Introduction: Remotely Controlled Cam Dolly ( With Kinect Support)
Hello
In this tutorial i am going to show you how to build a remote controlled camera dolly using an arduino. This can be very usefull for getting cinematic movie shots and will get you more familier with arduinos and code.
Lets begin!
Step 1: Step 1: the Required Parts.
First you need the elektronics to build the circuit.
These parts are:
1x Arduino Uno.
1x Joystick module.
1x bluetooth hc-05 transciever module.
1x A4983 stepper motor controller.
1x Breadboard.
18x ( or preferably more) male to male jumper cables.
4x ( or preferably more ) male to female jumper cables.
1x Nema 17 stepper / 3d printer motor ( I used a 12 volt stepper motor but others will work fine too).
1x Nema 17 plate mount.
1x Pully.
1x 3d Printer belt
2x the size of the overall track length.
2x 8mm rods in the length you want your rail to become ( i used 2 rods of 1 meter each).
4x 8mm rod holders.
4x 8mm linear bearing.
1x 100 microfarad capacitor.
1x Idler pully.
Once you have collected all these parts you can proceed to step 2.
Step 2: Step 2: Circuit Assembly
Once you have alle the electrical components mentioned in step 1 you can assemble the circuit that drives the motor. The above image shows the circuit layout that is used in my project. Once you have connected everyting properly it is time to proceed to step 3.
Step 3: Step 3: the Code
By now you are ready to start coding the circuit you just made. You can do this by downloading the Arduino software from the arduino site ( https://www.arduino.cc/en/Main/Software ). Stepper motors are controlled with pulses, becouse of this reason the speed the stepper motor can rotate depands on the time between each pulse. To use this to our advantage I made the Speed variable. This variable is an integer( a whole number ) wich i use to delay the steps the motor takes. I advice you to perform a couple of test runs before proceding to step 4. some stepper motors arent as fast as others, if your stepper motor is to slow i recommend lowering the value of the Speed variable. If the motor jams however i recommend making the Speed variable higher.
You can directly copy- paste this script into the arduino IDE and connect you arduino via USB. after that use the arrow pointing left in the top left of the screen. Now it will upload the sript to the arduino.
NOTE!!!: before uploading the script to the arduino you need to disconnect the cablet that are intertod into Pin 0 and Pin 1. If you dont do this your pc wont be able to get access to the arduino and you will not be able to upload your scripts. When its done uploasing you can connect the Pin 0 and Pin 1 back up to the bluetooth module and the arduino.
const int stepPin = 2;
const int dirPin = 3;
const int xPin = 0;
const int yPin = 1;
int Speed = 1700;
int tx =1;
int rx =0;
int AppSpeed = 0;
bool App = false;
String Buff;
void setup()
{
pinMode(2, OUTPUT);
pinMode(3, OUTPUT);
pinMode(A0, INPUT);
pinMode( tx, OUTPUT);
pinMode(rx, INPUT);
Serial.begin(9600);
}
void loop()
{
while (Serial.available() > 0)
{
char recieved = Serial.read();
Buff += recieved;
// Process message when new line character is recieved
if (recieved == 'x' || recieved == '\n' )
{
Serial.println(Buff);
if(Buff.toInt() > 1200)
{
AppSpeed = 1200;
}
else
{
AppSpeed = Buff.toInt();
}
Buff = ""; // Clear recieved buffer
}
}
if ( AppSpeed != 0)
{
if(AppSpeed > 0)
{
digitalWrite(dirPin, LOW );
digitalWrite(stepPin, HIGH);
delayMicroseconds(Speed - AppSpeed);
digitalWrite(stepPin, LOW);
delayMicroseconds(Speed - AppSpeed);
}
if(AppSpeed < 0)
{
digitalWrite(dirPin, HIGH);
digitalWrite(stepPin, HIGH);
delayMicroseconds(Speed + AppSpeed);
digitalWrite(stepPin, LOW);
delayMicroseconds(Speed + AppSpeed);
}
}
if(analogRead(xPin) > 523)
{
digitalWrite(dirPin, HIGH);
digitalWrite(stepPin, HIGH);
delayMicroseconds(Speed - 3*(analogRead(xPin) - 523));
digitalWrite(stepPin, LOW);
delayMicroseconds(Speed - 3*(analogRead(xPin)- 523));
}
if(analogRead(xPin) < 515)
{
digitalWrite(dirPin, LOW);
digitalWrite(stepPin, HIGH);
delayMicroseconds(Speed + 3*(analogRead(xPin) - 515));
digitalWrite(stepPin, LOW);
delayMicroseconds(Speed + 3*(analogRead(xPin)- 515)); }
}
Step 4: Step 4: Track Construction
When you have successfully uploaded the srcipt to your arduino ant tested the motor you are ready to start building the track itself. I set the tracks about 10 cm appart from each other on 2 pieces of wood. I then attatched the linear bearings to another piece of wood. This piece is going to be our cart.. I recommend experimenting here, I designed my system on the fly in order to make improvements. You want the cart to slide smoothly and easely on the rails for maximum effect.
