Introduction: DIY NES Classic Advantage Controller
A Nintendo Advantage inspired controller to use with the NES Classic Edition, also known as the NES Mini. This project is based off of the Wii RetroPad Adapter project by Bruno Freitas. I made this because of bad experiences with 3rd party controllers lagging and/or just not working.
Main "Advantages" over the stock controller and some other 3rd party controllers:
- Turbooooooo - On/Off toggle and adjustable rate from 1 to 35 presses per second (range can be changed)
- Home Button - Return to the NES Mini menu screen to change games or save states without having to press Reset on the console
The NES Classic Advantage Controller project uses an Atmega328P-PU microcontroller to emulate a Nintendo Classic controller but without the X/Y/L/R buttons and analog sticks.
I also made a 3d printed enclosure for the controller. The CAD and STL files are on Thingiverse. Instructions on using the enclosure are in this instructable. If you don't have a 3d printer, check out SlagCoin.com. SlagCoin is a great resource for building joysticks.
Step 1: Bill of Materials & Tools
Please support your local or in-country venders as I know you may get most, if not all of the materials cheaply from eBay. The 3d printed case is meant to fit the listed arcade controller components.
Bill of Materials
1 x Atmega328P-PU
1 x 0.1uF capacitor
2 x Mini Panel Mount SPDT Toggle Switch
2 x B10K Panel Mount Potentiometers (16mm body)
1 x Perfboard
1 x Sanwa joystick top (Ball or Bat)
2 x Sanwa OBSF-30 Buttons
3 x Microswitches(Use switches with a strong spring as this will push up the Home/Select/Start buttons)
2 x 5mm LEDs
14 x 1/4" pan head 4-40 screws
2 x 1/2" pan head 4-40 screws
4 x 1/2" countersunk 4-40 screws
1 x Wii controller cable (I took mine from a nunchuck)
1 x 1/8" metal rod at least 4 1/2" long
Bunch of wire (best to have different colors)
Optional items to make life a bit easier
- 2.54mm pin headers
- 2.54mm DuPont connectors (male and female)
- 28pin IC socket
- Heat shrink tubing (various sizes to bundle your wires 2 to 3 wires together)
Tools and other miscellaneous stuff
- Hobby knife
- 4-40 bottom or plug tap
- Phillips Screw driver
- Sand paper
- Small hobby files
- Hot glue gun or kragle (Krazy Glue)
- Tri-wing screwdriver (optional for opening Wii nunchuck).
- Your AVR programmer of choice (eg. Atmel-Ice, UsbAsp, an Arduino, etc.)
- Decal paper
Software
Either of the following depending on how you want to program your Atmega328P microcontroller
- Atmel Studio
- Arduino IDE
- WinAVR
Step 2: Flashing & Test Your Chip
You can use almost any microcontroller as long as the microcontroller is able to operate at 3.3V, has at least 2 ADC ports and at least 13 digital I/O ports. I used an Atmega328P since that has just the right amount of pins, can be programmed on an Arduino board, and is fairly cheap. The reason for 3.3V is that is what the Wii and NES Mini uses for the controllers.
Basic Steps in a nutshell:
- Download source code from GitHub
- Build the program in the src folder with your AVR toolchain of choice
- Burn Atmega328P fuses to L:0xE2 H:0xDE E:0xFD (this worked for me, you could try other settings as long as you do not run the chip faster than 8MHz)
- Flash the program to the chip
The following sections are two different methods of flashing your chip. Pick the one that best fits you.
Easy Way But Pricey
Use Atmel Studio 7 and an Atmel-Ice. This is the easy way because the source code is in an Atmel Studio 7 project so you can build and upload the program with just one button click. This is also the most expensive way as you will need an AVR programmer that is supported by Atmel Studio 7 out of the box. I purchased an Atmel-Ice (its not cheap) as I wanted to use the debugging feature and I know I will be using it for future projects. You could use another Atmel programmer that Atmel Studio supports out of the box, just make sure to change the project properties for your programmer.
- Connect the Atmel-Ice to your computer.
