Introduction: 2-Year Ultra Low-Power LED Flasher
In this Instructable, I am going to try and explain, step by step, how I designed this Ultra Low-Power LED Flasher with the following specification:.
- LED flash rate 1 flash per second
- LED on-time of 5ms
- At least 2 years battery life
- Use standard "AA" size batteries
- Unfortunately, uses two "AA" batteries
- Easy obtainable components
- No surface mount components
- No PC Board
To keep track of this Instructable, I will be monitoring the views using my "Instructable Hit Counter".
Step 1: Project Baseline
Before I decided on a baseline, I tested several common flasher circuits. These included standard flashing LED's, the NE555 timer IC, and transistor flip-flop circuits commonly used. The first problem I experienced, is the amount of components needed to get a short output pulse of around 1 - 5ms every 1 second, or On/Off ratio of 1/1000 to 5/1000. Thus I did not investigate these designs further.
The circuit I decided to use as a starting point, is the LM3909 LED flasher IC. Although production of this very popular chip has ended, they are still freely available on eBay at a reasonable cost. Looking at the LM3909 datasheet, it states that the flasher circuit can run up to 6 months on a single alkaline cell.
So why did I not use the LM3909, you may ask?. Well, in South Africa, our postal system had collapsed, and the average waiting time for an eBay order is between 3 and 6 months....
My Baseline:
- Standard AA battery
- 12 Months minimum operation per battery
- Replace the LM3909 with a micro controller
Attachments
Step 2: AtTiny85 Selection
I decided to make use of an AtTiny85 micro controller, as I have some in my bins. Using the datasheet, I had two options:
- AtTiny85-20
Minimum operating voltage is 2.7V
- AtTiny85V-10
Minimum operating voltage is 1.8V
From above data, it is clear that I will have to use two AA size batteries to power the circuit. So the operating voltage will be taken as 3V nominal for the rest of the design.
The next step was to decide on which AtTiny to use, and for this, I had to do some research on battery capacity.
Conclusion:
The disadvantage of replacing the LM3909 is that the circuit can not operate on a single battery.
Attachments
Step 3: Battery Selection
I will be basing all my calculations on the "AA" Duracell MN1500, as this is what I had on hand. This might not be the best battery for the project.
Minimum operating voltage of the AtTiny are taken, and divided by 2 to give minimum voltage per cell required.
- AtTiny85-20
Vminimum = 2.7V, or 1.35V per cell
From the datasheet (lowest mW curve available), battery capacity is around 0.25Ah at 1.35V.
- AtTiny85V-10
Vminimum = 1.8V, or 0.9V per cell
From the datasheet (lowest mW curve available), battery capacity is around 2.2Ah at 0.9V.
Conclusion
From above findings, it shows that by using the AtTiny85V-10, the operating time on a set of batteries can be greatly increased.
Attachments
Step 4: Calculating Average Current Consumption
Next, we need to determine the average current consumption of our circuit. Using the Duracell MN1500 with an AtTiny85V-10, we have 2.2Ah available.
Ah = Iaverage x 24 hours x 365 days,
2.2Ah = Iaverage x 24 x 365
Iaverage = 251uA
Conclusion
The flashing circuit should use less than 251uA average to get a battery life of one year.
Step 5: Determining Average Current Consumption of the AtTiny85V-10
The test circuit is per schematic, and to keep measurements comparable, I connected the circuit to a 3V regulated power supply.
The test code is straight forward, switching an output on for 5ms, and off for 995ms.
In the Arduino IDE, there are only three internal clock selections available. I will test each of them individually. To learn more about setting the configuration bits, please refer to AVRDude.
- AtTiny85 at 16MHz (with DigiSpark Bootloader)
Set Fuse Low Byte = 0xE1
Set Fuse High Byte = 0xDD
Imax = 13.20mA
Imin = 4.64mA
Iavg = 8.80mA
- AtTiny85 at 8MHz
Set Fuse Low Byte = 0xC2
Set Fuse High Byte = 0xDF
Imax = 4.28mA
Imin = 4.04mA
Iavg = 4.13mA
- AtTiny85 at 1MHz
Set Fuse Low Byte = 0x42
Set Fuse High Byte = 0xDF
Imax = 1.28mA
Imin = 1.00mA
Iavg = 1.10mA, or 1100uA
Conclusion
- Use the internal 1MHz oscillator of the AtTiny85.
