Introduction: DIY Thermometer With TTGO T Display and DS18B20 V2
Greetings everyone, and welcome back.
Here's something useful: A DIY thermometer made completely from scratch using a custom PCB and a couple of 3D-printed parts.
The DS18B20 temperature sensor probe and the TTGO T-display board form the project's core, and we have incorporated a lithium cell circuit onboard to power the circuit. The DS18B20 temperature sensor measures the temperature, which is subsequently shown on the TTGO Board's display.
This Instructables covers the entire build process of this project, so let's get started.
Supplies
These were the materials required in this build-
- TTGO T Display DEV Board
- Custom PCBs
- 3D-printed parts
- DS18B20
- IP5306 IC
- 10uF Capacitors
- 3.7V 100mAh LiPo Cell
- 5.6uH Inductor
- horizontal push button
- USB Micro Port
- M2 Screws
Step 1: Previous Project
This project is actually Version 2 of a similar project that was produced before. It had the same configuration, but it was assembled with a 3D printed body and the battery was connected directly to the TTGO's battery connection.
You can read about the previous project here.
https://www.instructables.com/DIY-Thermometer-With-TTGO-T-Display-and-DS18B20/
Making this arrangement was difficult; therefore, it was time to simplify the model by installing every component on a PCB.
Step 2: New Design
First, we model the TTGO board, the DS18B20 sensor, and the battery using Fusion360. Next, we position the temperature sensor on the front side and the TTGO board a little away from it. To connect both of them, we model a PCB.
A holder part with two screw mounts that fit into the PCB holds the temperature sensor in place. One PCB will be positioned on top, containing all of the components, and a second PCB will be placed in its place, empty.
To prepare the PCB design for the following setup, we use the measurements from the Cad design of the PCB.
Step 3: PCB Design
The first step in the PCB design process is creating the schematic, which shows the TTGO T-Display board connected to the DS18B20 temperature sensor by a CON3 header pin. We attach a resistor between the sensor pin and the CON3 header pin of the temperature sensor's VCC.
The DS18B20 digital thermometer provides 9-bit to 12-bit Celsius temperature measurements and has an alarm function with nonvolatile, user-programmable upper and lower trigger points.
The DS18B20 communicates over a 1-wire bus that by definition, requires only one data line (and ground) for communication. This sensor pin is connected to GPIO2 of the TTGO T-Display Board.
This sensor comes in two forms: one that looks like a transistor and a probe-like device that we are using in this project.
Check out its datasheet for more information about the sensor itself.
https://datasheets.maximintegrated.com/en/ds/DS18B20.pdf
Our selected power source is the IP5306 IC, a well-known Li-ion power management IC that outputs a steady 5 volts from a 3.7 volt Li-ion cell and offers features like charging cutoff and battery fuel level indication.
We create the PCB outline and arrange everything in accordance with the layout by using the PCB Design layout from the Cad design.
Step 4: PCBWAY PCB Service
After completing the PCB design, we export the gerber data and send it to PCBWAY for samples.
An order was placed for a yellow soldermask and white silkscreen.
After placing the order, I received the PCBs within a week, and the PCB quality was pretty great. The silkscreen I used is completely random and asymmetrical, so it's pretty hard to make, but they did an awesome job of making this PCB with no errors whatsoever.
You guys can check out PCBWAY if you want great PCB service at an affordable rate.
Step 5: PCB Assembly Process
- Using a solder paste dispensing needle, we first add solder paste to each component pad individually to begin the PCB assembly process. In this instance, we are using standard 37/63 solder paste.
- Next, we pick and place all the SMD components in their places on the PCB using an ESD tweezer.
- With extreme caution, we lifted the complete circuit board and placed it on the SMT hotplate, which increases the PCB's temperature to the point at which the solder paste melts and all of the components are connected to their pads.
- Next, we add all the THT components, which include the header pin, horizontal switch, and USB micro port, to their locations and then solder their pads using a soldering iron.
- At last, we added the TTGO T-Display board to its location through the header pins, and the PCB assembly is now complete.
Step 6: MAIN ASSEMBLY
- The main assembly process starts by soldering the positive and negative LiPo cells to the circuit's battery terminals.
- Next, we attach the temperature sensor's wires to the pads provided on the sensor circuit.
- The temperature sensor holder and the back holder with the TOP PCB are then fastened with M2 screws.
- To keep the LiPo cell in its place, we use Kapton tape to secure it with the back side of the TOP PCB.
- Next, we use an empty PCB, place it on the bottom side of the model, and secure it in place using four M2 screws.
- The thermometer is now complete; let's take a look at its code in the next step.
