Introduction: A Robot That Tells If an Electronics Vendor Is Cheating You or Not
Last year, me and my dad went to a newly opened robotics/electronics store very close to where we live. As I entered there, It was full of electronics, serovs, sensors, Raspberry pis, and Arduinos. The next day, we went to the same store and bought many senors and 3 Arduinos worth about 150 USD. As soon as I went home, none other products worked except the Arduino Mega. We were scammed for about 130 USD which is a lot.
So, I decided to create a robot that can test about 12 sensors and 2 motors(still working on the servo) and can determine if the product is faulty.
Let's get straight into it!
Supplies
1. Arduino UNO
2. 2.4" TFT touchscreen display
3. couple of jumper wires
4. sensors and motors so that you can test them (here I used - Motion sensor, MQ6 gas sensor, Sound sensor, potentiometer and water level sensor)
Step 1: How It Works.....
I have programmed an Arduino to create and interactive UI with a TFT display. As we know, the TFT display has only one free pin i.e. the A5 pin. (if you use the UNO). So any sensor that uses Analog pins to read data are compatible with this setup...
In the UI, you can change the desktop background color, and you can open the app which was developed for Arduino by me to test sensors.
On opening the app, you can see 2 options, INPUT and OUTPUT. so if you click Input, It shows 4 sensors (It is compatible with 12, but i'm yet to program it as i have exams approaching....no time :( . Anyone is free to edit the code...)
And it can also check if the servo motor works of you connect it in the slot.
have a look :
Step 2: Uploading the Code
The code is a 600 line program that I meticulously coded in about 50 hours. It does have a few bugs so plz amend it and send it to me in my mail (hiyer2006@gmail.com).
Upload the code and connect the TFT shield to the Arduino. I recommend you to play around with the UI so that you get a better hang of it.
The code:
#include <Adafruit_GFX.h> #include <Adafruit_TFTLCD.h> #include <TouchScreen.h> #include <Fonts/FreeMonoBoldOblique12pt7b.h> #define LCD_CS A3 #define LCD_CD A2 #define LCD_WR A1 #define LCD_RD A0 #define LCD_RESET A4 #define BLACK 0x0000 #define BLUE 0x001F #define NAVY 0x000F #define RED 0xF800 #define GREEN 0x07E0 #define CYAN 0x07FF #define MAGENTA 0xF81F #define YELLOW 0xFFE0 #define WHITE 0xFFFF #define LIGHTGREY 0xC618 #define PURPLE 0x780F #define OLIVE 0x7BE0 #define Selection #define YP A1 #define XM A2 #define YM 7 #define XP 6 #define TS_MINX 940 #define TS_MINY 160 #define TS_MAXX 160 #define TS_MAXY 970 TouchScreen ts = TouchScreen(XP, YP, XM, YM, 300); Adafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RESET); #define BOXSIZE 40 #define PENRADIUS 3 #define MINPRESSURE 10 #define MAXPRESSURE 1000 int sound; char state = 6; int touch = 0; int color = BLACK; int redir = 0; int ota; void setup() { Serial.begin(9600); tft.reset(); tft.setFont(&FreeMonoBoldOblique12pt7b); tft.begin(0x9325); tft.setRotation(45); tft.setTextSize(0.5); tft.fillScreen(WHITE); tft.setCursor(60,160); tft.setTextColor(BLACK); tft.print("Swipe to log in"); } void loop() { float sensorVoltage; float sensorValue; TSPoint p = ts.getPoint(); pinMode(XM, OUTPUT); pinMode(YP, OUTPUT); if (p.z > MINPRESSURE && p.z < MAXPRESSURE) { p.x = map(p.x, TS_MINX, TS_MAXX, tft.width(), 0); p.y = map(p.y, TS_MINY, TS_MAXY, tft.height(), 0); Serial.print("("); Serial.print(p.x); Serial.print(", "); Serial.print(p.y); Serial.println(")"); if (p.x > 240 && p.x < 300) { //setings touch if (p.y > 130 && p.y < 240) { touch = 2; } } if (p.x > 240 && p.x < 270) { //home if (p.y > 20 && p.y < 40) { state = 6; } } if (p.x > 240 && p.x < 270) { //back settings if (p.y > 20 && p.y < 40) { touch = 1; } } ///////////////////////////////////////////////////// if (p.x > 160 && p.x < 190) { //about if (p.y > 12 && p.y < 70) { touch = 10; } } if (p.x > 240 && p.x < 270) { //peripherals if (p.y > 20 && p.y < 40) { touch = 1; } } if (p.x > 240 && p.x < 270) { //log out if (p.y > 20 && p.y < 40) { touch = 1; } } if (p.x > 40 && p.x < 80) { //personalisation if (p.y > 15 && p.y < 110) { touch = 11; } } //////////////////////////////////////////////// if (p.x > 150 && p.x < 200) { //about if (p.y > 40 && p.y < 70) { redir = 1; } } if (p.x > 150 && p.x < 200) { //about if (p.y > 80 && p.y < 120) { redir = 2; } } if (p.x > 150 && p.x < 200) { //about if (p.y > 120 && p.y < 160) { redir = 3; } } if (p.x > 150 && p.x < 200) { //about if (p.y > 170 && p.y < 210) { redir = 4; } } if (p.x > 90 && p.x < 140) { //about if (p.y > 40 && p.y < 70) { redir = 5; } } if (p.x > 90 && p.x < 140) { //about if (p.y > 80 && p.y < 120) { redir = 6; } } if (p.x > 90 && p.x < 140) { //about if (p.y > 120 && p.y < 160) { redir = 7; } } if (p.x > 150 && p.x < 200) { //about if (p.y > 170 && p.y < 210) { redir = 8; } } if (p.x > 150 && p.x < 200) { //about if (p.y > 170 && p.y < 210) { redir = 9; } } if (p.x > 150 && p.x < 200) { //about if (p.y > 170 && p.y < 210) { redir = 10; } } if (p.x > 150 && p.x < 200) { //about if (p.y > 170 && p.y < 210) { redir = 11; } } if (p.x > 150 && p.x < 200) { //about if (p.y > 170 && p.y < 210) { redir = 12; } } /////////////////////////////////////////////// if (p.x > 100 && p.x < 220) { //Sensor test App if (p.y > 20 && p.y < 100) { touch = 26; } } ///////////////////////////////////// if (p.x > 200 && p.x < 240) { //Sensor test App if (p.y > 100 && p.y < 160) { sound = 1; } } if (p.x > 100 && p.x < 220) { //Sensor test App if (p.y > 20 && p.y < 100) { touch = 26; } } if (p.x > 100 && p.x < 220) { //Sensor test App if (p.y > 20 && p.y < 100) { touch = 26; } } if (p.x > 100 && p.x < 220) { //Sensor test App if (p.y > 20 && p.y < 100) { touch = 26; } } if (touch == 1 && state == 6) { tft.setRotation(0); tft.fillScreen(color); sound = 0; tft.fillRect(200,180,80,140,WHITE); tft.drawRect(201,181,81,141,BLACK); tft.fillRect(80,30,100,100,CYAN); tft.drawRect(81,31,101,101,BLACK); tft.fillRect(10,30,50,100,NAVY); tft.drawRect(11,31,51,101,BLACK); tft.fillRect(10,180,30,120,PURPLE); tft.drawRect(11,181,31,121,BLACK); tft.fillRect(45,180,30,120,BLUE); tft.drawRect(46,181,31,121,BLACK); tft.fillRect(80,180,30,120,CYAN); tft.drawRect(81,181,31,121,BLACK); tft.fillRect(115,180,30,120,GREEN); tft.drawRect(116,181,31,121,BLACK); tft.fillRect(150,180,30,120,YELLOW); tft.drawRect(151,181,31,121,BLACK); tft.fillTriangle(210,30,210,50,220,40,WHITE); tft.fillRect(200,35,12,12,WHITE); tft.drawTriangle(210,30,210,50,220,40,BLACK); tft.drawRect(200,35,12,12,BLACK); tft.drawCircle(210,40,14,WHITE); //tft.fillTriange(a1,b1,a2,b2,c1,c2,RED); tft.setRotation(45); tft.setCursor(200,20); tft.print("Settings"); tft.fillRoundRect(45,75,75,75,4,WHITE); tft.drawRoundRect(45,75,75,75,4,BLACK); tft.fillRect(75,75,15,30,BLACK); tft.fillRect(75,120,15,30,BLACK); tft.fillRect(90,105,30,15,BLACK); tft.fillRect(45,105,30,15,BLACK); state = 7; touch = 0; ota = 0; } if (touch == 2) { tft.fillScreen(WHITE); tft.setRotation(0); tft.fillTriangle(210,30,210,50,220,40,WHITE); tft.fillRect(200,35,12,12,WHITE); tft.drawTriangle(210,30,210,50,220,40,BLACK); tft.drawRect(200,35,12,12,BLACK); tft.drawCircle(210,40,14,BLACK); tft.setRotation(45); tft.fillTriangle(270,40,270,20,260,30,BLACK); tft.drawLine(300,30,270,30,BLACK); tft.setCursor(20,200); tft.print("personalisation"); tft.setCursor(20,170); tft.print("log out"); tft.setCursor(20,140); tft.print("peripherals"); tft.setCursor(20,110); tft.print("About"); touch = 1; state = 2; } if (touch == 10 && state == 2){ tft.fillScreen(WHITE); tft.setCursor(10,160); tft.setTextColor(BLACK); tft.print("Created