Introduction: WarGames WOPR Computer LED Effect
This instructable is dedicated to imitating the lighting effects shown on the WOPR computer from the 1983 movie WarGames (effect starts at 0:20 in the video). Using an Arduino Uno, 220 Ohm resistors, and some 5mm LEDs, you too can achieve this effect! Let's get started.
Step 1: Setting Up the Breadboard
Firstly, we need to set up out breadboard.
Materials you will need if you want to replicate the exact setup I have here:
Arduino Uno Board
Breadboard with ground row
3 Red LEDs, 5mm
3 Yellow LEDs, 5mm
6 220 Ohm resistors
7 Jumper wires
I connected the cathodes (short ends) of all the LEDs to the ground row of the breadboard, which in turn I connected to one of the GND pins on the Arduino board. This way, I can ground all the LEDs at once.
Next, I connected the anodes (long ends) of the LEDs to the resistors, one for each LED. The orientation of the resistors does not matter.
Finally, I connected the other end of each resistor to 6 Arduino pins (in my case, I used 2-7) using jumper wires.
After the board is set up, it should look like the attached photos.
Step 2: Arduino Code
Now its time to code! I have attached a full copy of the code with included comments that hopefully explain what each of the lines do. You just have to copy the code, connect your Arduino, and upload.
const int led1 = 7; //Initializing led pin numbers as integers<br>const int led2 = 6; const int led3 = 5; const int led4 = 4; const int led5 = 3; const int led6 = 2; int led1state = 0; //Initializing the state of each led, changed randomly later int led2state = 0; int led3state = 0; int led4state = 0; int led5state = 0; int led6state = 0; int blinkDelay = 0; //Initializing an integer for the delay between blinks void setup() { pinMode(led1, OUTPUT); //Setting the pins associated with the ledX variables to outputs
pinMode(led2, OUTPUT); pinMode(led3, OUTPUT); pinMode(led4, OUTPUT); pinMode(led5, OUTPUT); pinMode(led6, OUTPUT); }
void loop() { //This block repeats infinitely led1state = random(0, 2); //The random(min, max) method returns a random number between led2state = random(0, 2); //the included min (inclusive) and max (exclusive) values. led3state = random(0, 2); //This block of code sets each led pin to a random number led4state = random(0, 2); //between 0 and 1, thus choosing whether it outputs hi or lo. led5state = random(0, 2); led6state = random(0, 2); blinkDelay = random(250, 2000); //Randomizes the delay between the blinks, between 250 and 2000ms digitalWrite(led1, led1state); //This block sets the output pins to whatever random state they are assigned digitalWrite(led2, led2state); digitalWrite(led3, led3state); digitalWrite(led4, led4state); digitalWrite(led5, led5state); digitalWrite(led6, led6state); delay(blinkDelay); //Waits a random amount of time before repeating again }
Step 3: Finished Product
You're done! Bask in the millions of calculations that your mini WOPR is making every second. Here's a video of what it should look like.
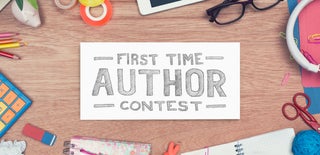
Participated in the
First Time Author Contest 2018