Introduction: Automated Greenhouse With Arduino Mega
Here's what High School teachers do during Covid-19 when teaching from home. :)
Background - I was planning on automating a greenhouse at my school as a project. When we were shut down I thought building a greenhouse would be a great thing to do as I was thinking of one anyway. Since I have historically had an issue with remembering to water plants I knew automation was my only real option. I will post an instructable for the greenhouse but that's another day. This instructable is mostly around the Arduino portion of the greenhouse, though I will talk about other aspects. This system uses rain barrels for water but you can substitute the rain barrel with a standard water supply. You will need power in the greenhouse, I just ran an extension cord but you could install an electrical outlet if you were so inclined.
Basic Requirements:
Able to solder or use quick connectors for electrical connections
Basic tool knowledge, nothing too adventurous in this Instructable
Tools Needed:
A soldering kit or electronics connectors
Pliers
Side Cutters
Small Philips Screwdriver (cross)
Wire Strippers
Supplies
Arduino Mega Board and power supply
Arduino 4 relay module - optional 8 relay module (this is what I used)
Soil Moisture sensor
Ultrasonic Sensor
LCD display - 2 line or 4 line (I used the 2 line 1st then switched to the 4 line)
Irrigation Kit - optional extra irrigation line, T's and Irrigation drippers
Rain Barrel
Water Pump - 500 GPH minimum up to 2000 GPH
6 feet of 18-22g wire - an old ethernet cable can be pulled apart for the wire inside
Step 1: System Planning and Options
Water Supply
The main aspect of keeping your plants alive is adequate watering and sunlight. The sunlight part will depend on the placement of your greenhouse and potential shading, but that's another discussion. Watering can be done with a standard garden hose, however, if you are like me and want automation then you can use a rain barrel system or a switch on a water supply. I used the rain barrel with a pump, but the same thing can be achieved with a water supply switch instead of a pump. The rain barrel requires that you greenhouse has an eavestrough to collect the water and direct it into the rain barrel(s).
I used the rain barrel method and added a 2nd rain barrel to ensure I wouldn't run out of water. So far I have not run out of water. They are interconnected at the base so that both barrels fill and drain together. I have taps on the connection so I can open or close the connection if needed. I initially went with the 500 GPH water pump but found the flow too low for the system I created so I ended up purchasing a high rated water pump and have the original one on a manual switch so I can manually water at any time. I also opted for an 8 relay module though I have only actually used 4 of the relays. I also have an on/off button that is optional for a light as well as a temp/humidity sensor to control my fan and heater.
A couple of things to consider when choosing your water pump/supply. If you run the main water supply above your water source you will need a much stronger pump to bring the water up with enough pressure to run your system. I will probably rerun my water as the pressure is a bit low at the drippers, especially since I have quite a few of them.The other thing to think about is the number of water drippers in your system, the more you have the lower the pressure to all of them. So you will need to adjust the length of time your water pump runs in order to get enough water to the plants.
Arduino Program Overview
Basically the system reads the soil moisture and if the soil gets too dry the system activates the pump to provide water through the irrigation system. The system can also activate a heater or fan by measuring the temperature and then activating a relay as needed. There is also a water level sensor so the pump will not activate if there is not enough water in the rain barrel.
Arduino Schematic without the optional button
https://www.circuito.io/app?components=512,10167,1...
This schematic shows different connections to the Mega board then what I use but it is simple to modify either the code or the circuit to match what I built. The dht11 sensor in the circuit diagram shows a resistor, the one I used has it built in.
Step 2: Arduino Code Explained
Here is the code that I wrote for this system, the file is attached above, called greenhouse.ino. I will explain the variables that you can modify for your own system. In general the only thing you may want to change that is not below is the length of time your water pump runs when it gets activated. The system is currently set for 5 minutes, defined by the pumprun variable, which is set to 300 seconds. The section that activates the water pump uses this variable to both run the pump then to wait the same amount of time to let the water soak in before checking the moisture again. So adjust this variable higher to give the plants a better soak each time the pump runs or lower to provide less water but more often.
