Introduction: Arduino Decimal Counter With 7 Segment Display
Using an arduino uno and a seven segment display we are going to make a very simple and basic circuit that counts from 0 up to 9 and repeats until you turn it off.
Step 1: Stuff That We'll Need
- Arduino UNO
- 220 Ω resistor
- 7-segment display (I am using a common anode one)
- 10 jumper wires
Step 2: Connecting the Resistor With the Display
First of all take the 7-segment display and put it in the breadboard. Now connect the resistor with the power bus on your breadboard and with the two COM pins of the display using two of the jumper wires as shown in the picture above.
Step 3: Connecting the Arduino With the Display
In this step we are going to connect the Arduino with our display using digital pins 12 to 6. To begin connect pin C of the display with digital 12 on the Arduino. Then continue by connecting pin D with digital 11, E with 10, G with 9, F with 8, A with 7 and lastly B with 6. So to sum up we have:
- C to 12
- D to 11
- E to 10
- G to 9
- F to 8
- A to 7
- B to 6
After that continue by connecting the resistor with the 5V output on the Arduino's board.
Step 4: The Code
Now that we have finished building our little circuit it's time to write the code, so connect your Arduino to your computer, open up the Arduino IDE and get ready. First of all we are going to declare some variables to hold the values of digital pins that we will use and one that we will use to put a time delay between the numbers. After that we will declare 10 functions, one for each number, from which we will send voltage to the correct set of pins to light the proper LEDs for each digit we want. Lastly in the setup() function we are going to initialise the digital pins as output and in the loop() function we will call the functions that we declared earlier in the proper order with the time delay between them. I am also going to paste the code below so feel free to copy it and use it for your project.
int c = 12;
int d = 11;
int e = 10;
int g = 9;
int f = 8;
int a = 7;
int b = 6;
int chrono = 1000;
void zero(){
digitalWrite(a, LOW);
digitalWrite(b, LOW);
digitalWrite(c, LOW);
digitalWrite(d, LOW);
digitalWrite(e, LOW);
digitalWrite(f, LOW);
digitalWrite(g, HIGH);
}
void one(){
digitalWrite(c, LOW);
digitalWrite(d, HIGH);
digitalWrite(e, HIGH);
digitalWrite(g, HIGH);
digitalWrite(f, HIGH);
digitalWrite(a, HIGH);
digitalWrite(b, LOW);
}
void two(){
digitalWrite(c, HIGH);
digitalWrite(d, LOW);
digitalWrite(e, LOW);
digitalWrite(g, LOW);
digitalWrite(f, HIGH);
digitalWrite(a, LOW);
digitalWrite(b, LOW);
}
void three(){
digitalWrite(c, LOW);
digitalWrite(d, LOW);
digitalWrite(e, HIGH);
digitalWrite(g, LOW);
digitalWrite(f, HIGH);
digitalWrite(a, LOW);
digitalWrite(b, LOW);
}
void four(){
digitalWrite(c, LOW);
digitalWrite(d, HIGH);
digitalWrite(e, HIGH);
digitalWrite(g, LOW);
digitalWrite(f, LOW);
digitalWrite(a, HIGH);
digitalWrite(b, LOW);
}
void five(){
digitalWrite(a, LOW);
digitalWrite(b, HIGH);
digitalWrite(c, LOW);
digitalWrite(d, LOW);
digitalWrite(e, HIGH);
digitalWrite(f, LOW);
digitalWrite(g, LOW);
}
void six(){
digitalWrite(a, HIGH);
digitalWrite(b, HIGH);
digitalWrite(c, LOW);
digitalWrite(d, LOW);
digitalWrite(e, LOW);
digitalWrite(f, LOW);
digitalWrite(g, LOW);
}
void seven(){
digitalWrite(a, LOW);
digitalWrite(b, LOW);
digitalWrite(c, LOW);
digitalWrite(d, HIGH);
digitalWrite(e, HIGH);
digitalWrite(f, HIGH);
digitalWrite(g, HIGH);
}
void eight(){
digitalWrite(c, LOW);
digitalWrite(d, LOW);
digitalWrite(e, LOW);
digitalWrite(g, LOW);
digitalWrite(f, LOW);
digitalWrite(a, LOW);
digitalWrite(b, LOW);
}
void nine(){
digitalWrite(a, LOW);
digitalWrite(b, LOW);
digitalWrite(c, LOW);
digitalWrite(d, LOW);
digitalWrite(e, HIGH);
digitalWrite(f, LOW);
digitalWrite(g, LOW);
}
void setup() {
pinMode(c, OUTPUT);
pinMode(d, OUTPUT);
pinMode(e, OUTPUT);
pinMode(g, OUTPUT);
pinMode(f, OUTPUT);
pinMode(a, OUTPUT);
pinMode(b, OUTPUT);
}
void loop() {
zero();
delay(chrono);
one();
delay(chrono);
two();
delay(chrono);
three();
delay(chrono);
four();
delay(chrono);
five();
delay(chrono);
six();
delay(chrono);
seven();
delay(chrono);
eight();
delay(chrono);
nine();
delay(chrono);
}
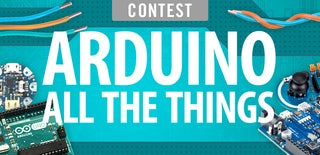
Participated in the
Arduino All The Things! Contest