and developed"); tft.setCursor(100,190); tft.print("by :"); tft.setCursor(60,220); tft.print("A teen from India"); tft.setRotation(0); tft.fillTriangle(210,30,210,50,220,40,WHITE); tft.fillRect(200,35,12,12,WHITE); tft.drawTriangle(210,30,210,50,220,40,BLACK); tft.drawRect(200,35,12,12,BLACK); tft.drawCircle(210,40,14,BLACK); tft.setRotation(45); tft.fillTriangle(270,40,270,20,260,30,BLACK); tft.drawLine(300,30,270,30,BLACK); state = 6; } if (touch == 11 && state == 2){ tft.fillScreen(WHITE); tft.setCursor(60,40); tft.print("Pick a"); tft.setCursor(40,60); tft.print("background color"); tft.fillRect(60,180,45,45,RED); tft.fillRect(120,180,45,45,YELLOW); tft.fillRect(180,180,45,45,BLUE); tft.fillRect(240,180,45,45,GREEN); tft.fillRect(60,130,45,45,MAGENTA); tft.fillRect(120,130,45,45,NAVY); tft.fillRect(180,130,45,45,LIGHTGREY); tft.fillRect(240,130,45,45,CYAN); tft.fillRect(60,80,45,45,WHITE); tft.drawRect(60,80,45,45,BLACK); tft.fillRect(120,80,45,45,BLACK); tft.fillRect(180,80,45,45,OLIVE); tft.fillRect(240,80,45,45,PURPLE); tft.setRotation(0); tft.fillTriangle(210,30,210,50,220,40,WHITE); tft.fillRect(200,35,12,12,WHITE); tft.drawTriangle(210,30,210,50,220,40,BLACK); tft.drawRect(200,35,12,12,BLACK); tft.drawCircle(210,40,14,BLACK); tft.setRotation(45); tft.fillTriangle(270,40,270,20,260,30,BLACK); tft.drawLine(300,30,270,30,BLACK); state = 6; if (redir == 1){ color = WHITE; } if (redir == 2){ color = BLACK; } if (redir == 3){ color = OLIVE; } if (redir == 4){ color = PURPLE; } if (redir == 5){ color = MAGENTA; } if (redir == 6){ color = NAVY; } if (redir == 7){ color = LIGHTGREY; } if (redir == 8){ color = CYAN; } if (redir == 9){ color = RED; } if (redir == 10){ color = YELLOW; } if (redir == 11){ color = BLUE; } if (redir == 12){ color = GREEN; } } if (touch == 26){ ota = 1; tft.fillScreen(WHITE); tft.setRotation(0); tft.fillTriangle(210,30,210,50,220,40,WHITE); tft.fillRect(200,35,12,12,WHITE); tft.drawTriangle(210,30,210,50,220,40,BLACK); tft.drawRect(200,35,12,12,BLACK); tft.drawCircle(210,40,14,BLACK); tft.setRotation(45); tft.fillTriangle(270,40,270,20,260,30,BLACK); tft.drawLine(300,30,270,30,BLACK); tft.setRotation(1); tft.setCursor(30,80); tft.print("Input"); tft.setCursor(30,150); tft.print("Output"); tft.drawRect(25,130,90,30,BLACK); tft.drawRect(25,60,90,30,BLACK); if (p.x > 190 && p.x < 220){ ////////////INPUT if (p.y > 30 && p.y < 90){ tft.setCursor(150,220); tft.print("Water level"); tft.setCursor(150,170); tft.print("Gas level"); tft.setCursor(150,120); tft.print("Distance "); tft.setCursor(150,70); tft.print("Sound"); touch = 1; state = 2; } } } while (sound == 1){ tft.fillScreen(WHITE); sensorValue = analogRead(A5); sensorVoltage = sensorValue; tft.setCursor(60,160); tft.print(sensorVoltage); Serial.println("Sound level = "); Serial.println(sensorVoltage); delay(1000); if (sound == 0){ break; } } } }<br>
Step 3: Libraries Required :
- Adafruit_GFX.h
- Adafruit_TFTLCD.h
- TouchScreen.h
Github links for the same :
https://github.com/adafruit/Adafruit-GFX-Library
Step 4: The Connections
You need 6 jumper wires. 3 males and 3 females. solder them directly to the Arduino board like this:
Step 5: Slap It All Together
Tuck the wires in to the arduino (refer image), Use some glue gun if needed...
Then Attach the TFT shield then test your Sensors.
Thank you for scrolling all the way down. Make sure to vote for me if you felt this project was unique and helpful
Happy making and beware of Electronics scammers 😂
Step 6: Further Improvements:
- More sensor compatibility
- Servo motor code had to be finished
- Another app that can interface with Bluetooth modules so that we can control a Bluetooth powered robot with it.
- Bug fixes
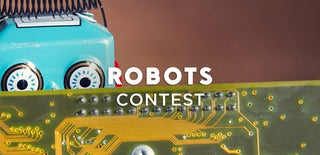
Participated in the
Robots Contest