There are 4 include files that you will also need to add to your Arduino library.
Wire.h LiquidCrystal_I2C.h NewPing.h dht11.h
All of these include files are available on the Arduino.cc website
The 4 line LCD display is connected on a Mega board at pins 20 (SDA) and 21 (SCL), on a different board you will need to find where SDA and SCL are located. 0x27 is the address of the LCD board, the board you get might have a different address, there is also a program that will poll for the address and display it in the serial monitor in your arduino program.
LiquidCrystal_I2C lcd(0x27,20,21);
The ultrasonic sensor is connected to pins 7 (trigger) and 9 (echo), the low water level in my system is set to 60 CM from the top of the barrel. As the water level drops the distance to the sensor mounted at the top of the barrel will increase.
The dht11 temperature & humidity sensor is connected to pin 18
The following variables are used to control the system, the variable names should be self explanatory. temp means temperature, hum means humidity, etc.
The variable that you need to set are;
lowlevel = 60 is for your low water level as measured from the top of the barrel where your ultrasonic sensor is located and pointed to the top of the water. Set this value to 5 CM less then the lowest level your pump can run. This will prevent your pump running dry, which can burn out your pump.
distance = 70 is the initial setting for the ultrasonic sensor, this is set to above the low water level so the pump does NOT automatically go on when the system starts
lowtemp = 5 sets the temperature in Celcius (about 42 F) when the heater will turn on
hightemp = 25 (82 F) is when the fan will turn on
highhum = 70 this is there in case you want to do something for high humidity
soilpin = A0 is the pin for the soil sensor analog signal - do not change this
soilValue = 25 is the initial soil moisture value
soillimit = 400 is the dry soil value
soiltimer = 500 is how many times the program loops before the soil is checked (aprox every 5 minutes) We do this so we don't burn out the soil sensor by polling it multiple times a second. I will probably increase this value as I finish adjusting the irrigation system. Checking the soil moisture once an hour should be more than enough.
looptimer = 1000 is to count the number of loops the system is making, I show this value in the LCD display so I can see when things should be happening. The water pump activation is checked every 1000 loops (about 10 minutes)
The next section defines all the constant variables that do not change.
buttonPin = 2 is the pin location for the pushbutton sensor
There are 9 LED lights that are completely optional that light up when certain things happen these are all connected to even numbered pins 28 to 44
There are 8 relays in this system, which can be substituted for the 4 relay unit. relay1 to relay8 are connected to the odd numbered pins 31 to 45.
The relays are setup as follows
relay1 is for the water pump in the rain barrel
relay2 is for the fan
relay3 is for the heater
relay4 is for a future grow light
relay5 is for a 2nd future grow light
relay6 is a spare
relay7 is a spare
relay8 is for a work light, a button is connected to this system that allows this relay to be activated or deactivated by pressing the button.
Attachments
Step 3: Finish the Arduino System
I built the Arduino system in my house and tested all the sensors without having anything attached to the relays. This allowed me to fix any loose connections and make sure all my sensors were working. You can test the soil sensor in a cup of water, the ultrasonic sensor by moving your hand closer and further from the sensor and the temp/humidity by putting it in your hands forming a cup around the sensor and blowing into your hands. The temp & humidity will increase. Even though there are no pumps, fans, lights or heaters hooked up the relays will click on and off and you will see a led light activate when the relay closes.
Once you have connected and tested your Arduino you will then need to decide how to protect it and place it in your greenhouse. I have access to a 3D printer so I designed an enclosure to protect the system. A Tupperware container will do the same thing.
When you bring the system into your greenhouse you want to have your water and electrical close to the Arduino control system. I have mine on a shelf above and beside the rain barrel with a power bar on the 2x4 stud behind it.
You will need to do some minor electrical work on the various components.