- With the AtTiny85 running at 1MHz, and still NO LED connected, the average current of 1100uA is still nowhere near the required 251uA.
Attachments
Step 6: The SLEEP Command
The AtTiny can be placed into a sleep mode when the LED is in the off state. This will shut down certain hardware in the chip, as well as further reducing the average current used.
For the design, I want the LED to flash once every second. By using the SLEEP commands, I will turn on the LED for 5ms, then turn off the LED. Thereafter, the chip will be placed into the SLEEP mode to reduce power.
After 1 second, the build-in watchdog timer will be used to wake up the chip from sleep, and the LED be turned on again.
For this, I will be using additional libraries
#include <avr/sleep.h> #include <avr/wdt.h>
Three new functions were added to the program
setup_watchdog()
With this function, the time-out of the watchdog timer is set to 1 second
system_sleep()
In this routine, the chip is placed into SLEEP mode, and will wait for a time-out of the watchdog timer to wake up.
ISR(WDT_vect)
This is the interrupt vector where the code will jump to once the watchdog timer times out. After the watchdog timer timed out, code will resume to run again.
Findings
With SLEEP mode enabled, the current readings are as follow:
Imax = 0.32mA
Imin = 0.24mA
Iavg = 0.27mA, or 270uA
Conclusion
Even by placing the chip into SLEEP mode, does not reduce the average current to a low enough value.
Attachments
Step 7: AtTiny Hardware Configurations in SLEEP
There are still a few more things that can be done to reduce the power in SLEEP mode
I/O Pin Configuration
When a pin is set up as an OUTPUT, it uses internal circuitry to switch the pin HIGH or LOW. This uses power. The solution might be to switch the pin to an INPUT during SLEEP to save extra power.
<p>// Setup pin as OUTPUT<br> //-------------------- pinMode(Led,OUTPUT); // Flash LED once every second for 5ms digitalWrite(Led,HIGH); delay(5); digitalWrite(Led,LOW); // Setup pin as INPUT //------------------- pinMode(Led,INPUT);</p>
A/D Converter
The A/D converter is not needed, so we will switch it off.
<p>cbi(ADCSRA,ADEN); // switch Analog to Digital converter OFF</p>
Findings
By configuring OUTPUT pins as INPUTS during SLEEP, and turning of the A/D converter, the current readings are as follow:
Imax = 61.2uA
Imin = 4.4uA
Iavg = 8.7uA
Conclusion
The aim was to get an average current of less than 251uA. We are already on 8.7uA.
With an average current of say 10uA for the AtTiny85, almost all power can now be directed towards driving the LED.
Step 8: AtTiny85 Configuration Bits
Lastly, by changing some High Byte fuses, current consumption can be decreased slightly further.
Set Fuse Low Byte = 0x42
Set Fuse High Byte = 0xFF
The 100K pull-up resistor on the RESET pin can now be removed from the circuit.
! ! ! NOTE ! ! !
By setting the High Byte to 0xFF, will disable the external RESET pin, as well as the Serial Programming circuit.. Only use this additional configuration if you are able to reset the AtTiny config bits with a separate programmer.
By disabling the Serial Download Enabled bit, and by disabling the external RESET function, the final readings are:
Imax = 56.0uA
Imin = 4.0uA
Iavg = 8.1uA
Conclusion
With an average current of say 10uA for the AtTiny85, almost all power can now be directed towards driving the LED.
Step 9: LED Calculations
The goal is to use an average of 251uA. With the Attiny85 using only 10uA, we are left with
251uA - 10uA, or 240uA
Assuming a LED current of 20mA, we can now calculate the On time
Ton = 240uA per second / 20mA per second
Ton = 12 ms
The code was changed to flash the LED for 12ms.