Step 7: CODE
#include <OneWire.h>
#include <DallasTemperature.h>
#include <TFT_eSPI.h> // Graphics and font library for ST7735 driver chip
#include <SPI.h>
#include <WiFi.h>
#define TFT_BLACK 0x0000 // black
#define ONE_WIRE_BUS 2
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
TFT_eSPI tft = TFT_eSPI(); // Invoke library, pins defined in User_Setup.h
void setup(void)
{
tft.init();
tft.setRotation(1);
Serial.begin(9600);
sensors.begin();
}
void loop(void)
{
Serial.print(" Requesting temperatures...");
sensors.requestTemperatures(); // Send the command to get temperature readings
Serial.println("DONE");
tft.fillScreen(TFT_BLACK);
tft.setCursor(0, 0, 2);
tft.setTextColor(TFT_WHITE,TFT_BLACK);
tft.setTextSize(2);
tft.println("Temperature is: ");
tft.setCursor(0, 40, 2);
tft.setTextColor(TFT_WHITE,TFT_BLACK);
tft.setTextSize(3);
tft.println(sensors.getTempCByIndex(0));
delay(2000);
}
Let's break down the code:
#include <OneWire.h>
#include <DallasTemperature.h>
#include <TFT_eSPI.h> // Graphics and font library for ST7735 driver chip
#include <SPI.h>
#include <WiFi.h>
#define TFT_BLACK 0x0000 // Black color code for the TFT screen
#define ONE_WIRE_BUS 2 // Pin connected to the DS18B20 sensor
OneWire oneWire(ONE_WIRE_BUS); // OneWire instance with the specified pin
DallasTemperature sensors(&oneWire); // DallasTemperature instance using the OneWire instance
TFT_eSPI tft = TFT_eSPI(); // TFT_eSPI instance for the TFT LCD screen
Here, the required libraries are included, and some constants and instances for OneWire, DallasTemperature, and TFT_eSPI are defined.
void setup(void)
{
tft.init(); // Initialize the TFT screen
tft.setRotation(1); // Set the screen rotation
Serial.begin(9600); // Initialize serial communication
sensors.begin(); // Initialize the DS18B20 sensor
}
In the setup function, the TFT screen is initialized, its rotation is set, serial communication is started, and the DS18B20 sensor is initialized.
void loop(void)
{
Serial.print(" Requesting temperatures...");
sensors.requestTemperatures(); // Send the command to get temperature readings
Serial.println("DONE");
tft.fillScreen(TFT_BLACK); // Clear the TFT screen with black color
// Display temperature information on the TFT screen
tft.setCursor(0, 0, 2);
tft.setTextColor(TFT_WHITE, TFT_BLACK);
tft.setTextSize(2);
tft.println("Temperature is: ");
tft.setCursor(0, 40, 2);
tft.setTextColor(TFT_WHITE, TFT_BLACK);
tft.setTextSize(3);
tft.println(sensors.getTempCByIndex(0)); // Display the temperature value
delay(2000); // Wait for 2 seconds before the next loop iteration
}
In the loop function, it requests temperature readings from the DS18B20 sensor, clears the TFT screen, and displays the temperature information on the screen. The temperature is obtained using sensors.getTempCByIndex(0) and printed on the screen. Then, it waits for 2 seconds before the next iteration.
Overall, this code reads the temperature from a DS18B20 sensor and displays it on an SPI-connected TFT LCD screen.
These are the libraries you need to download and install first before using this sketch:
https://github.com/milesburton/Arduino-Temperature-Control-Library
Step 8: TESTING
We first used a hot medium—a cup of tea—to test the thermometer. After dipping the DS18B20 Sensor's probe into the tea cup and waiting a minute for readings, the temperature reached 64°C.
Next, we plunge the DS18B20 Sensor probe into a cup of ice and water, a cold medium, and allow it to take readings for a minute. This yields temperature readings of 0.56°C.
Step 9: CONCLUSION
Here is the end result of this straightforward build: a DIY thermometer that functions perfectly across all temperatures, hot or cold.
We saved a lot of space by employing a development board like the T-display board, which has an inbuilt display to make things compact.
The DIY Thermometer project has reached its final edition, and nothing has to be changed going forward because everything functions flawlessly.
I have included all the files so you may try making one on your own.
Leave a comment if you need any help regarding this project. This is it for today, folks.
Thanks to PCBWAY for supporting this project.
You guys can check them out if you need great PCB and stencil service for less cost and great quality.
And I'll be back with a new project pretty soon!
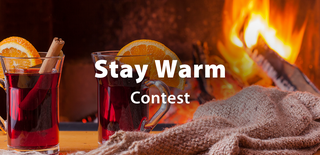
Participated in the
Stay Warm Contest