Introduction: Arduino 30 Sec. Countdown
Hello, everyone! I hope you are doing fantabulous! Today, I want to share with you my project (which is free - besides, perhaps, buying the parts) about how to make the Arduino countdown from 30. This, again, is an open source project, so... little to no cost for you! And who doesn't want that?
Anyways, on to the project! Let's answer some of your burning questions you might have:
- Why is this project so important? Or at least special?
Well, the reason why this is so important/special is because there isn't THAT well of documentation of counting down on a display (especially, the seven segment display - more on that later).
- What's the use of this project?
The use of this project can be used on YOUR very own project. If you mean things, like, applications, then here is the following: microwaves (though I wouldn't suggest it with the Arduino), claw machines, ovens (still no Arduino), etc.
- Can I make it count for more or less?
Absolutely! You can make the counter count up or countdown. Just make sure you view the code down below.
Without further delay (heh, get it?), let's get started.
Supplies
Hmm... okay. So, you want to know about the tools and supplies used, yes? Okay. Well, I think the tools are a bit common sense (and so is the cable to the Arduino), so I won't really go over that.
It also depends on what you have already. For instance, you might already have wires and not need wire strippers. But, I will list some of the supplies you need for the actual wiring part. The rest is up to you.
- Arduino Mega 2560 (as the title implies) or similar - yes, even an Arduino Uno will suffice.
- 4 digit seven segment display
- Some wires (about 20 for me)
- MAX7219 IC
- 10 micro Farad (uF) electrolytic capacitor.
- "104" or 0.1 uF ceramic capacitor
- And a 10k-100k ohm resistor
- *Optional* - Grounding strap (for static electricity). I am very proud of it, so keep that in mind.
And I am not at your house, so I really don't care where you get this stuff from (possibly) online. Unless, of course, you already have this stuff. If you want a link, then comment below.
Step 1: One of Two Things
One of two things, you can either buy it how it comes (preassembled) on Amazon, or you can make it yourself. (Here is the Amazon link: Preassembled MAX7219 ). I, personally (as an electronic enthusiast), prefer to build it myself. But that's more of a pride thing. Also, it's the only things I had lying around. Until Christmas, of course.
Step 2: Building It Yourself
Okay, if you are looking at this tutorial, I am assuming you want to build it. If you want it preassembled, then skip the next couple of steps (just jump straight into the code, in other words). To build yourself, there is a few steps we have to follow: taking off the MAX7219 of the dot matrix display and hooking it up to the Arduino/seven segment display. Let's begin!
Step 3: Taking Off the IC
I have added the video as a visual guide. It's too easy, you just need a little bit of prying force. Now, when I say "a little bit" I MEAN IT. Please, please, please, DON'T damage the IC/chip. Just use a little - A LITTLE - bit of force. I cannot stress how important it is that if you don't use a little bit of force (assuming you would use a lot), you WILL break the pins on MAX7219.
It is VERY delicate! Anyway, if you have that done, let's move on...
Step 4: Adding It to the Breadboard
The video in the introduction is VERY helpful, by the way. I will be referencing it. I just assumed you watched. Anyway, as I have struggled in the video, you just slap that thing on a breadboard. No, not literally. So, once you put that IC on the breadboard, you can follow my wiring diagram.
If you are not new to this kind of stuff, then, you can follow my schematic. The reason why I am putting both on here is for both beginners and intermediate people. If you are at the advanced level, I am sorry to tell you, you WILL cringe.
Anyway, allow me to explain myself on my wiring stuff. The latter has +5V on a step-down buck converter. The GND is, well, GND for both the Arduino and +5V buck converter. They are daisy chained. *An important note - put the notch/divot forward (meaning, pin 1 should face "up").*
The rest is pretty simple: you hook up the segment according to the left and right side of the chip. (Which, by the way, is numbered 1-24). One starting at the top left of the chip (which is on the left of the divot). The segments go on the right side of the chip and go to each segment "location." Such as, A goes to, you guessed it, Seg A. This is because each thing called a segment is put into place on the actual display.
