Introduction: Arduino Doorbell
Today, I will be showing you how to make a doorbell using an Arduino. This doorbell plays a random tune from a library of songs. You can customize your doorbell and add even more songs. Install it outside your bedroom, classroom, office, or even your front door!
Supplies
- Arduino Uno (most other types will do)
- Computer with Arduino IDE for programming
- USB-A to USB-B cable for programming Arduino
- Breadboard
- 4x jumper wires
- Pushbutton or other switch
- 10 kOhm Resistor
- Passive buzzer (i.e. piezoelectric speaker)
- For permanent installation:
- 9V DC Power Supply or 9V battery to power Arduino
- 2x long wires for wiring button outside door
Step 1: Hardware Setup
First, we will set up the hardware. We will start with the passive buzzer (i.e. speaker). We want to connect the positive end of the passive buzzer (denoted by "+" sign) to digital pin 8 on the Arduino. We will connect the other end of the passize buzzer to ground.
Next, we will install the pushbutton for the doorbell. We will use a 10 kOhm external pulldown resistor for the switch, so there is no floating voltage or unstable state on the input to the Arduino. By using the pulldown resistor, the Arduino reads the voltage as 0V when the button is not pressed and 5V when the button is pressed. For more information on pullup or pulldown resistors, you can read this article: https://circuitdigest.com/tutorial/pull-up-and-pull-down-resistor.
We will connect one side of the pushbutton to 5V. The other side of the pushbutton will be connected to ground through a 10 kOhm pulldown resistor. Remember: pushbuttons are connected horizontally internally. They are only connected vertically when pressed. More information is provided in this article: https://circuitdigest.com/electronic-circuits/push-button-led-circuit/.
Attachments
Step 2: Software Setup
All of the code is attached. Below are the descriptions of each of the functions with snapshots of the code.
setup:
In the setup() function, we want to configure the digital pins for our button and our speaker. We want to configure pin 2 as an input for our button, and we want to configure pin 8 as an output for our speaker.
We also want to "seed" our random number generator for randomly selecting a tune when someone rings our doorbell. Seeding our random number generator means feeding it a random input. We will seed our random number generator with the voltage value on analog input 0. Since nothing is connected to this input, there will be a "random", fluctuating voltage on this pin providing our random number generator with many different values. This ensures that we will have a different order of song selections for our doorbell. For more information on the Arduino random() function, go here: https://www.arduino.cc/reference/en/language/functions/random-numbers/random/.
#include "pitches.h" #include "songs.h" #define BUTTON_PIN 2 #define SPEAKER_PIN 8 /* set up function */ void setup() { // enable input/output pins pinMode(BUTTON_PIN, INPUT); pinMode(SPEAKER_PIN, OUTPUT); // seed random() function so that we get a different order randomSeed(analogRead(0)); }
loop:
In our loop() function, we will continually check to see if the button is pressed (digital pin 2 is high). If the pin is high, we wait 50 ms and check again to make sure that it is still high. This ensures that the button is pressed and it was not stray noise on the digital input pin causing a false positive.
Once we have confirmed that the button was pressed, we use our random number generator to select one of the 5 songs using a switch statement. The data for these songs is stored in "songs.h" and the pitch information is stored in "pitches.h". Once we choose a song, we pass this information into the function play_song().
/* main while loop function */ void loop() { // check to see if button is pressed if (digitalRead(BUTTON_PIN) == HIGH) { // delay 50 ms to make sure it's still pressed // avoids any stray misreadings delay(50); if(digitalRead(BUTTON_PIN) == HIGH) { // randomly choose a song int song_choice = random(5); // select which song to play switch (song_choice) { case 0: play_song(haircutLength,haircut,haircutDurations,haircutTempo); break; case 1: play_song(marioLength,mario,marioDurations,marioTempo); break; case 2: play_song(miiLength,mii,miiDurations,miiTempo); break; case 3: play_song(hpLength,hp,hpDurations,hpTempo); break; case 4: play_song(takeonmeLength,takeonme,takeonmeDurations,takeonmeTempo); break; default: play_song(miiLength,mii,miiDurations,miiTempo); break; } } } }
play_song:
play_song() takes 4 arguments: an integer number of notes in the song, an integer array of the pitches in the melody, an integer array of the duration, and an integer tempo for that particular song. You must specify each of these for every song you wish to play. For more information on how to use the Arduino tone functions, you can look at this tutorial: https://www.arduino.cc/en/Tutorial/ToneMelody. I added some functionality on top of this tutorial for dotted notes. If a value in the note duration array is negative, that means that it is a dotted note (the length is 1.5 times greater).
/* plays the song */ void play_song(int num_notes, int melody[], int noteDurations[], int tempo) { // step through and play all of the notes for (int i=0; i<num_notes; i++) { int duration = 0; // calculate the duration of each note if (noteDurations[i] > 0) { duration = tempo / noteDurations[i]; } // if it's a negative number, means dotted note // increases the duration by half for dotted notes else if (noteDurations[i] < 0) { duration = tempo / abs(noteDurations[i]) * 1.5; } tone(SPEAKER_PIN, melody[i], duration); // to distinguish the notes, set a minimum time between them. // the note's duration + 30% seems to work well: int pauseBetweenNotes = duration * 1.30; delay(pauseBetweenNotes); // stop the tone playing: noTone(SPEAKER_PIN); } }
Sample of songs.h:
Below is a sample of one of the songs in "songs.h". The notes are macros defined in "pitches.h". The numbers correspond to the frequencies of the notes in hertz (Hz). The duration of the notes are defined as: 1 = whole note, 2 = half note, 4 = quarter note, 8 =eighth note, -4 = dotted quarter note, etc. The length is the total number of notes in the song. The tempo is a divider for the speed of the song (a higher number means a slower tempo). You will have to play around with this number until you get a tempo that you like.
/* harry potter */ int hp[] = { NOTE_D4, NOTE_G4, NOTE_AS4, NOTE_A4, NOTE_G4, NOTE_D5, NOTE_C5, NOTE_A4, NOTE_G4, NOTE_AS4, NOTE_A4, NOTE_F4, NOTE_GS4, NOTE_D4 }; int hpDurations[] = { 4, -4, 8, 4, 2, 4, -2, -2, -4, 8, 4, 2, 4, 1 }; int hpLength = 14; int hpTempo = 1050;
Step 3: Tweaks!
Add more songs! Follow the format shown in "songs.h" and use the tutorial for help: https://www.arduino.cc/en/Tutorial/ToneMelody. For every new song you add, remember to add a new case to the switch statement and increase the maximum number that can be generated by your random() function. Happy coding!
Step 4: More Projects
For more projects, visit these links:
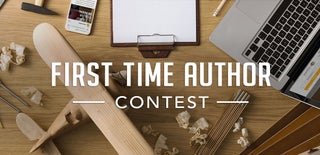
Participated in the
First Time Author Contest