Introduction: Voice Controlled Android Mood Light
I needed to create a class for our local Maker Group. Something that guaranteed even first-time visitors a definite win and big reward with no muss, no fuss, and no specialized tools or materials. The students needed to take home something both functional and fun that they could show their friends, do it in one afternoon - and it had to be free. (And hopefully bring them back for more, or for a related class) So I created this project.
Turn your old phone or tablet into a voice-controlled mood-light and status board. FREE!!! Easy to do in one afternoon - NO EXPERIENCE REQUIRED (Sunday, Sunday Sunday)
Anyone with an Android device and access to a computer can program a voice controlled application in an hour or two. It uses the free MIT App Inventor, so there's no cost. They can easily customize it, then add their own craftiness to a covering or case. And they can bring the same thing back to class and keep expanding and improving their projects for several months.
The project provides a good starting place for programming, but isn't quite enough to really satisfy - it's designed to bring people back for the next class. Evil, I know. But it leads folks to Arduino, which leads to Raspberry Pi, which leads to electronics and soldering. If they are happy with the basic app and don't want to program any more, well they can make a custom case for it when they come back to the Maker Group for classes on fabric and sewing, paper and drawing, woodwork and frame-making or even 3d design and printing.
Basic Requirements
- An old Android phone or tablet (currently 2.3 or later)
- Internet Access
- An App Inventor Account (Free)
- Preferably, a computer with Chrome browser installed
Preparation
You do need to be familiar with MIT's App Inventor program. There are plenty of Instructables about it (some quite advanced). But the best place to learn App Inventor is on their web site and excellent series of tutorials. Most people learn the basic concepts in a matter of minutes. After all, this is the same shop that created the Scratch programming environment, and the original LEGO Mindstorms programming app. If you are too old to know about these, ask your local school aged kid to help you.
If you are teaching the class, you should probably be familiar with most of the components and commands. Someone in the class is almost guaranteed to ask to do something different than what's shown in this tutorial. You can stick to the script and only do what's shown here. But I've noticed we get a much higher rate of returning visitors when we can help everyone create a unique version with "advanced" features that they can show to their friends.
So familiarize yourself with the basics, then come on back for a step-by-step tutorial.
Step 1: Design and Set Up
Create a Canvas.
- In "Designer" mode, go to the "Drawing and Animation" palette.
- Drag a "Canvas" to the screen.
- Set the width and height of the canvas to "Fill Parent"
- We will create the code to change the color of the canvas in later steps.
Create Voice Recognition
- From the "Media" palette, drag "SpeechRecognizer" to the screen.
- This is the component that will listen for our voice commands.
- We will configure this item later on.
Create Speech Capability
- Also from the "Media" palette, drag a "TextToSpeech" object to the screen.
- We will use this component to create spoken prompts for the user.
- This item will also be configured later in the tutorial.
We just installed the basic components for our app - all in a few seconds. Now we move on to configuring and coding them. For that, we need to switch into the "Blocks" mode. Look in the upper right corner of the screen and click the Blocks button. If you need to get back into Designer mode, just click the Designer button.
Step 2: Start the SpeechRecognizer
REMEMBER: Switch to "BLOCKS" Mode : Be sure to switch to block mode by clicking on the button in the upper right corner of the screen. A new set of palettes will appear. We will use these palettes and blocks to program the mood light.
We want to start listening for commands as soon as the application opens. To do that we will "call" the SpeechRecognizer object when the first screen "initializes." AppInventor has automatically created a "screen" for us. Every app has at least one screen, some have several. We only need the default one.
Initialize the Screen
- Click on the Screen object in the left-hand menu.
- From the fly-out menu, drag the "When Screen Initialized do" object onto the stage.
Start the Speech Recognizer
- Click on the "SpeechRecognizer" object in the left menu
- Drag the "call SpeechRecognizer getText" object onto the stage
- Plug this command inside the Screen Initialized block
Now, the program automatically starts listening for voice commands (getText) as soon as the first screen loads (initializes). Next, we tell the computer what to do when it hears commands.
Step 3: Creating the Decision Tree
The computer is now listening for voice commands, so next, we need to specify what to do after it hears certain words. In this project we will mostly use color names like Blue, Green and Yellow. When the computer hears those words it will change the color of the Canvas object.