When you have build the frame you can start to attach the motor and belt to it. I mounted the Nema 17 mouning plate on the left side of the frame and the idler wheel on tht right. now you have to attach the pulley to the stepper motor and connect the belt. I screwed 2 large screws into the bottem of the cart, then i looped the belt around the idle pully and the stepper motor pully toward the middle. when i got them tightened up i wrapped both ends of the belt around 1 screw each and secured them with a couple of zip ties.
If you have completed these steps correctly you should be able to move the cart using the joystick module. If you are able to controll it it is time for step 5.
Step 5: Step 5: the Android App
Now im going to show you how to build an android app to controll the rails with your phone.
We are going to use app inventor 2 for this step.
You want to try to replicate the pictures above in app inventor. This way you will be sure that your app will work with your arduino.
I have also included the apk file so you can install my app directly to you smartphone or tablet.
At this point you should be able to fully controll your cart using the joystick module and the android app. If you want to be able to controll the rail from a pc using an Xbox 360 kinect then proceed to step 6.
Step 6: Step 6: Kinect
This step is not needed in order to make the rails function, but if you happen to have an old xbox 360 kinect you can use it to track a persons motion and let the kart follow them.
In order for it to work you need:
The kinect for windows 1.8 toolkit and sdk.
a xbox 360 kinect.
a bluetooth capable laptop or pc.
You have to put the kinect on top of or hanging from the bottom of the kart. I have not yet made it possible to pu the kinect in a stationary position while following so it needs to be connected to the moving cart at all times.
When you have unzipped the download folder you will be able to find the .exe as following. First open the "WindowsFormsApplication1" folder , then open the "bin" folder, then open the "Release"folder. After that open the "WindowsFormsApplication1" exe file.
Now the app should have opened. First you have to click the Scan button, this will look for bluetooth devices it can connect to, after roughly 15 seconds the devices will show up in the right text box. The arduino is now called HC-05, if it isnt there click scan a second time and check if you arduino is not already connected to another device. Once you see HC-05 you can connect o it by doubble clicking its name. Once it is succesfully connected it will say so in the left text box.
Once you have connected to the arduino you can click the "Start kinect "button. This will dind the plugged in kinect and start trying to track the users body. Once you have enabled the kinect you have to stand in front of the kinect at a distance of rougly 1,5 meters. This will enable the kinect to locate you and try to track you. It may help to spread your arms slightly or bend through your knees a bit, this way the kinect can recognise you easier for the first time, after that you can move around freely. When the kinect sees you it will rapidly send numbers to the left textbox, this can be usefull to see when it has picked you up. The cart with the kinect on top will follow you from left to right. Do however test the maximum speed your stepper motor can operate at, if you try to move ot faster than it can handle it will jam. This isnt a direct problem becouse it wont damage the motor directly, you will however heve to step in fornt of it again to unjam it.
This is where my tutorial ends, from here on out you can modify and improve your build however you want. I hope you enjoyed.
Attachments
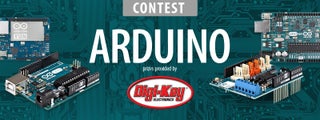
Participated in the
Arduino Contest 2016