Seg A is the first segment or "line" and is in the middle of D1. You can research more on that theory, if you want, as I am not going to go over that. I am not THAT good at explaining the theory behind it.
Anyway, here's the trick: the digital pins go on the left side, BUT they go from DIG0-DIG7! So which one do you choose? The easiest (and what makes the most sense to me) is the DIG0, DIG1, DIG2, and DIG3. In other words, hook it up in that combination, and you should be fine. The rest is history.
Hey, by the way... I also forgot to mention that +5V. Yeah, that's pretty important. You HAVE TO USE +5V or there could be catastrophic consequences. Heath my warning...
Oh, yes, one more thing: I think you have to use the digital pins on some Arduinos. Don't quote me on that 'cause I am not that sure. I have an Arduino Mega 2560 rev3, so... I can't speak for that.
Step 5: Coding
Ah! My favorite step! Okay, as you may already know (because of today's title), this is supposed to count down from 30. But, how does it do that, and how does it know whether it counted down in time? Okay, calm down. I'll explain the rest in pieces in the next step.
#include <LedControl.h>
//Austin Harris
//December 23, 2022
//Timer Countdown Thing - V1.0.3
#define DataIn 2
#define LOAD 4
#define CLK 3
LedControl lc = LedControl(2,4,3,1); // DataIn, CLK, LOAD, # of module(s)
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
lc.shutdown(0,false);
/* Set the brightness to a medium values: 0-15 */
lc.setIntensity(0,15);
/* and clear the display */
lc.clearDisplay(0);
lc.setDigit(0,1,3,false);
lc.setDigit(0,0,0, false);
delay(1000);
}
void loop() {
// put your main code here, to run repeatedly:
lc.setDigit(0,1,2,false);
for(int i2 = 9; i2 >= 0; i2--){
lc.setDigit(0,0,i2,false);
delay(1000);
if(i2 == 0){
lc.setDigit(0,1,1,false);
for(int i3 = 9; i3 >= 0; i3--){
lc.setDigit(0,0,i3,false);
delay(1000);
if(i3 == 0){
lc.setDigit(0, 1, 0, false);
for(int i4 = 9; i4 >= 0; i4--){
lc.setDigit(0, 0, i4, false);
delay(1000);
while(i4== 0){
Serial.println("Congrats! You did it!");
delay(1000);
}
}
}
}
}
}
digitalWrite(24, HIGH);
digitalWrite(32, HIGH);
}
Step 6: Code Explaination
Like, honestly, if you could come up with some better code (or when I do more research), then please share it! I would love to hear your comments about the code! (Unless it's negative). Anyways, here we go:
#include <LedControl.h>
This is the inclusion of the LedControl library (which can be found on GitHub ). For beginners, this means that another set of code is taken into this code. Making it easier on the user to control the seven seg.
#define DataIn 2
#define LOAD 4
#define CLK 3
These "#define"s are a set of code to make the DataIn (terrible syntax, I know), LOAD, and CLK variables. Not gonna teach that here.
LedControl lc = LedControl(2,4,3,1); // DataIn, CLK, LOAD, # of module(s)
Now, the module is not named by convention. Meaning, I didn't name it a cool name or anything. It's just lc. That's boring, but I WAS in a rush. So I didn't care about the name. Anyway, the LedControl library "sets up" the pins, or makes the variables actually connect with the Arduino.
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
lc.shutdown(0,false);
/* Set the brightness to a medium values: 0-15 */
lc.setIntensity(0,15);
/* and clear the display */
lc.clearDisplay(0);
lc.setDigit(0,1,3,false);
lc.setDigit(0,0,0, false);
delay(1000);
}
This is the setup. I don't really know how to explain that, but, basically, it runs the code on "setup" or startup. Anyhow, the Serial.begin sets up the Serial Monitor (for beginners, look it up). The lc.shutdown, ironically, is false. Meaning, that the display is actually on. Why? Because it is not set as true. It's just on, okay? The intensity is the brightness of the display. It can be set to 0-15 (on the right side).