We do this by testing to see if the voice command matches any words that we have specified. If the voice command does match a pre-defined word then we want the computer to take certain actions - like change the canvas color and give some verbal feedback. If no match is found we need to tell the user something went wrong.
We begin by creating a blank frame to hold all the tests and actions.
What to Do After Getting a Voice Command
- Click on the SpeechRecognizer in the left menu
- Drag the "After Getting Text" Block onto the stage
- (Place the block directly onto the stage, NOT inside the previous block)
Create Test Slots
- Click on Control in the Built-In section of the left-hand menu
- Drag an If-Then command block onto the stage
- Plug the If-then block inside the afterGettingText block
- Click on the blue gear icon on the If-then block
- From the pop-up that appears, drag several Else-If sub-blocks into the main if-then block
- Also drag one Else sub-block to the end of the list
In the next step, we will begin to fill these empty slots with tests and actions - the heart of the program.
Step 4: Test for Matches
The application is listening for spoken commands, and there is a framework to fill with tests on those voice commands. So now, let's define the tests. First, we tell the computer to test whether two objects are equal, then define the first object as the voice command and the second object as a piece of text. We will only create one test here, but the same technique is used to create six or ten or a hundred tests. If the first test is a match then the program takes an action, else it moves to the next test and so on.
Create an Equality Test
- Click on Logic in the Built-In section of the left menu
- Drag an = (equals) test onto the stage
Set First-Item to the Voice Result
- Click on the SpeechRecognizer in the left menu
- Drag a Result block onto the stage
- Plug the SpeechRecognizer.Result into the left slot of the Equality test block
Set Second-Item to a Text Block
- Click on Text in the Built-in section of left menu
- Drag a basic text field block onto the stage
- In that text block, type in the word you want to test for
- Plug the text block into the right-hand slot of the Equality test block
Put the Test into the Right Place
- Now, plug the entire Equals-Test block into the IF Slot of the decision tree
- In the next steps we will assign some actions to take when the test is true
EXAMPLE: If the user says "blue" the program will capture that word in a "result" bin. Then it will test to see if that result-word (blue) matches the word you typed inside the text block. If it does match, the program will then execute the actions in the "then" portion of the block (we will define the actions in the next steps). If the voice command does not match the text, then the program moves on to the next test until it does find a match or reaches the "something's wrong" final else statement.
NOTE: The voice command doesnot have to be a color. In the example code we use the words "dark" and "light" to trigger black and white. We could just as easily use words like:
- Mom/Dad/Billy/Suzy
- Happy/Sad/Angry/Hungry
- Sleeping/Studying/Broadcasting/Party
Step 5: Give Verbal Feedback
Now we need to create some actions for when the voice command matches the test. First, we will tell the user which color the program thinks it has found a match for.
Speak Block Do Your Thing
- Click on the TextTo Speech object in the left menu
- Drag a Speak.Message block onto the stage
Type What You Want it to Say
- Click on the Text block in the built-in section of the left menu
- Drag a basic blank text block onto the stage
- Type in the phrase you want it to say
Assemble the Parts
- Plug the filled-in text block onto the Speak.Message block
- Plug the assembled Speak.Messageblock into the Then slot
Now, when the program detects a match with the voice command, the program will speak the phrase you just
typed. Get creative if you want:
- Right said Fred, It's red
- Boo hoo hoo, it's blue
- Green, really? That's not your color dude.
Step 6: Set the Canvas Color
Now, we will finally change the color of the canvas to match the spoken command.
Set Canvas Background Color
- Click on the Canvas object in the left menu
- Drag the SetCanvasBackgroundColorTo block to the stage
Select a Color Swatch
- Click on the Color object in the Built-in section of the left menu
- Drag a color swatch onto the stage
Assemble the Parts
- Plug the color-swatch into the SetBackgroundColor block
- Plug the assembled block into the Then slot of the If-then statement (below the speak block)
Lather, Rinse, Repeat
This is probably a good time to test how the program works. Load it onto your Android device and test it before you duplicate the commands 10 times.
Now that you know the basic code works, duplicate the test-and-actions for each color that you want to be able to select.
You have just created the basic framework for the program. When the program detects a match for the voice command it will speak the designated phrase, then change the color of the canvas to a specified color. You can also specify individual RGB and alpha values, so you could create a command like, more-blue and less-red. You could also create a command to set a random color, have the colors pulse and fade or cycle through the rainbow.
