Introduction: App for Mobile Control LED(Arduino+Bluetooth Module)
Hello,
In this i will explain how to code for Android app in Android Studio.
For Arduino code and circuit, refer...
https://www.instructables.com/id/Mobile-Control-LEDAndroidBluetooth
Step 1: Creating New Project(LED Control)
Download Android Studio, install and open it
- create new project.-name LED Control
- Select minimum SDK to target
- create blank activity named list
Step 2: Design the List Activity
Now design list activity as shown in fig. above.
Or directly copy the code in Text from the file provided in last.
Step 3: Coding for List Activity
package com.example.kulwinder.ledcontrol;
import android.bluetooth.BluetoothAdapter; import android.bluetooth.BluetoothDevice; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.ArrayAdapter; import android.widget.Button; import android.widget.ListView; import android.widget.TextView; import android.widget.Toast; import java.util.ArrayList; import java.util.Set;
public class list extends AppCompatActivity { ListView list; Button paired;
private BluetoothAdapter adapter = null;//Represents the local device Bluetooth adapter private Set paireddevices; public static String EXTRA_ADDRESS = "device_address";//use to store MAC address of connected device
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_list);
//Calling the widgets paired = (Button) findViewById(R.id.paired); list = (ListView) findViewById(R.id.listView);
//if the device has bluetooth adapter = BluetoothAdapter.getDefaultAdapter();
if (adapter == null) { //if no adapter/device availabe Toast.makeText(getApplicationContext(), "No Bluetooth Device available", Toast.LENGTH_LONG).show(); finish(); } else if (!adapter.isEnabled())//if adapter is available { //Ask to the user for turning on the bluetooth Intent i1 = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);//intent use for to atrat new activity startActivityForResult(i1, 1); }
paired.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { pairedDevicesList();//call function } });
}
//function private void pairedDevicesList() { /*Once you have the local adapter, you can get a set of BluetoothDevice objects representing all paired devices with getBondedDevices() */ paireddevices = adapter.getBondedDevices(); ArrayList alist = new ArrayList();
if (paireddevices.size() > 0) //if paired devices are there { for (BluetoothDevice bt : paireddevices) { alist.add(bt.getName() + "\n" + bt.getAddress()); //Get the device name and the address } } else { Toast.makeText(getApplicationContext(), "No Paired Bluetooth Devices Found.", Toast.LENGTH_LONG).show(); } // to show a vertical list of scrollable items we will use a ListView which has data populated using an Adapter /* The ArrayAdapter requires a declaration of the type of the item to be converted to a View (a String in this case) and then accepts three arguments: context (activity instance), XML item layout, and the array of data. Note that we've chosen simple_list_item_1.xml which is a simple TextView as the layout for each of the items. */ final ArrayAdapter adapter1 = new ArrayAdapter(this, android.R.layout.simple_list_item_1, alist); list.setAdapter(adapter1); list.setOnItemClickListener(myListClickListener); //Method called when the device from the list is clicked }
private AdapterView.OnItemClickListener myListClickListener = new AdapterView.OnItemClickListener() { public void onItemClick(AdapterView
Step 4: Design Led Activity
Procedure for new empty Activity shown in fig.
Design led activity as shown in fig.
