReds, LOW); ///Servo Eyes Look servoEyesBacFor(); ///Servo Mouth Close servoM.write(50); }else{ digitalWrite(fan1,HIGH); //Leds - Green OFF Reds ON digitalWrite(ledGreens, LOW); digitalWrite(ledReds, HIGH); //Servo Stop Straight servoEye.write(140); ///Servo Mouth Open servoM.write(80); //Puttin' on the Ritz playMusic(); } }/*****************EYEs FUNCTION****************************************/
void servoEyesBacFor(){ for(posEye1 = 90; posEye1 <= 180; posEye1 += 1) // goes from 0 degrees to 180 degrees { if(distanceSensor.readSensor() <= 40.00){ break; } // in steps of 1 degree servoEye.write(posEye1); // tell servo to go to position in variable 'pos' delay(5); } for(posEye1 = 180; posEye1 >= 90; posEye1-=1) // goes from 180 degrees to 0 degrees { if(distanceSensor.readSensor() <= 40.00){ break; } servoEye.write(posEye1); // tell servo to go to position in variable 'pos' delay(5); } } /***************************************/ void playMusic(){ for (int thisNote = 0; thisNote < 24; thisNote++) { ///Check distance and break out of loop if out of range if(distanceSensor.readSensor() >= 40.00){ thisNote = 0; break; } int noteDuration = 1000/noteDurations[thisNote]; tone(musicPin, melody[thisNote],noteDuration); pauseBetweenNotes = noteDuration * 1.30; delay(pauseBetweenNotes); noTone(musicPin); } } /***********************The End!*************************/
Step 2: Circuit Schematic & Design:
Connecting the components and the Arduino micro-controller was facilitated using a breadboard. The breadboard was divided in tow sections. One 5 volt and one 12 volt rail. The AC to DC Power Supply (Dual Output 5 Volt 12 Volt 4 Amp 1 Amp 32 Watt) connected at each respective rail. Both shared a common ground. A ground and 5v connection were also made from the breadboard to the Arduino.
5 Volt Side:
The ultra range sensor connected to the ground and positive rail of the breadboard 5v side. The echo and trigger are connected to pins 12 and 13 of the Arduino.
The three servos are connected to the 5v ground and positive rail, and the signal to pins 3 and 5. The two servos for the eyes share the pin connection 5 of the Arduino.
The green and red LEDs share a common ground. The red LEDs are connected to a single pin 11 as are the green LEDs to pin 10. Resistors are used on the board reduce the voltage levels. *Please refer to the image above- right side.
The Piezo Buzzers are connected to the ground of the breadboard and then jumper wires connect to both to a single pin (9).
12 Volt Side:
The Fan is connected to the positive rail on the 12 volt side. Another connection from the fan goes to the breadboard, then to a transistor. A resistor is connected to the transistor and Arduino wire to pin 4. The transistor is also connected to the ground rail. *Please see he above image bottom left.
Step 3: 3D Modeling:
I used various software packages to create the 3d models. Autodesk Inventor and Autodesk Maya were used to create the low resolution mechanical model templates as well as the animations/simulations. Pixologic ZBrush was used to generate the high level of detail, by subdividing the polygon mesh and sculpting with the 3d brushes utilizing a wacom tablet.
3D Files:
The following link is the Autodesk Maya base template. You can use this link to create a different face/head.
Step 4: 3D Printing:
The model was decimated and checked using Autodesk Meshmixer. Once the models were ready for 3d printing they were imported to Makebot's Makerware. 3D printing was done on the Makerbot Replicator 2 (4th generation) in PLA. *It is important to note that I cut the front of the face in half horizontally in order to minimize both the amount of support structure needed and the overall print time. Once the face was completed, I used epoxy to join the two halves.
Step 5: Assembly:
After the pieces were printed I began finishing the model by first sanding, then painting and finally building brackets and attaching the components. I used a low grit sandpaper to smooth the parts. The painting and color detail was done using acrylic paint, and colored sharpies. I used thin aluminum strips that were easy to bent as brackets and screwed the servos to them; then to the inside of the face for the eyes and to the back of the center column for the jaw. The original shelf of the center column (see column 3d model file) was removed because of spacing and servo weight issues. The pegs on the back of the front of the face were also removed from the final 3d print. The back of the head is mounted to the neck/top of the box with an aluminum bracket. The ears are then screwed to the back of the head. Once the back of the head is in position the front of the face should snap to the back of the head by the right and left ears. The jaw is mounted to the servo on the center column. An additional screw was used to hold and position the opposite side of the jaw. *Please see the images above.
Conclusion:
Overall, I believe that the project was an awesome success. Of course going back I would change some parts, such as the jaw geometry and center column, and would experiment with different power sources. The entire process: design, modeling, coding, assembly and finishing was challenging and fun!
Resources
Here is a link for more information on the Tone Function and playing music:
How To Use: Tone Function - Arduino Playing The James Bond Theme
Makecouse:
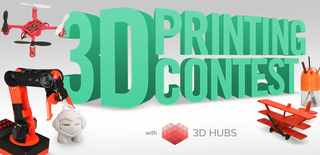
Participated in the
3D Printing Contest
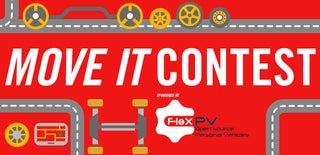
Participated in the
Move It