Introduction: Arduino Based Visual Music Display
Ever seen those cool displays using little rows of LEDs that bounce up and down in time to the music? Or those bars that do the same? Ever wanted to make one? Now you can! I had this idea recently, and realized that I could make it quite easily. It's a simple and fun project, with a cool result.
Featured!! Wow!! Thanks everyone!
Step 1: Parts Needed:
Here's a list of parts that you need. Happily, there's not too many!
You'll need:
- An Arduino UNO or compatible
- Breadboard and wires
- 6 red (Or other color) LEDs
- 6 220 ohm resitors
2 breadboard audio jacks (Or some other way to connect audio in/out to your arduino)
That's all! Let's get building!
Step 2: Wire It All Up:
Time to build it! You can either follow the Fritzing drawing, or my instructions.
- Wire your Arduino's power to your breadboard's power strip. (Don't forget ground!)
- Plug the audio jacks in next to each other, and wire the audio out pins to each other. Wire both ground pins to ground.
- Connect the Analog pin A0 to the audio out on the jacks.
- Place the 6 LEDs in a row on the breadboard, connect the ground sides to ground through the 220 ohm resistors.
- Wire the Arduino's digital pins 8-13 to the 6 LEDs on the breadboard.
You're done!
Step 3: The Code:
Time to program the Arduino! Luckily, the program is a simple as the wiring. Feel free to fiddle around with the code!
There are two different programs, with slightly different code, that achieve different looks. The first one looks less bouncy and flickers/fades the LEDs more. The second is bouncier and doesn't fade the LEDs as much. You can either download them via the .zip files, or copy/past from here.
Here's the code for the first version, AudioVisualDisplay:
/* * AudioVisualDisplay, a program by Jacob Field, written for the arduino * UNO. This program uses 6 LEDs connected to pins 8, 9, 10, 11, 12, * and 13 as a visual volume display for audio. The audio to be displayed * is fed into the Arduino via the analog pin A0. I recommend using a * second audio jack so you can hear the audio being played. ;) Feel free * to use this code, or any part of it, in your own personal projects, * or distribute it freely as you see fit, just give credit where credit * is due. */ byte ledValue; int signalIn; void setup() { //Set all the LED pins as outputs pinMode(8, OUTPUT); pinMode(9, OUTPUT); pinMode(10, OUTPUT); pinMode(11, OUTPUT); pinMode(12, OUTPUT); pinMode(13, OUTPUT); } void loop() { signalIn = analogRead(A0); //Read the analog value and store it ledValue = map(signalIn, 0, 100, 0, 6); //Convert the 10 bit value down to only 7 different values switch(ledValue){ case 0: //If the value is 0, set all the LEDs low digitalWrite(8, LOW); digitalWrite(9, LOW); digitalWrite(10, LOW); digitalWrite(11, LOW); digitalWrite(12, LOW); digitalWrite(13, LOW); break; case 1: //If the value is 1, set the first LED high digitalWrite(8, HIGH); digitalWrite(9, LOW); digitalWrite(10, LOW); digitalWrite(11, LOW); digitalWrite(12, LOW); digitalWrite(13, LOW); break; case 2: //If the value is 2, set the first 2 LEDs high digitalWrite(8, HIGH); digitalWrite(9, HIGH); digitalWrite(10, LOW); digitalWrite(11, LOW); digitalWrite(12, LOW); digitalWrite(13, LOW); break; case 3: //If the value is 3, set the first 3 LEDs high digitalWrite(8, HIGH); digitalWrite(9, HIGH); digitalWrite(10, HIGH); digitalWrite(11, LOW); digitalWrite(12, LOW); digitalWrite(13, LOW); break; case 4: //If the value is 4, set the first 4 LEDs high digitalWrite(8, HIGH); digitalWrite(9, HIGH); digitalWrite(10, HIGH); digitalWrite(11, HIGH); digitalWrite(12, LOW); digitalWrite(13, LOW); break; case 5: //If the value is 5, set the first 5 LEDs high digitalWrite(8, HIGH); digitalWrite(9, HIGH); digitalWrite(10, HIGH); digitalWrite(11, HIGH); digitalWrite(12, HIGH); digitalWrite(13, LOW); break; case 6: //If the value is 6, set all the LEDs high digitalWrite(8, HIGH); digitalWrite(9, HIGH); digitalWrite(10, HIGH); digitalWrite(11, HIGH); digitalWrite(12, HIGH); digitalWrite(13, HIGH); break; } }
