Introduction: Control Arduino From Your IOS Device and Your Apple Watch
This Instructable is old and doesn't apply to the new version of Arduino Manager. For an updated Instructable follow this link:
How to Control Arduino R4 WiFi From Your IPhone / Apple Watch / IPad / Mac
This simple instructable is about Arduino Manager, a powerful general purpose iOS App which allows to control any Arduino (or Arduino compatible) board by the means of about 40 different Widgets.
Some of the available Widgets are:
- Display Widget
- LED Widget
- Switch Widget
- Push Button Widget
- Knob Widget
- Slider Widget
Arduino Manager also provides (at an extra cost):
- Code Generator: This add-on allows to generate the basic Arduino code for supporting the Widgets you have chosen.
- PIN Code Generator: Linking widgets to Arduino PINs this add-on dramatically speeds up Arduino code development. In many cases you don't need to write a single line of code.
- Voice Commands: Enables to control most of the Arduino Manager Widgets by the means of voice commands (English only).
Recently, Arduino Manager for iOS has gained Apple Watch support: you can control any Arduino board directly from your wrist, everywhere in the world.
Arduino Manager for iOS is available here: iTunes Store for $7.99. It is also available for Android and Mac OS X (please, note that not all the widgets are available on all the platforms).
The video below shows what we are going to build:
More information, documentation, video tutorials, libraries and examples on Arduino Manager are available here:
Step 1: What You Need
For this project you need:
- An iOS device which supports Bluetooth 4.0 (aka Bluetooth Low Energy).
- An Arduino UNO (or any compatible board)
- Arduino IDE (you can download it here: Arduino IDE)
- An Adafruit nRF8001 Bluefruit LE Breakout (View Product)
- A breadboard
- A few male-male jumpers
- 1 RED LED
- 1 YELLOW LED
- 2 330Ω resistors (0.25w)
Step 2: External Circuit
First we need to build the additional electronic circuit which has 3 components:
- Adafruit nRF8001 Bluefruit LE for connecting iOS devices to Arduino via Bluetooth 4.0 (BLE)
- One Yellow LED that we are going to turn on and off directly from our wrist
- One Green LED of which we are going to control the light intensity directly from our wrist
Everything is kept easy just for demonstration purpose. You can easily add a Relay or a power circuit based on a Triac to control lights or motors or whatever (let me know if you are interested in these circuits, I'll add additional instructables).
Images above show the circuit diagram and the how to wire the circuit using the breadboard.
Refer to the following information for wiring the nRF8001 Bluefruit LE.
UNO
- SCK: pin 13
- MISO: pin 12
- MOSI: pin 11
- REQ: pin 10
- RST: pin 9
- RDY: pin 2
MEGA
- SCK: pin 52
- MISO: pin 50
- MOSI: pin 51
- REQ: pin 10
- RST: pin 9
- RDY: pin 2
Step 3: Install Arduino Manager Libraries
In order to make Arduino Manager working with Arduino, you need to install some additional libraries. Fortunately, this step is not too hard because a Library Installer is available for Windows and MAC OS X environment.
If you are working with Linux, please consult the available documentation.
Your Arduino IDE has to be already installed. Please, open it at least once before installing the libraries.
Please, be sure that your Arduino libraries directory doesn't contain the Adafruit_nRF8001 directory. In case you already have installed that library, remove it and restart the Arduino IDE.
Installing Libraries for Windows
- Download the installer from here: Libraries Installer for Windows
- Unzip the downloaded file (IOSControllerLibrariesInstaller.exe.zip)
- Run the installer
- At least select IOSController for Bluefruit Breakout
- Click Install
- Restart Arduino IDE
Installing Libraries for MAC OS X
- Download the installer from here: Libraries Installer for MAC OS X
- Unzip the downloaded file (IOSControllerLibrariesInstaller.app.zip)
- Run the installer
- At least select IOSController for Bluefruit Breakout
- Click Install
- Restart Arduino IDE
Step 4: Upload Arduino Code
Now we are ready to upload the Arduino code for the project.
- The code attached to this page
- Open it with the Arduino IDE
- Select UNO in the Arduino IDE (Tools->Board->UNO)
- Select the USB port where your Arduino board is attached (Tools->Port->...)
- File->Upload
Now your Arduino is ready for receiving commands from your iOS device and from your Apple Watch.
