Introduction: Robotic Arm
Robotic arms are quite cool stuff and a good project to start in robotics.
In order to create the body of the robotic arm, you could use thick boards as i did or U could use acrylic sheets for the same. Make a sketch of your design and cut the pieces out.
For the electric circuit, u need:-
1. Micro servos * 3
2. 100k Potentiometers
3. 10k resistors
4. Jumper wires
5. Solder iron, solder, circuit board
6. Arduino UNO R3
7. Pushbuttons
8. 9v battery and holder
9. SMPS
10. Body parts
Step 1: Circuit
The circuits are quite simple. In the pictures provided, you will be able to see how one set of servo and potentiometer is connected to arduino. Repeat the same schematic for the different servo sets with a different pin for each servo set. In the overall circuit keep the ground of both the power supplies same. And remember, the power supply to our servos would be from the smps and not the arduino board.
Step 2: Coding
Here is the code for the arm, copy and paste it on your arduino ide and upload the sketch on ur arduino and u r done !!! But remember, you need to create two push buttons to control the base of the arm. push button circuits are quite easy (refer to arduino website for it) . The pins to which each of the given components are to be attached have been mentioned in the code itself!!!
//PROGRAM DEVELOPED BY:Kuldeep Paul
//Copyright: Kuldeep Paul //INDUX INCORPORATION //PROJECT ARMACON //SOUTH POINT HIGH SCHOOL 2015 /*General user guide:- * servo pins-all PWM pins on the board-3-5-6-9-10-11 * potentiometer pins-analog pins 0-1-2-3-4-5 * pushbutton pins-7-8 */ #include
Servo myservo1; // create servo object to control a servo Servo myservo2; Servo myservo3;
int potpin1 = 0; int potpin2 = 1;
// analog pin used to connect the potentiometer int val1,val2,val3,old_val1,old_val2,old_val3; // variable to read the value from the analog pin
//pushbutton-1 const int BUTTONA = 13; // the input pin where the // pushbutton is connected int valA = 0; // val will be used to store the state // of the input pin int old_valA = 0; // this variable stores the previous // value of "val" int stateA = 0; // 0 = Rec off and 1 = Rec on
//pushbutton-2 const int BUTTONB = 12; // the input pin where the // pushbutton is connected int valB = 0; // val will be used to store the state // of the input pin int old_valB = 0; // this variable stores the previous // value of "val" int stateB = 0; // 0 = Play off and 1 = Play on
int s1[100],s2[100],s3[100];
void setup() { myservo1.attach(6); // attaches the servo on pin to the servo object myservo2.attach(5); myservo3.attach(3);
pinMode(BUTTONA, INPUT); // and BUTTON is an input pinMode(BUTTONB, INPUT); // and BUTTON is an input } void loop() { //pushbutton A valA = digitalRead(BUTTONA); // read input value and store it // yum, fresh // check if there was a transition if ((valA == HIGH) && (old_valA == LOW)){ stateA = 1 - stateA; delay(10); } old_valA = valA; // val is now old, let's store it
//pushbutton B valB = digitalRead(BUTTONB); // read input value and store it // yum, fresh // check if there was a transition if ((valB == HIGH) && (old_valB == LOW)){ stateB = 1 - stateB; delay(10); } old_valB = valB; // val is now old, let's store it
if(stateB==0) { int i=0,a=10; val1 = analogRead(potpin1); // reads the value of the potentiometer val1 = map(val1, 0, 1023, 0, 160); // scale it to use it with the servo myservo1.write(val1); // sets position to the scaled value delay(15); // waits for the servo to get there if(val1!=old_val1){s1[i]=val1; i++;} old_val1=val1;
val2 = analogRead(potpin2); // reads the value of the potentiometer val2 = map(val2, 0, 1023, 0, 160); // scale it to use it with the servo myservo2.write(val2); // sets position to the scaled value delay(15); // waits for the servo to get there if(val2!=old_val2){s2[i]=val2; i++;} old_val2=val2;
if(stateA==1) { myservo3.write(a); } else if(stateA==0) {if(a<=170){ a=a+10;} else { while(a>=10); a=a-10; } } }
else if (stateB==1) { myservo1.write(30); delay(1500); myservo1.write(30); delay(1500); myservo1.write(30); delay(1500); myservo2.write(20); delay(1500); myservo2.write(20); delay(1500);
}
}
Step 3: Add to It
Add to this project by adding more servos and more features. Learn as you go!!! Happy Arduinoing!!!
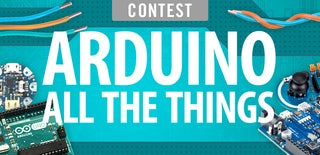
Participated in the
Arduino All The Things! Contest