Here's the code for the second version, AudioVisualDisplayRev2:
/* * AudioVisualDisplay, Revision 2, a program by Jacob Field, written for the * arduino UNO. This version uses a different algorithm to analyze the * signal, creating a diffrent look This program uses 6 LEDs connected to pins * 8, 9, 10, 11, 12, and 13 as a visual volume display for audio. The * audio to be displayed is fed into the Arduino via the analog pin A0. * I recommend using a second audio jack so you can hear the audio being * played. ;) Feel free to use this code, or any part of it, in your own * personal projects, or distribute it freely as you see fit, just give * credit where credit is due. */ byte ledValue; int valueHigh; int signalIn; void setup() { //Set all the LED pins as outputs pinMode(8, OUTPUT); pinMode(9, OUTPUT); pinMode(10, OUTPUT); pinMode(11, OUTPUT); pinMode(12, OUTPUT); pinMode(13, OUTPUT); } void loop() { signalIn = analogRead(A0); //Read the analog value and store it valueHigh = valueHigh - 5; //Subtract 5 from valueHigh so that it goes down over time if(signalIn >= valueHigh){ //If the value coming from the audio jack is higher than the previous value of valueHigh: valueHigh = signalIn; //Set valueHigh to thge value coming in from the audio jack } ledValue = map(valueHigh, 0, 100, 0, 6); //Convert the 10 bit value down to only 7 different values switch(ledValue){ case 0: //If the value is 0, set all the LEDs low digitalWrite(8, LOW); digitalWrite(9, LOW); digitalWrite(10, LOW); digitalWrite(11, LOW); digitalWrite(12, LOW); digitalWrite(13, LOW); break; case 1: //If the value is 1, set the first LED high digitalWrite(8, HIGH); digitalWrite(9, LOW); digitalWrite(10, LOW); digitalWrite(11, LOW); digitalWrite(12, LOW); digitalWrite(13, LOW); break; case 2: //If the value is 2, set the first 2 LEDs high digitalWrite(8, HIGH); digitalWrite(9, HIGH); digitalWrite(10, LOW); digitalWrite(11, LOW); digitalWrite(12, LOW); digitalWrite(13, LOW); break; case 3: //If the value is 3, set the first 3 LEDs high digitalWrite(8, HIGH); digitalWrite(9, HIGH); digitalWrite(10, HIGH); digitalWrite(11, LOW); digitalWrite(12, LOW); digitalWrite(13, LOW); break; case 4: //If the value is 4, set the first 4 LEDs high digitalWrite(8, HIGH); digitalWrite(9, HIGH); digitalWrite(10, HIGH); digitalWrite(11, HIGH); digitalWrite(12, LOW); digitalWrite(13, LOW); break; case 5: //If the value is 5, set the first 5 LEDs high digitalWrite(8, HIGH); digitalWrite(9, HIGH); digitalWrite(10, HIGH); digitalWrite(11, HIGH); digitalWrite(12, HIGH); digitalWrite(13, LOW); break; case 6: //If the value is 6, set all the LEDs high digitalWrite(8, HIGH); digitalWrite(9, HIGH); digitalWrite(10, HIGH); digitalWrite(11, HIGH); digitalWrite(12, HIGH); digitalWrite(13, HIGH); break; } }
Step 4: Finished!
You're finished! Now you can test it out!
The code isn't perfect, some improvements could be made, but for now it gets the job done. You can fiddle with some of the values to suite it more to your liking. Hopefully this was a fun little project for you! Feel free to suggest improvements in the comments!
Here's one suggested improvement by lucanletter
"I also changed something that I can highly recommend: I wanted to have both audio-channels (left and right) visualized but I also wanted to have the stereo sound on my jukebox so I added a 1N4148 diode to one pin of the audiojack and tadaa it works just fine."
The diode makes sure that the audio input from one channel doesn't interfere with the audio from the other channel, as seen in the schematic he drew (Last picture).
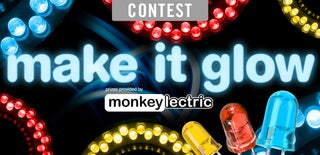
Participated in the
Make It Glow! Contest
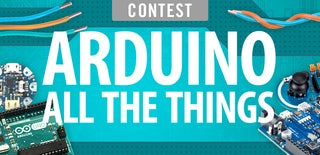
Participated in the
Arduino All The Things! Contest