Introduction: Arduino Virtual Data (in This Example Clock) With Processing
Hi! In the previous project, i showed you how to control shift register with computer. Now i´m just sharing what i have learned about it: You don´t need to by parts to test your project! Only bad side is that you have to run processing all the time when you want this project working, and you have to keep Arduino attached to your computer. So this is very good way to try example clock without buying any module! You only need your Arduino, laptop, Arduino IDE and Processing. It's also good to have something to visualize time: I'm using my HC595N shift register to drive 8 leds and i use binary(8-bit) to visualize 0-255 with 8 led. Let's start!
Step 1: Writing Processing Code
Now we need code, that sends time to Arduino. First we need to include serial library. Second thing is creating the serial port with Serial port; command and then we can set up the port in setup() void: port = new Serial(this, "COM3", 9600); You have to put "COMX". X is Arduino com port number.
This is pretty simple code, and you can now write to our port hour(): port.write(hour());
It's easier to read full code from here:
import processing.serial.*;
Serial port;
void setup() {
port = new Serial(this, "COM3", 9600);
}
void draw() {
port.write(hour());
delay(2000);
}
====================
And that's all! Simple, right? Now let's move to the next step!
Step 2: Arduino Code
Now we have code, that sends data to Arduino. Now we have to create something to receive all that information!
first thing we do, is int value = 0;
Then we have to open serial communication in setup() void, with command Serial.begin(9600);
Then we have to listen incoming data with
if(Serial.available() > 0) { (if incoming data )
value = Serial.read(); (value = data we got from serial)
}
If you want to do something with that value, just use value as variable. I´m using shift register in this example, so i can do it like that:
Full code is here:
======================
int dataPin = 3; //dataPin = 3
int clockPin = 4; //clockPin = 4
int latchPin = 5; //latchPin = 5
int value = 0;
void setup() {
Serial.begin(9600);
pinMode(dataPin, OUTPUT); //set dataPin to output
pinMode(latchPin, OUTPUT); //set latchPin to output
pinMode(clockPin, OUTPUT); //set clockPin to output
}
void loop() {
if(Serial.available() > 0) {
value = Serial.read();
}
//do something with the value (value = hour from computer)
digitalWrite(latchPin, LOW); //put latch off so we can't see any flickering during shifting data
shiftOut(dataPin, clockPin, MSBFIRST, value); //send data to shift register
digitalWrite(latchPin, HIGH); //put latch back on so we can see the leds
}
=====================
Step 3: Hardware + Reading Binary
Now we have to connect the shift register:
Latch -> D5, Clock -> D4, Data -> D3, VCC -> 5V, GND -> GND, and so on.
If you need more information about shift registers, you can check it here:
https://www.arduino.cc/en/Tutorial/ShiftOut
Now we have everything ready, so we can put Arduino code to Arduino, and run processing app. You should see some leds on, and i´m telling now what it means:
You have eight leds: first is 1, second is 2, third is 4,fourth is 8, fifth is 16, sixth is 32, seventh is 64, and the last one is 128. You have to plus all leds that is on. I have first, third and fourth led on, so clock is 13(1PM)! Easy right?
Thanks for reading! What i should do next?
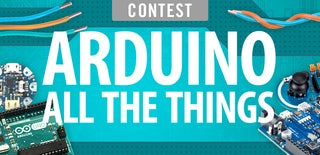
Participated in the
Arduino All The Things! Contest