Step 7: Catching No-Match-Found Errors
But what if the voice command does not find a match - you misspoke, or sneezed instead? That's what the final Else statement is for. When all the other tests fail, the program executes the action in this else statement. You create this statement just like you did the previous statements (except there is no test is needed).
- Plug a text field into a SpeakMessage block and plug that into the final else slot.
- Tell the user that, "Oops, I don't know what you are trying to say - please try again."
You are almost done. There are only a few more steps to take now.
Step 8: Starting Voice Recognition Manually
Once the voice command has been tested and the appropriate actions have been taken, the program stops listening for more commands. There are a lot of ways around this, but most are complicated for beginners. So we will stick with something simple - touch the screen to make the program start listening again.
- Click the Canvas object in the left menu
- Drag the whenCanvasTouchDown block onto the stage (as a separate object, not inside any other block)
- Click the SpeechRecognizer object in the left menu
- Drag a callSpeechRecognizer.GetText block onto stage and plug it into the whenCanvasTouchDown block
Now, whenever the screen is touched the program will begin listening for a voice command.
Step 9: What the Full Program Looks Like
You are done - you have just created a voice controlled Andoid app that turns your old phone or tablet into a mood-light. If you are having any trouble getting it to work, download the full-sized version of the image in this step. That image shows the entire program plus a few extras.
But as you can see, the entire program is really just
- a starting call
- a series of tests and actions
- then a restart.
This program just scratches the surface of what you can do with MIT App Inventor. There are lots more commands, and even the commands that we used in this project have options we have not explored. Take this basic program and build on it to make your own customized mood-light, status-board or display panel.
Step 10: Extending and Expanding
Okay, let's do one more thing, just for fun. Instead of just changing the color of the screen, let's show a photograph. You can also display videos, web pages or text messages. Play around and have fun.
- Change back to Designer mode by clicking the button in the upper right corner of the screen
- Click on Canvas in the Left-hand menu
- Also click on Canvas in the Components menu (second panel from right)
- This will bring up the Properties panel for the Canvas
- In the Properties panel (far right) click on Background Image
- Load a photo using the pop-up dialog box
- Switch back to Blocks mode
- Add an extra if-then statement
- Add a test for the picture command
- Add a setBackgroundImageTo block to the decision tree - use the name of the photo
- Also add a setBackgroundImageTo the restart Touchdown block - set the name to "none"
And boom, now you can load photos as well as change the color. You might want to play around with the image size. Or try loading in a video object. You can draw shapes on the canvas, or create animations. You can display numbers, words, graphs - or make several canvases for multiple items.
You can also create programs to access your phone's built-in sensors. You can connect to other applications on your phone, connect to the web and pull information from there, and connect to other devices using Bluetooth or WiFi.
Or use the incredibly handy IFTT to get Alexa or other assistant to set your mood board, or control all your old devices..
You've just started with MIT App Inventor, but you can see how easy and powerful it is to use. So go exploring and create your own display board.
Step 11: Cases and Coverings
So that was a lot of fun, programming a voice activated application. But it still looks like the same old phone or tablet - kind of boring. Why not add a covering or case to device to make it look really custom made. There's only a few considerations to take into account:
If you cover the screen the material has to:
- Have enough holes to allow your finger to touch the screen
- Or be conductive enough to transmit your touch to the tablet surface
Good options are mesh fabric or lace. They both change the look of the phone, but allow your skin to contact the screen. Some thin papers and Mylar type plastics let enough electricity through to register as a touch.
- If you put a frame around it, be sure to leave enough room for the power cord.
- Be sure the device is mounted securely if you place it on the wall. It might be old and outdated, but it's still working - so don't break it now that you know how to program it.
But this is an entirely different subject, worth and complete class and Instructable all by itself. And that's a great way to get new visitors back into your makerspace. So be sure to tell them all to "Come back for the second part of the class."
Happy making, and be excellent to each other.
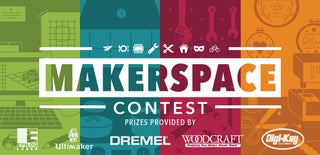
Participated in the
Makerspace Contest 2017