Attachments
Step 5: Learning the Arduino Code (Optional)
The code for supporting Arduino Manager is mainly based on four additional functions:
- doWork - Replaces the loop functions. Here goes your code
- processIncomingMessages - Receives information from the connected iOS device
- processOutgoingMessages - Sends information to the connected iOS device
- doSync - Sends status information to the iOS devices as soon as it connects
Let's see each function.
The doWork function just sends information on the current status (on/off) of the red LED.
void doWork() {
iosController.writeMessage("Red",digitalRead(REDLEDPIN));
}
The processIncomingMessages is called for each message received and has two parameters:
- variable - the name of the widget which is sending data
- value - data associated to the widget. For example, in case if the Switch Widget value is 0 if the switch is off and 1 if it is on.
So, the function turns on and off the red LED according with the position of the switch on the iOS device and with the position of the Slider. Note that the Slider sends a value in the range 0-1023 and the analogWrite accepts values in the range 0-255. The map function transforms a value in one range to the corresponding value in the other range (see Arduino documentation).
void processIncomingMessages(char *variable, char *value) {<br>
if (strcmp(variable,"Red")==0) {
digitalWrite(REDLEDPIN,atoi(value));
}
if (strcmp(variable,"Yellow")==0) {
analogWrite(YELLOWLEDPIN,map(atoi(value),0,1023,0,255));
}
}
The processOutgoingMessages is empty because in this case we don't have information sent from the Arduino Board.
The doSync function is called only once when the iOS device gets connected to Arduino and sends information for initializing some Widgets like Switch Widget and Slider Widget.
If the LED has changed status from previous connection, the iOS has to know if the Switch Widget has to be initialized on or off. The same for the position of the Slider Widget.
void doSync(char *variable) {<br>
if (strcmp(variable,"Red")==0) {
iosController.writeMessage(variable,map(digitalRead(REDLEDPIN),0,255,0,1023));
}
if (strcmp(variable,"Yellow")==0) {
iosController.writeMessage(variable,analogRead(YELLOWLEDPIN));
}
}
You can easily add a switch and some simple code to switch on and off the red LED directly from the board to see how this function works.
More information about how to write the code and about the available library functions are in the documentation (Arduino Manager Documentation).
Step 6: Arduino Manager Configuration
Once you have downloaded and installed Arduino Manager from iTunes, you have to configure it for the project.
Just follow the procedure below:
- Enter in Edit mode, using the switch located in the bottom right side of the screen of your iOS device
- Double Tap on the fist free space. The list of available Widgets shows up.
- Select Switch Widget
- Replace the ?, with Red
- Double Tap on the second free space and add a LED Widget called Red
- Double Tap on the third free space and add a Slider Widget called Yellow
- Switch Edit to off
Step 7: Arduino Manager Connection Configuration
We are ready to configure the connection to our Arduino board, with this simple procedure:
- Tap on the sliding menu on the bottom left corner (see picture in the previous step to locate it)
- Tap Connections
- Tap Scan BLE to locate the Arduino around you
- In few seconds you should see a new entry starting with UART
- Select UART
- Close the sliding menu
- You should now see that Arduino icon on the bottom side of the screen gets green. The connection between your device and the board has been established
Now we are ready to work with the application.
Step 8: Controlling the LEDS From the IOS Device
Now the Arduino board should respond to our commands.
- Tapping on the switch you should see the red LED turning on
- Moving the slider the yellow LED gets brighten
This is just a quick overview of the Arduino Manager capabilities. You can now start to learn more yourself.
Next step is controlling the LEDs from your wrist.
Step 9: Controlling the LEDs From the Apple Watch
We are ready to work with Arduino Manager from the Apple Watch:
- Tap on Arduino Manager on your watch
- Tap on Connect and wait that the Widgets appear
- Tapping on the Switch you can see that the LED turns on and off
- Tapping on the - and + sign of the Yellow slider, you can increase and decrease the LED brightness
If you cannot get connected to the board at the first time, try again. More than one try may be required. This because the Watch has to wait for the iPhone that turns on the Bluetooth subsystem and establishes the connection. Bluetooth is turned off if not needed, to save battery power.
Step 10: Conclusions
This brief overview of Arduino Manager features just give you an idea of what you can do with it.
Any kind of Arduino project which requires an user interface can take advantage from Arduino Manager.
It also helps you during the development phase of your Arduino projects allowing you to focus on writing your own code and helping you in debugging your code.
More information are available here:
documentation is available here:
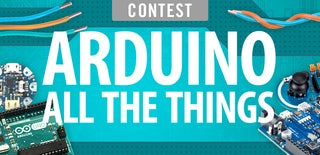
Participated in the
Arduino All The Things! Contest