Introduction: DIY Larson Scanner
The name Larson scanner was coined by Evil Mad Scientist, the original maker of the Cyclon Jack-O-Lantern, which is based on the Cyclons of Battlestar Galactica. This project demonstrates how to build your very own Larson Scanner using LEDs, a shift register, and an Arduino, without having to but the kit.
Step 1: Tools and Materials
- Arduino Uno
- 8 LEDs
- 8 pieces of 100Ω resistor
- Jumper Cables
- Shift register - SN74hc95
Step 2: Wiring the LEDs
1. Connect all the cathodes, negative pins, of each of the LEDs to the common ground rail of the breadboard.
2. Connect all the anodes of each of the LEDs to a 100Ω resistor.
Step 3: Connecting the Shift Register to the LEDs and Arduino
3. Connect the shift register's pin 15 to the first LED's 100Ω resistor
4. Connect the shift register's pins 1 to 7 to the remaining seven LED's 100Ω resistors.
5. Connect the shift register's pin 8 to pin 13, and then connect one of them to the common ground rail on the breadboard.
6. Connect the shift register's pin 16 to pin 10, and connect one of them to the common 3.3V power rail.
7. Connect the shift register's pins 11, 12, and 14 to the Arduino's pins 2, 3, and 4.
8. Lastly, connect the 3.3V pin and GND pin from the Arduino to the common power and ground rails on the breadboard, respectively.
That's it, the circuit is complete!
Step 4: Coding
<pre>int datapin = 2; <br>int clockpin = 3; int latchpin = 4;
// We'll also declare a global variable for the data we're // sending to the shift register:
byte data = 0;
void setup() { // Set the three SPI pins to be outputs:
pinMode(datapin, OUTPUT); pinMode(clockpin, OUTPUT); pinMode(latchpin, OUTPUT); }
void loop() {
int index; int delayTime = 100; // time (milliseconds) to pause between LEDs // make this smaller for faster switching
// step through the LEDs, from 0 to 7
for(index = 0; index <= 7; index++) { shiftWrite(index, HIGH); // turn LED on delay(delayTime); // pause to slow down the sequence shiftWrite(index, LOW); // turn LED off }
// step through the LEDs, from 7 to 0
for(index = 7; index >= 0; index--) { shiftWrite(index, HIGH); // turn LED on delay(delayTime); // pause to slow down the sequence shiftWrite(index, LOW); // turn LED off } }
void shiftWrite(int desiredPin, boolean desiredState)
{ // First we'll alter the global variable "data," changing the // desired bit to 1 or 0:
bitWrite(data,desiredPin,desiredState);
// Now we'll actually send that data to the shift register. // The shiftOut() function does all the hard work of // manipulating the data and clock pins to move the data // into the shift register:
shiftOut(datapin, clockpin, MSBFIRST, data);
// Once the data is in the shift register, we still need to // make it appear at the outputs. We'll toggle the state of // the latchPin, which will signal the shift register to "latch" // the data to the outputs. (Latch activates on the high-to // -low transition).
digitalWrite(latchpin, HIGH); digitalWrite(latchpin, LOW); }
Step 5: Demo
The LEDs bounce like ping pong balls from one side to another. You can also change the LED shift to change the patterns generated by the Larson Scanner!
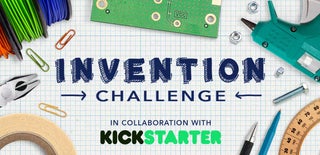
Participated in the
Invention Challenge 2017
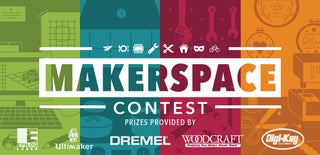
Participated in the
Makerspace Contest 2017