Step 5: Code Led Activity
package com.example.kulwinder.ledcontrol;
import android.app.ProgressDialog; import android.bluetooth.BluetoothAdapter; import android.bluetooth.BluetoothDevice; import android.bluetooth.BluetoothSocket; import android.content.Intent; import android.os.AsyncTask; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.SeekBar; import android.widget.TextView; import android.widget.Toast; import java.io.IOException;import java.util.UUID;
public class LED extends AppCompatActivity {
Button on, off, discon, exit; SeekBar brightness; TextView value; String address=null; private ProgressDialog progress;//Progress bars are used to show progress of a task. BluetoothAdapter adapter=null;//Represents the local device Bluetooth adapter BluetoothSocket socket=null;//socket is an endpoint for communication between two machine private boolean isBtConnected=false;
static final UUID myuuid=UUID.fromString("00001101-0000-1000-8000-00805F9B34FB"); /*the uuid is used for uniquely identifying information. it identifies a particular service provided by a Bluetooth Device */ @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_led);//this view our activity
Intent newint = getIntent(); /*for receivig data d=from another activity....Here we recieve address of selected paired device from list activity */ address = newint.getStringExtra(list.EXTRA_ADDRESS);
//calling the widgets on= (Button) findViewById(R.id.onbt); off= (Button) findViewById(R.id.offbt); discon= (Button) findViewById(R.id.disconnectbt); exit= (Button) findViewById(R.id.backbt); value= (TextView) findViewById(R.id.value); brightness= (SeekBar) findViewById(R.id.brightsb);
new connectbluetooth().execute();//calling function to connect
//functions of buttons on CLICK on.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { onled();//callinf onled function on click of on button } });
off.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { offled(); } });
discon.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { disconnect(); } });
exit.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { System.exit(0);
brightness.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() { @Override public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) { if(fromUser==true)//(fromUser)=callback that notifies client when the progress level has been changed { value.setText(String.valueOf(progress));//print seekbar value to text field-value try { socket.getOutputStream().write(String.valueOf(progress).getBytes()); //send value of progress to bluetooth socket and socket send it to bluetooth module } catch(IOException e) {}
} }
@Override public void onStartTrackingTouch(SeekBar seekBar) { }
@Override public void onStopTrackingTouch(SeekBar seekBar) { }}); }
private void message(String a) { Toast.makeText(getApplicationContext(),a, Toast.LENGTH_LONG).show(); //display the message on screen for 3.5 sec }
private void onled() { if (socket!=null) { try { socket.getOutputStream().write("on".toString().getBytes()); //send "on" to bluetooth module } catch (IOException e) { message("Error"); //send string s="Error"to message function } } }
private void offled() { if (socket!=null) { try { socket.getOutputStream().write("off".toString().getBytes()); //send "off" to bluetooth module } catch (IOException e) { message("Error"); //send string s="Error"to message function } } }
private void disconnect() { if (socket!=null) { try { socket.close(); } catch (IOException e) { message("Error"); //send string s="Error"to message function } } finish(); }
//An asynchronous task is defined by a computation that runs on a background thread and whose result is published on the UI thread. private class connectbluetooth extends AsyncTask { private boolean ConnectSuccess = true;
@Override protected void onPreExecute() { progress = ProgressDialog.show(LED.this, "Connecting...", "Please wait!!!"); //show a progress of connection }
@Override protected Void doInBackground(Void... devices) //while the progress dialog is shown, the connection is done in background { try { if (socket == null || !isBtConnected)//when bluetooth device not connected { adapter = BluetoothAdapter.getDefaultAdapter();//get the mobile bluetooth device BluetoothDevice device = adapter.getRemoteDevice(address);//connects to the device's address and checks if it's available socket = device.createInsecureRfcommSocketToServiceRecord(myuuid);//create a RFCOMM (SPP) connection BluetoothAdapter.getDefaultAdapter().cancelDiscovery();//it ask for permission...so add it socket.connect();//start connection } } catch (IOException e) { ConnectSuccess = false;//if the connection try failed, you can check the exception here } return null; } @Override protected void onPostExecute(Void result) //after the doInBackground, it checks if everything went fine { super.onPostExecute(result);
if (!ConnectSuccess) { message("Connection Failed. Try again."); finish(); } else { message("Connected."); isBtConnected = true; } progress.dismiss();//close progress bar i.e. working } }
}
Step 6: Creating Apk
Now create apk for the app->
for this go to Build->Build apk
now install the app and enjoy controlling led through mobile.
you can download whole Android file from the link:
https://drive.google.com/open?id=0B4eY-jcXDOueX0M5WFktZHdLQ2s
If any queries feel free to write.
thank you!
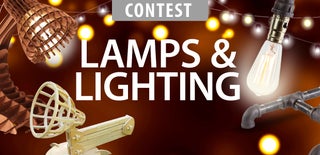
Participated in the
Lamps and Lighting Contest 2016
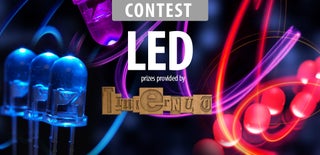
Participated in the
LED Contest