- Connect the Atmel-Ice to your chip. Follow the directions in the user manual. Side note: I had trouble connecting to my chip on crappy solderless breadboards, so use good quality breadboards or make/get a development environment board.
- Power your chip externally with 3.3V. This is a gotcha as I thought the Atmel-Ice would power the chip like how when you connect an Arduino through USB to your computer.
- Open Atmel Studio.
- Click on Tools -> Device Programming to open the Device Programming dialog window.
- In Interface setting (should be default view after dialog window opens), use the following settings.
- Tool: Atmel-Ice
- Device: ATmega328P
- Interface: ISP
- Click Apply
- Click on Read under Device signature, you should get the device signature for the chip, if not, check your connections
- Click on Fuses on the left side (still in the Device Programming dialog) and use the following settings.
- EXTENDED: 0xFD
- HIGH: 0xDE
- LOW: 0xE2
- Click Program.
- If the fuses have been set correctly, close the Device Programming dialog window.
- Download source code.
- Open the project by selecting NesClassicAdvantage.atsln in the source code src directory.
- You may have to fix references as I have the project in the directory D:\Projects\AVR\NesClassicAdvantage\src on my computer and my installation of Atmel Studio is in D:\Programs
- You may also have to update the project properties for your programmer.
- Okay, so might not be as easy as I make it out to be but still fairly easy once your references are set.
- Click on the little hollow Green triangle in the tool bar which is "Start Without Debugging", or you can press Ctrl-Alt-F5. Atmel-Studio will build and flash to your chip if all is good.
- If no error, your chip is ready to use.
Arduino IDE, Way Cheaper Way
Use the Arduino IDE and an Arduino. This is actually the way I flashed my chip before I got the Atmel-Ice. You will need the Arduino IDE and your choice of a programmer (Arduino, FTDI Programmer, USBasp, etc.). This would be the cheapest way as Arduino IDE is free. Genuine Arduinos are fairly expensive compared to clones but still way cheaper than an Atmel-Ice. Arduino clones, FTDI programmers and the USBasp clones are really dirt cheap from eBay. If you want cheap, this is the way to go. Also, you can still use Atmel Studio with these programmers but I'll leave that to you to research as there are many tutorials already out there for that.
- Burn fuse settings to your chip.
- I followed this tutorial Running ATmega328P on internal 8MHz clock (this is where I got the fuse settings from).
- Download source code
- Launch the Arduino IDE
- Create a new Sketch, File -> New
- Save the sketch.
- Replace the code in the default main sketch with the code from src/NesClassicAdvantage/NesClassicAdvantage/Sketch.cpp.
- Click on Sketch -> Add File to add the following files from the src/NesClassicAdvantage/NesClassicAdvantage/ directory
- WMCrypt.cpp
- WMCrypt.h
- WMExtension.cpp
- WMExtension.h
- Click on Sketch -> Verify/Compile. Make sure there are no errors after compiling.
- Burn the sketch to your chip on breadboard (you don't need an external crystal as the fuse you set earlier runs on the internal oscillator) with either of the methods below
- Using an Arduino as an AVR ISP (In-System Programmer), follow direction at end of the fuse tutorial above, it has what setting to use in the Arduino IDE.
- Use FTDI Programmer (google search, pick the one that works for you)
- Use a USBasp (google this one, pick the one that works for you)
Test Your Chip
Wire up the chip on a breadboard and test it out. The Fritzing shows how to hook up the chip. If all is well, you are pretty much done. All that is left is to put the components in a case. You can follow the rest of the instructable for using the case that I made. Or go to SlagCoin.com for information on building arcade joysticks.
Step 3: Perfboarding Part
This part is up to you how you want to design your perfboard or you can follow the pictures for how I wired mine up. Basically sockets with adjacent headers and extra headers for connecting a programmer and power.
Before you start soldering pieces into place, drill 1/8" holes the width and length of the standoffs. The length and width spacing are 25.4mm x 43.18mm, the mounting hole will line up with the holes on the perfboard. Test fit the holes with the four standoffs below the 30mm buttons.
