Introduction: Reading a Push Button Toggle
Push buttons are very simple to use in analog circuits as switches. Pushing the button simply means a close circuit and letting it go back to rest is an open circuit, under the assumption of a normally open push button switch. However, the use of a push button goes beyond as a simple switch when an Arduino or any microcontroller is involved.
An Arduino can read the input signals of a push button switch and act accordingly. In addition, it can store this value and act as a 'programmed toggle flip-flop.' However, this particular use case for reading a push button switch may not be quite as intuitive to make as expected. As such, the focus of this project is the proper use and wiring for a digital reading of a push button to be used to toggle multiple colours of an RGB LED!
Step 1: Tools and Materials
- Arduino 101 or Arduino Uno
- Breadboard
- Push Button Switch
- RGB LED
- 3 pieces of 100Ω resistors
- Jumper Wires
Step 2: Circuitry
Connecting the Arduino Power to the Breadboard
- Connect the 3.3V pin of the Arduino to the red power rail of the breadboard.
- Connect the GND pin of the Arduino to the black power rail of the breadboard.
Wiring the Push Button
- Connect a 10K Ω resistor from one of the pins of the Push Button Switch to the red power rail of the breadboard.
- Connect the same pin above to the digital pin 3 of the Arduino.
- Connect the other pin on the same side to the ground rail of the breadboard.
Lastly, connect the RGB LED to the Arduino.
- Connect the longest pin of the RGB to the common ground rail of the breadboard.
- Connect the remaining three pins to a 100Ω resistor in series to pins 9, 10, 11 respectively.
Step 3: Code
const int buttonPin = 3;
//RGB LED pins const int redPin = 11; const int greenPin = 10; const int bluePin = 9;
//create a variable to store a counter and set it to 0 int counter = 0; void setup() { // Set up the pushbutton pins to be an input: pinMode(buttonPin, INPUT);
// Set up the RGB pins to be an outputs: pinMode(redPin, OUTPUT); pinMode(greenPin,OUTPUT); pinMode(bluePin,OUTPUT); }
void loop() { // local variable to hold the pushbutton states int buttonState;
//read the digital state of buttonPin with digitalRead() function and store the //value in buttonState variable buttonState = digitalRead(buttonPin);
//if the button is pressed increment counter and wait a tiny bit to give us some //time to release the button if (buttonState == LOW) // light the LED { counter++; delay(150); }
//use the if satement to check the value of counter. If counter is equal to 0 all //pins are off if(counter == 0) { digitalWrite(redPin,LOW); digitalWrite(greenPin,LOW); digitalWrite(bluePin,LOW); }
//else if counter is equal to 1, redPin is HIGH else if(counter == 1) { digitalWrite(redPin,HIGH); digitalWrite(greenPin,LOW); digitalWrite(bluePin,LOW); }
//else if counter is equal to 2 greenPin is HIGH else if(counter ==2) { digitalWrite(redPin,LOW); digitalWrite(greenPin,HIGH); digitalWrite(bluePin,LOW); }
//else if counter is equal to 3 bluePin is HIGH else if(counter ==3) { digitalWrite(redPin,LOW); digitalWrite(greenPin,LOW); digitalWrite(bluePin,HIGH); }
//else reset the counter to 0 (which turns all pins off) else { counter =0; } }
Step 4: Demo
As I push the push-button, it first toggles to red, then green, then blue, and finally off. This cycles through all the colours as only 1 push button is pressed!
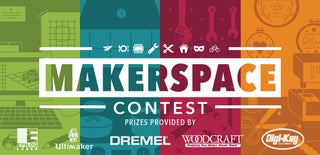
Participated in the
Makerspace Contest 2017