Water Pump
Connect your water hose to the pump and drop it in your rain barrel. I used the 5/8 hose connectors to create the exact size of water hose I needed, cutting and feeding the hose through the top of the barrel then connecting the end. Cut the electrical cord to the water pump about 2 feet from the end that plugs into an outlet. Feed the electrical wire up through the lid of the rain barrel after drilling a hole large enough for the wire. Strip about 2" of insulation from the wire to expose the individual wires inside. There should be 3 wires exposed, neutral, power and ground usually coloured white, black and green. Strip the same amount of insulation from the other end of the wire that you cut, the one with the outlet plug attached. Strip and reconnect the white and green wires to each other by either soldering or using electrical connectors. Strip about 1/4" of insulation from each the black wires. Insert the exposed wires into relay1 then tighten the set screws. The example pictures above are using white wire and a single relay but the multiple relay connects exactly the same way. The wires are inserted into the left and centre connections. See the pic above.
Ultrasonic Sensor and Soil Sensor - wire extension
You will need a few feet of wire, like the kind in a network cable. 4 individual wires are needed for the ultrasonic sensor, 2 wires are needed for the soil sensor. There are electrical quick connectors that can be used or soldering to extend the length of the wires so the sensors can reach from the rain barrel or soil sensor location to the arduino system. Drill 2 holes in the top of the rain barrel to fit the ultrasonic sensor into the top of the rain barrel. Insert the soil sensor into the soil that you will be monitoring.
Optional Heater and Fan
Use the same method as used in the water pump to connect a heater or fan to your arduino system relays. Some heaters and fans use a 2 wire electrical connection instead of a 3 wire connection. Since you do not need to feed the wire through anything you can just split out the one wire for the relay connection. See the pic above.
Temperature and Humidity - dht11 sensor and button
These two components do not need to be extended or altered in any way, as long as they are accessible and able to measure the air in the greenhouse.
Step 4: Build Your Irrigation Distribution
Create your irrigation system by cutting and connecting the water line with the drippers. Extra 1/4" irrigation line and drippers are a great idea but not necessary. I do recommend purchasing extra garden hose to 1/4" line converters if you have more than 15 drippers on a line. You will get better pressure and water delivery to your plants if you split your garden hose into 4 distribution points instead of the two that come with the irrigation system I used. Remember to run the main water line (hose line) low to the ground then run the 1/4" line up to your plants and containers for best pressure. The other item I noticed after a couple of weeks is that you need to filter the water coming into your rain barrels for bugs, leaf debris, etc. For me the easiest way was to attach a nylon stocking to the end of the downspout. This will catch the debris while not blocking the water flow and is easy to clean out or change if needed. If you don't filter out the debtis it will eventually clog your lines and drippers. This results in having to try and clean out all your lines and drippers, a major pain in the body part.
I suspect regular cleaning of the lines and drippers is probably necessary, just watch for impeded flow.
Step 5: Changes and Improvements
I needed to change out the soil sensor as the one I used seems to not read consistently. I'm now trying the gikfun capacitive soil sensor. The schematic and code will not need to change for the new sensor so I will post soil moisture values for the code as I figure them out and if they need to be modified. So far the default value in the code is working fine for me.
The Irrigation system was being constricted by the 1/4" tubing. A 5/8" water hose reduced to a 1/4" tube works much better if it is split 4 times instead of the 2 that come with the irrigation system I purchased. Water distribution is much better using 4 distribution points. You could probably use 6 distribution tubes and getter even better flow but the 2 are working for the size of my greenhouse. There are about 10-12 drippers off of each distribution tube in my system now.
The nylon stocking on the downspout is working perfectly. I also wrapped a stocking around the pump itself inside the rain barrel. There have been no clogs in the irrigation system in the past 3 weeks. :)
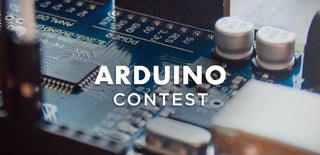
Participated in the
Arduino Contest 2020