Introduction: Arduino Basics - Part 1
Hi ! This is the first part of my guides for arduino and it's for beginners , so it might be boring for more advanced users ! In this part I'll give a step by step coding guide on how to work with simple electronic parts (I'll use only LEDs but these codes work for any other thing that works simply and gathers no information (like a direct drive motor))
Coding is as important as making (for example , a robot) . A great code makes the most use out of a great device , but a bad code simply ruins a great device . For example , you've made a great line following robot , but without a nice code , it won't be the best robot !
For this instructables , you will need :
your microcontroller board (Arduino. I used uno but you can use any other board (unless it has different codes)
3x LEDs or higher .
wires . the most important part ! :D
(optional) a breadboard (to learn how to use breadboards too)
the wire that connects your arduino to your computer
a computer with Arduino IDE installed
a power source (I'll explain later)
Step 1: Arduino
Why Arduino ? Why not another type of board ?
because of its simple programming language and small size and wide range of purposes , you can use it anywhere !
usually other boards have hard programming languages and larger size . However they are more powerful and more useful . But for a beginner or a simple project , arduino is the best .
How can I power the arduino without burning it ?
With a normal 9v battery (although it might not be powerful enough to turn on other stuff attached to the board) or the usb cable (which is short and has to be attached to a computer) or a 9v adapter (0.6 A is enough) . The best choice is the adapter : longer than the usb cable and gives enough energy to keep multiple stuff on , and way cheaper than using batteries then throwing them in the trash can . Also better for our planet as disposing batteries has some problems AND factories have to make lots and lots of batteries . But with an adapter , only an adapter is made and is used for a long time ! However always be ready for using batteries as you cant use your adapter in a robotics match
How do I delete the codes I've written in the "flash" part of the memory ??
When you give a new program to the board , the previous one is removed from the "flash" . EEPROM has to be deleted with a code and ram is deleted when you take the power source OR press the reset button (pressing the reset will just restart the code , and if your "ram" is information gained from sensors , then again the ram will get the info )
Which board model is the best ?
Uno is great for beginners and for using in a normal robot . It has 31.5 kilobytes (~248,000 bits) of space you can write codes in , 16 Mhz processing power , 3 ground pins ; 14 digital (PWM) pins and some other pins , and is small (5x7 cm) . For more advanced users , Mega and Due are great as they have more space , more pins , more processing power and etc etc etc . For small and lightweight projects , micro and mini and pro mini pro micro and etc. One upside of uno is that if the main IC has a problem you can easily take it out and buy another one and place it there (it has a socket ) But for other boards you must de-solder the IC and buy another one and get arduino bootloader and etc. (ATmega 328 with Arduino bootloader can be bought from online shops) .
Step 2: Blink . a Very Simple Example .
Blink is one of the examples available in the arduino app . it blinks an led . The LED turns on for 1 second , is off for 1 second . But this continues because of the loop code .
The first code to learn is void . It is only and only used TWICE in each program (maybe even ONCE) . It is not a code itself but it is used before setup and loop .
void setup () {
some basic info
}
this is how you use setup . it is used to give some information to the board . As you can see in the "blink" , it is used to tell the board pinMode(led, OUTPUT) some things . But what is led ? How should I know , asks the board .
Before any code , we gave pin 13 a NAME , and that name is "led" . You can name it anything , for example pin13 . or LED1 or MrLED or anything , but then , any place you wrote led , you should delete led and write the new name . Used for working with multiple things .
the next code we must learn is void loop :
void loop () {
codes,codes,codes
}
As its name suggests , it LOOPS . It repeats the action . If we would not use LOOP , then the led will just turn on for 1 second and turn off . You can use reset so it does the action again , but it wont look like blinking but it will just be on for 1 second and off for multiple seconds and on for 1 second , which isn't BLINKing .
The next codes we must learn are digitalWrite( ,HIGH) , digitalWrite( ,LOW) and delay( ) .
#1 - digitalwrite high
In the blink example we see :
digitalWrite(led,HIGH);
this code gives the LED energy and the LED turns on . Then delay is used to determine how long the led should be on (or off)
#2 - digitalwrite low
Same as the above example but we write LOW instead of HIGH , which turns the LED off . Again we use delay to determine how long it should stay on .
#3 - delay
Delay determines how long should actions be done . It is used simply
delay( );
The amount of time is written in the brackets . 1 is 1/1000 of a second so 1000 is a second .
Step 3: LEDs
This step is for beginners , if you know about LEDs then skip this step !
LED is the short form of LIGHT EMITTING DIODE . Its a diode that makes light when current gets past it . LEDs , look similar but are different . Their first difference is how many colors they can make . This is a bit new . The next difference is how much voltage they can survive (max) . Some are 5 or maybe less (like mine) and better ones can even survive 10 and higher .
What is important about LEDs and makes them different than other lamps is that they are DIODES which means current can get past from only one side (they have specific sides , one + and one -) . The - side is called cathode and + is called anode . TO understand which is which , there are 3 ways :
#1 - Usually the "leg" of + is longer . However you must cut them to make them equal , so you can use easier . But how do you understand which is which , later ??
#2 - There are 2 pieces inside the LED , one is bigger . The bigger one is - and the smaller one is +
#3 - (might not apply to EVERY SINGLE LED IN THE WORLD) The negative (-) side is not round like the other parts of the led .
Step 4: Not an Instructables on How to Blink
Blinking isn't all you can do with an LED . You can use the codes to send morse code signals ! I've attached an IDE file . It has a code that sends SOS through morse with an led on pin 7 .
(it's named "sketch_aug13b")
Another work is to use multiple leds ! I'll write an instructables about multi-tasking later , the code I've currently attached (3 led blink) works with 3 leds : led on pin 7 blinks , then 8 blinks then 10 blinks then again 7,8,10 and over and over again .
Please , do not just use the codes without trying to write yourself ! This is about learning and the attached files are just for checking how well your code is . My code is not the best code and the timings are not certainly the best , many people can make even better codes .
you can also make the 3 led blink with a breadboard . Use pictures as guide . It is good to learn how to work with breadboards .
Step 5: Troubleshooting
No LEDs are making light .
make sure they're on the right pin and check the wires . Make sure the wires are connected . If that doesn't help , make sure you LED is still alive . Maybe it has burnt . sometimes leds burn with no reason . If it's black on the inside and / or smells , it has burnt .
Can't compile my program .
Make sure you haven't missed any of the IMPORTANT codes , such as void setup , void loop and etc . If that doesn't help , make sure you haven't missed ; or () or {} . If the error resists , ask . (I'm not professional at it (yet) so it's better to ask in the "ask" part of instructables or in the official arduino forum)
Don't see the attached files .
You cannot see them with ios devices , and they might as well not appear on the android app . Visit the website with a computer .
Thanks for reading , and , I hope it was useful ! I'll put more guides soon !
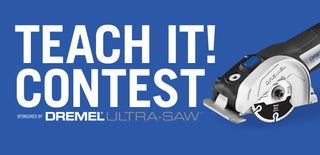
Participated in the
Teach It! Contest Sponsored by Dremel
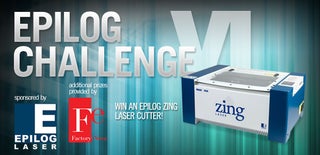
Participated in the
Epilog Challenge VI
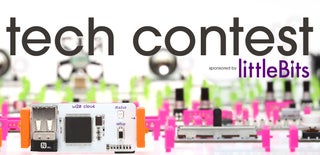
Participated in the
Tech Contest