The clear display part "resets" or clears the display. The set digit part is set to "3" toward the middle of the display. In other words, it is at the 3rd position (second digit) to the right. The "0" is on the far right (the first digit).
Then, we give a delay of 1 second, because it pauses every second. *It's supposed to countdown from 30 seconds, in total*.
void loop() {
// put your main code here, to run repeatedly:
lc.setDigit(0,1,2,false);
for(int i2 = 9; i2 >= 0; i2--){
lc.setDigit(0,0,i2,false);
delay(1000);
if(i2 == 0){
lc.setDigit(0,1,1,false);
for(int i3 = 9; i3 >= 0; i3--){
lc.setDigit(0,0,i3,false);
delay(1000);
if(i3 == 0){
lc.setDigit(0, 1, 0, false);
for(int i4 = 9; i4 >= 0; i4--){
lc.setDigit(0, 0, i4, false);
delay(1000);
while(i4== 0){
Serial.println("Congrats! You did it!");
delay(1000);
}
}
}
}
}
}
}
The loop, ah, the most tricky part. This is where I had the most struggle, because I was like, "Do I want to use a certain amount of for loops? Or what about if statements? What about a while loop?" Well, guess what... I use all of that! So, for the loop, I just used a bunch of the same thing really. So, call me repetitive all you want, but it made the most sense to me.
For the first line of code in the loop, I just made it say, "You know what? Display a 2 since it's been a second." Oh, also, the false on the set digit just says whether or not it should display a hexadecimal character, but we want numbers, gosh darn it! Anyway, since we are counting down in seconds, we display that as a 2 and set the next digit (the previous 0) to 9. We do that using a for loop, because I am too lazy to type everything all out.
It's, also, highly inefficient to not use a for loop, in this case, 'cause we are counting. Remember? Anyway, since you now know what that for loop does, it just pretty much repeats itself.
Now, with the if statements, I just pretty much said that if the for loop digit is EQUAL TO (not assigned) 0, then we display a "1" on the far right. That's because it has been 10 seconds. Remember, we are counting in seconds! Which is why I added a 1 second (1000 milliseconds) delay. Then it repeats, to count down from 9 to 0. Again that's ten seconds. Here's a visualization, 9, 8, 7, 6, 5, 4, 3, 2, 1, and 0. Notice, that's 10.
On top of that, you probably counted with a second pause in between. That's the end goal here. See? It's pretty repetitive.
For the last thing: we say, "while the last for loop variable is EQUAL TO 0..." we party! We congratulate ourselves. Alright, so maybe you want something more useful. Like...
while(i4 == 0){
digitalWrite(LED, HIGH);
lc.shutdown(0, true);
delay(1000);
digitalWrite(LED, LOW);
lc.shutdown(0, false);
delay(1000);
}
That does two things (assuming you fixed it to use an LED): 1. It "blinks" the display or turns it on and off at 1 sec delay, and 2. It turns on the hypothetical (which I tested and it works, by the way) LED. I'll stir the pot even more! You can even add a piezo buzzer! :O Yes, you can do what you want with the while loop. As I have said in the video, I am using this for a claw machine. So that's where I will put some useful code there. The rest is history.
Step 7: The Finale, or Should I Say the Final Countdown?
Well, hopefully you have learned something new! I hope you enjoyed this tutorial, and I know this isn't a real step, but I thought I would wish you a great day! If you have any questions or need to tell me something, feel free to comment down below. See 'ya!
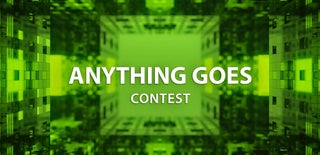
Participated in the
Anything Goes Contest