Best to use IC sockets so that you can remove the chip if needed. I prefer the circular type sockets over the flat leaf type since they usually come in a strip that you can cut to size.
Use pin headers to make connecting your components easier should you need to replace them or incorrectly wire them up to the chip.
If you have space on your perfboard, put in headers to connect your programmer so that you can flash your chip if needed. The 2x3pins are for the ISP and the 2pins off to the side are for connecting power.
Note: Not shown in the pictures are extra pin headers for VCC and GND (pins 20 & 22) for the Wii cable to connect to. I only realized I needed the extra headers when I did the wiring.
Step 4: Parts Preperation
To make life easier later on. Tin the end of the terminals of your parts.
Step 5: Printing a Case
I made a case using FreeCAD and posted the files up on Thingiverse. Included is the FreeCAD file, so feel free to tweak the layout, adjust hole diameters for different arcade manufacturers, etc. to your needs. Forewarning: I'm still new to 3d parametric modeling and FreeCAD so hopefully the way I made the model makes sense.
- Download Nintendo Classic Advantage Controller files from Thingiverse
- Print the files with settings that works best for your printer with your choice of filament, mine were
- Extrusion width: 0.45mm
- Layer height: 0.20mm
- Vertical shells/perimeter/walls: 5
- Horizontal shells: 5 top, 5 bottom
- Infill: 20%
- Brim: 4mm for the top and bottom of the case (I have warping issues when the print takes up the whole bed), the rest the parts do not need a brim
- Support: not needed
The longest prints will be the bottom and top of the case. The bottom took 18 hours and the top took 13 hours for me to finish. The other parts all together were about an hour.
Notes:
Okay, I admit, I got my potentiometers off eBay. Quality control is not too good in my opinion. You may have to scale the turbo knob either larger or smaller by a tiny bit. The shaft sizes for the same type of potentiometer can vary enough that the knob doesn't have a nice snug fit.
Step 6: Placing Parts
As with building anything, you will need to test fit many times placing parts into their respective places.
- Test fit that your top and bottom of the case fit nicely over each other. If not, file/sand to whatever you need to do.
- Test fit that your arcade buttons slip into 30mm holes nicely and snaps into place. The buttons should just have the slightest amount of pressure that they do not spin freely.
- Test fit that your switches. You don't need to lock them down tight. Just make sure they fit through the holes and are not too loose.
- Test fit the potentiometers. The little rectangular block protruding near the pot holes are to help keep the pot from spinning.
- Test that the LEDS fit easily into their respective holes.
- Just eyeball the joystick mounting plate holes line up with the raised stand-offs of the top of the case.
- Test fit the size of your controller cable with the hole in the side of the case.
- If you need to enlarge the cable outlet, screw the bottom and top together with the 1/2" countersink screws and carefully use a reamer to size.
- Above the joystick area is spot to clamp the cable. Test that your cable is held firmly. Enlarge the hole if needed with files or sandpaper.
- All good? Good. So now take all your parts out.
Don't worry about the Home, Select, and Start buttons yet. They should not fit at the moment.
Step 7: Tap Time
Use a 4-40 bottom tap to cut threads into the 18 standoffs. Make sure to use a bottom tap so that you can cut threads almost all the way to the bottom.
Make sure to mark your tap with a permanent marker for the height of the standoff. The mark will help you not strip your threads when you hit the bottom. Better to mark above the standoff. If you mark the tap in the area that will be in the standoff, you will wipe away your mark from the tap quickly.
Tip: I found that I had to back out and clean the tap every few quarter turns as the plastic builds up and binds up fast. If you let the plastic cut bits build up to much you will strip the threads you just cut. Also, you will not be able to go all the way down as the plastic bits will build up on the bottom.
Screw in a screw partially in when you are done. This will help plastic at the top of the standoff to stick out.
Finally, use a hobby knife to cut off the runout/pushout or whatever you call that excess material sticking out of the screw hole.
