Introduction: Arduino Light Switch
In this tutorial we are going to construct a very simple light switch using an Arduino Uno, a photoresistor and a little bit of code to turn an LED on and off based on the lighting of your room. So let's get down to business !
Step 1: What We'll Need
Here is a list of all the components that we are going to use in our project:
- 6 jumper wires
- 1 LED
- 1 Arduino Uno
- 1 photoresistor
- 1 220Ω resistor
- 1 10KΩ resistor
Step 2: Giving Power to Our Breadboard
Ok the first step simple is really simple. Using 2 jumper wires connect the 5V pin to the positive power bus on your breadboard and the GND pin to the negative power bus, just as shown in the picture above.
Step 3: Connecting the Resistors
Ok now in this step we are going to wire up the photoresistor with the A0 analog in pin. First of all connect the photoresistor with the positive charge and then put the 10KΩ resistor in series and connect it with the negative charge. Finally connect A0 analog in pin with the photoresistor as shown in the image above.
Step 4: Wiring Up the LED
In this step we are going to add the LED on our circuit. The first thing we want to do is connect the anode of the LED with the 220Ω resistor and then the resistor to digital pin 2 on the board of the Arduino. Lastly connect the LED's cathode to the negative power bus. You should end up with something that looks like the circuit in the image above. That's it, we have finished building the circuit. Now it is time for us to write some code !
Step 5: The Code
Now that we have finished with the construction of our circuit the time has come for us to write the code. So connect the Arduino with your computer and open up the Arduino IDE. First of all we are going to declare some integer variables:
- sensorValue
- sensorHigh
- sensorLow
- led (make this one a constant variable)
Now let sensorValue without a value (it will automatically gain one from our sketch) set sensorHigh equal to 1023 (this will be also changed by our sketch) and sensorLow equal to 0(our sketch will change this one too), finally set led equal to 2. Now in the setup() function set the led pin as an output and declare a while loop that we are going to use to calibrate the sensor, add the millis() function as a parameter to the loop and 500 as parameter to the function(mills() will check the current time. millis() reports how long an Arduino has been running). Inside the loop read the value of A0(using analogRead()) and store it in sensorValue, then write an if statement to see if sensorValue is greater than sensorHigh, if that's the case set sensorHigh equal to sensorValue and close the statement. Then add a second if statement to check if sensorValue is less than sensorLow, if it returns true set sensorLow equal to sensorValue, close the if statement and finally the while loop. To write the final part of our code let's move on to the loop() function. First of all once again read the value of A0 and store it in sensorValue, then write a conditional to check if sensorValue equals sensorHigh, in which case set the led pin to high (using digitalWrite()), else check if sensorValue is equal to sensorLow, in which case set the led pin to low, then if the above statements return false set the led pin to Low. I will also upload the .ino file here so fell free to download it and use it for your project
Attachments
Step 6: The End
There is only one thing left to do. Test our project. Upload the sketch, connect to power, wait for a couple of seconds and then close the lights. You should now see the LED glowing, turn on the lights again and the LED should be turned off. I suggest you have only one light source near the circuit, like a lamp, for it to work better.
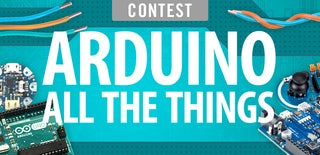
Participated in the
Arduino All The Things! Contest