Introduction: Python Modelling of the Random Walk
My simple piece of code written in Python allows you to model and simulate the random walk and view the diagram of the random walk in real time as it is created. Each time you run the program you will get a different result. The random walk is explained by Wikipedia:
"A random walk is a mathematical formalization of a path that consists of a succession of random steps. For example, the path traced by a molecule as it travels in a liquid or a gas, the search path of a foraging animal, the price of a fluctuating stock and the financial status of a gambler can all be modeled as random walks, although they may not be truly random in reality."
My code makes use of the turtle module to display the random walk and can display 3 different random walks with different step sizes. You can choose the amount of steps that the random walker does. This model can be related to Brownian motion and what is interesting is that on average the particle will move relative to it's original position. I have included annotations in the code to allow people to understand it easier. The interesting random pictures are interesting to look at.
Step 1: Installing Python
To install python 3 visit https://www.python.org/downloads/release/python-34... or you can install the Anaconda distribution which includes other packages such as matplotlib and numpy that are not included in the standard distribution by visiting http://continuum.io/downloads#py34. Remember to install the correct package for your operating system.
Step 2: Paste in the Code
Paste in the code below into a new code editor window and press run module. Enjoy watching the patterns being created and feel free to edit the code to make it more interesting.
import turtle # Allows us to use turtles
import random
wn = turtle.Screen() # Creates a playground for turtles
turtlebase = turtle.Turtle() #make a turtle that doesn't move so the user can see how far the turtle have moved
turtle1 = turtle.Turtle() # Create the 3 moving turtles and assign it to it's name
turtle2 = turtle.Turtle()
turtle3 = turtle.Turtle()
turtle1.color("blue") #make the colour of the turtle blue
turtle1.pencolor("blue") #make it's line colour blue
turtle2.color("Green")
turtle2.pencolor("green")
turtle3.color("red")
turtle3.pencolor("red")
count = 0 #make a variable called count
while count <= 2000: #make a counter loop
turtle1.setheading(random.randint(0,360))# choose an angle between 0 and 360. This tells the heading of the turtle
turtle1.forward(random.randint(-10,10)) #choose a random integer between -10 and 10. This tell how far the turtle is going to move
turtle2.setheading(random.randint(0,360))
turtle2.forward(random.randint(-15,15))
turtle3.setheading(random.randint(0,360))
turtle3.forward(random.randint(-20,20))
count = count +1 #increment the counter to move onto the next section of the loop
wn.mainloop() # Wait for user to close window
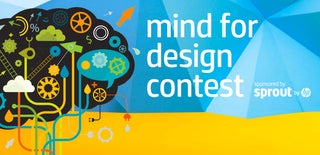
Participated in the
Mind for Design