Step 8: Button Building
Sand Stuff
- Use sandpaper or small hobby files to smooth the Home, Select, and Start button holes. I prefer using files but take care as files tend to cut fast.
- Sand the top portions of the printed buttons. You do not need to sand the flange.
- By the time the buttons and holes are smooth, the buttons should slide in and out of the hole easily. If not, use fine grit sandpaper on the button till the buttons run smooth.
Microswitch Assembly
- Place the printed buttons into the holes with the button flanges on the inside of the case.
- Cut the 1/8" metal rods into two 2-1/8" long pieces. I found 1/8" piano wire from my local hobby shop perfect for this.
- Stick the rods into the holes of the first microswitch. Enter the rods from the side of the switch that has the skinny cover (yellow side from the pictures). If you go the other way, you may pop off the cover. Push the rod through till 1/8" inch is left sticking out.
- Put a spacer on the rods.
- Slide the second microswitch on the rods with the same orientation as the first switch. The buttons and terminals must face the same way.
- Repeat adding a spacer and then the third final switch.
- Put the side mounts onto the microswitch assembly. The flat part with the screw holes will be on the same side as the microswitch button. You will have to hold the sides on.
- Place the microswitch assembly with its buttons facing the printed buttons. Ensure that the terminals are facing the inside of the case.
- Align the assembly mounting holes with the standoffs of the case and screw the assembly on.
- Test that the Home, Select, and Start buttons push in and rebound back easily.
Step 9: Panel Prettifying
Now is the time to prettify your case. I used decal paper from Testors to label the buttons.
- Print your labels and/or designs on regular paper. Cut and place on your panel with the components back in. You don't need to tighten down the pots and switches. They are in just to help with label/design placement.
- Resize/remake/replace your designs or labels till you are satisfied. Take a picture to help with placement later.
- Print your final designs on the decal paper. Make sure to follow the directions of your decal paper.
- Cut your labels/designs and use the picture you took to put the decals where they need to go.
- Finally remove the backing and stick the decals into place
Step 10: Permanent Parts Placement
Almost done!
- Place your parts into the case and use the 1/4" 4-40 screws to hold them into place. Do not overtighten and strip out the threads you made. If you do, use an extruded single line piece of filament (you know the skirt your printer makes before printing) and Kragle into the hole. May need to use two pieces if one piece is not enough to hold the screw in.
- For the Joystick, lightly screw the corners of the mount plate first. Use the middle standoffs as a guide to center the joystick and then tighten the corner screws. Add and tighten the center screws.
- With the parts in place, take a long piece wire that will be used as the ground bust. Use the wire to plan your route to one terminal of each component (except for the joystick since it comes with a wiring harness and the LEDs). I went in order from the chip to the 30mm buttons, microswitches, toggle switches, and finally the pots.
- Cut about 3/4" longer pieces the length between connecting components.
- Strip off 1/4" of insulation on each piece of wire.
- Twist the bare ends together.
- Tin the twisted wires together to create a terminal. Repeat for each twist to create a long bus wire.
- Solder the ground bus to a terminal on the components, except for the ones mentioned earlier.
- As with the ground bus, do the same for a power bus to the LEDs and pots.
- Solder the resistors from the LEDs to the 30mm button terminal connected to the ground bus.
- Now cut wires to length for the microswitches, 30mm buttons, toggle switches, and pots to their corresponding pins on the chip.
- Connect the joystick using the included harness to the chip. Use a voltmeter to figure out which wire is up/down/left/right.
- Affix the controller cable in place (that clamp above the joystick standoff).
- Wire up the controller cable to the chip.
- Test the controller before screwing the bottom of the case on.
- When all the buttons and joystick direction movements are correct, screw the bottom of the case on with the 1/2" 4-40 countersunk screws.
- Jump for joy.
Step 11: Play!!!
Play!!!
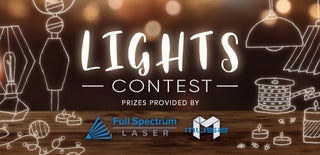
Participated in the
Lights Contest 2017