Introduction: Cyberpunk Autoadjust Desk Clock
Right now, with Coronavirus and all, it feels sort of dystopian with a degree of breakdown in the social order, so my first idea for a clock was to take old parts combined with some tech in this retro futuristic clock using an old PCB from a water heater, 7 segment display, ESP8266, 2 leds and a buzzer. There are no buttons since the clock obtains the exact time from the web using WiFI. So it's just plug'n play.
Supplies
NodeMCU ESP8266
7 Segment Display 4 digit
2 leds
1 buzzer
1 old PCB from a heater - any old PCB with electronics components will be ok -
1 USB cable
Step 1: Circuits
Connect Display to GND and VIN, D2 and D3
Connect buzzer to GND and D4
Connect leds to GND and D5/D6
Step 2: Code
Step 3: WiFi Credentials
Before uploading the code to ESP8266, remember to edit your WiFi credentials
const char *ssid = "YourSSID";
const char *password = "YourPassword";
Then you may also need to update your time zone. The code is configured for Buenos Aires, Argentina.
// Set offset time in seconds to adjust for your timezone, for example:
// GMT +1 = 3600 // GMT +8 = 28800 // GMT -1 = -3600 // GMT 0 = 0
timeClient.setTimeOffset(-10800);
Step 4: Demo
Here is a small demo.
More projects at https://twitter.com/RoniBandini and https://www.instagram.com/ronibandini/
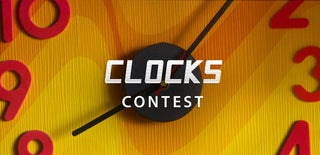
Participated in the
Clocks Contest