LED Resistor
First, we need to determine the forward voltage drop of the LED at 20mA. I will be using a super-bright red LED, with part number WW05A3SRP4-N.
At 20mA, the forward voltage drop of the LED was measured to be 1.993V.
Thus, Rled should be = (3V - 1.993V) / 20mA, or 50R. Use 56R.
Or will it be 56R ???
Findings
Iavg = 140uA, well below the calculated 250uA.
Using a scope (blue trace), the voltage across the resistor was measured to calculate the LED current.
Vres = 640mV, and measured value of R = 55.9R
Thus Iled = 640mV / 55.9R, or 11.4mA.
Conclusion
Sometimes, calculated versus actual values does not correspond. Do not accept this, Find out where the discrepancy comes from.
Looking at Table 21-1 of the AtTiny datasheet, I found my answer :) :). I assumed the output voltage on AtTiny pin will be 3V when turned on. However, the datasheet states that the output voltage can be as low as 2.5V when operating at 3V (last line in the table).
On the scope yellow trace, the pin output voltage can be seen as 2.6V when the LED is on.
To prove this, calculate LED current at 2.6V
Iled = (2.6V - 1.993V) / 55.9R, or 11mA
Attachments
Step 10: Final Design
Calculate the final value of R
Vpin = 2.60V, Vled = 1.993V @ 20mA
R = (2.6V - 1.993V) / 20mA, or 30.4R, use 33R
Iled calculated then as (2.6V - 1.993V) / 33R, or 18mA.
Findings
LED is on for only 12ms every second, so Iavg = 18mA x (12ms/1000ms), or 216uA
Add the 10uA, and total current consumtion is around 230uA
Now, 230uA X 24 hours X 365 days = 2.02Ah
So, the battery lifetime will be 2.2Ah / 2.02Ah, = 1.09 years.
Add some buffer, and set the On Time to 10ms.
Will 2 years be possible?
LED is on for only 6ms every second, so Iavg = 18mA x (6ms/1000ms), or 108uA
Add the 10uA, and total current consumtion is around 120uA
Now, 120uA X 24 hours X 365 days = 1.05Ah
So, the battery lifetime will be 2.2Ah / 1.05Ah, = 2.1 years.
Add some buffer, and set the On Time to 5ms.
Conclusion
- With two "AA" Durcell MN1500 batteries, it is possible to flash a single LED for 10ms every second for about a one year using a AtTiny85V-10.
- With two "AA" Durcell MN1500 batteries, it is possible to flash a single LED for 5ms every second for about a two years using a AtTiny85V-10.
Step 11: Building the LED Flasher
To complete the build, you will need the following:
- 1 x 33R resistor
- 1 x high-bright red LED
- 1 x AtTiny85V-10
- 1 x 8-pin IC socket
- 1 x project box
- 1 x dual "AA" battery holder with leads
- 2 x "AA" Duracell MN1500 batteries
- 1 piece of vero board
Building Instructions
- Cut of a piece of vero board in the required size. Use attached photos for reference
- Cut the four tracks on the vero board as indicated.
- Solder the jumper in place before adding the IC socket.
- Solder the resistor (33R) and IC holder onto the vero board.
- Next, solder the battery holder leads to the vero board
- Add the LED to the vero board. The LED can be soldered directly onto the board, or via two wires.
- Drill a 5mm hole for the LED in the project box.
- Program the AtTiny85V-10. Remember to compile and program the AtTiny using the 1MHz option.
- Insert the AtTiny into the IC socket.
- Secure the vero board and LED to the project box.
- Add the two batteries, and make sure LED is flashing.
- Close the project box.
- Remember to write the date on the back of the unit, so when the batteries does go flat, you will be able to calculate the actual battery life.
Sit back, and relax as you determine if the Ultra Low-Power LED Flasher will be flashing the LED for the next two years.
Thanks for reading.
Regards,
Eric Brouwer
Attachments
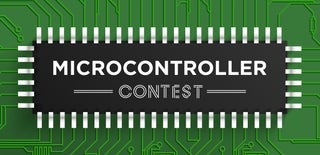
Participated in the
Microcontroller Contest