Introduction: Arduino Color Sensing Glove That Sends the HEX Code to Your Computer!
UPDATE: I remade the glove (added another touch area for when to scan, and changed the RGB LEDs) and have added the video so you can see it working.
Wouldn't it be cool to see something on our desk or nearby, a color that we like, and add it directly to our projects on our computer? Now you can!
What are we making?
You can make this hand-worn wearable that uses a color sensor to detect colors from the environment around us. Then it lights up NeoPixels with the color we scanned, and writes the HEX code to an OLED on the glove. This is an Arduino-based system with only a few components.
Why should you make this?
When you use it to sense the colors around you - which is already cool and fun, you can also send those colours to your computer program to use them directly! This HEX code can be sent to our computer (with touch input) - directly into what we are working on, to add the HEX code for us.
Note that you will need soldering skills if you want to put this on a glove or similar.
Supplies
The supplies I've used for this project include:
- Adafruit Flora board or Circuit Playground Classic
- Flora sewable color sensor
- 4x4 grid NeoPixels but any shape will work
- OLED screen 128 x 64
- wires- silicone is best when making a wearable because it's very flexible
- soldering or conductive thread to sew the connections
- you'll need a small piece of conductive fabric to act as a touch input button to send the HEX value to your computer
- Felt, neoprene, or material for the glove, or a recycled glove is even more eco!
In this case, I won't be using a battery if you want to enable the feature of transferring the HEX code to the computer, we will need a cable to connect to our computer so we will use that for power too.
Wearable Electronics items purchased from Tinker Tailor.
Step 1: Draw Out Your Connections
Sometimes it can be easier to follow your connections if you map them out your project. I usually start by adding all the components I need and then drawing my mappings.
For this circuit, we will connect:
The color sensor
- Ground - Ground on Flora
- Power - Power on Flora
- SDA - SDA on Flora
- SCL - SCL on Flora
The OLED
- Ground - Ground on Flora
- Power - Power on Flora
- SDA - SDA on Flora
- SCL - SCL on Flora
NeoPixels
- Ground - Ground on Flora
- Power - Power on Flora
- DO - to pin 10 on Flora
Conductive fabric as touch input
- Fabric sewn or wire connected to pin 9 on Flora, pin 12 Circuit Playground
Step 2: Check Each Component and Connection
To do this step by step we should add the color sensor first. Then we can check it's working. We will do this for all of our parts, the color sensor, the NeoPixels, and the OLED.
After you hook up the color sensor using I2C and the connections in the previous step, we will upload the code to our board.
First, we need to add a library.
Step 3: Adding a Color Sensor
Let’s open the Arduino IDE and install a library to use this sensor.
Open the Library Manager, and search for Adafruit TCS34625.
The color sensing library didn’t appear when I searched - I tried searching for color sensor, which worked. Click to click Install, and Install all.
This library is for a slightly different color sensor so I modified the sample file slightly.
#include <Wire.h> //include Wire.h to be able to communicate through I2C on Arduino board
#include "Adafruit_TCS34725.h" //Colour sensor library
//Create colour sensor object declaration, to see effects of different integration time and gain
//settings, check the datatsheet of the Adafruit TCS34725.
Adafruit_TCS34725 tcs = Adafruit_TCS34725(TCS34725_INTEGRATIONTIME_50MS, TCS34725_GAIN_4X);
void setup() {
Serial.begin(9600);
Serial.println("Color View Test!");
//Start-up colour sensor
if (tcs.begin()) {
Serial.println("Found sensor");
} else {
Serial.println("No TCS34725 found ... check your connections");
while (1); // halt!
}
}
void loop() {
uint16_t clear, red, green, blue;
//Collect raw data from integrated circuit using interrupts
tcs.setInterrupt(false); // turn on LED
delay(60); // takes 50ms to read
tcs.getRawData(&red, &green, &blue, &clear);
tcs.setInterrupt(true); // turn off LED
// Figure out some basic hex code for visualization
uint32_t sum = clear;
float r, g, b;
r = red;
r /= sum;
g = green;
g /= sum;
b = blue;
b /= sum;
r *= 256; g *= 256; b *= 256;
Serial.print("HEX: \t");
Serial.print((int)r, HEX); Serial.print((int)g, HEX); Serial.print((int)b, HEX);
Serial.print("\t \t RGB: \t");
Serial.print((int)r ); Serial.print(" "); Serial.print((int)g);Serial.print(" "); Serial.println((int)b );
Serial.println();
}
Upload the sketch to Flora (or what board you are using).
Open the Serial Monitor. You'll see a display of the color values in the Serial Monitor output.
The color sensor will blink as it’s sensing. The output to the monitor depends on the color of the item you hold over the sensor. I held a pink item which gave me that readout.
Step 4: Connecting and Testing Your OLED
The OLED has 4 pins, 2 are for the I2C protocol. As previously, connect SDA on the OLED to SDA on the Flora, and connect the SPI on the screen to the SPI on the Flora. Then hook up the ground pin and finish power.
We are using the same SDA and SCL pins. The components we put on these pins have their own address.
To easily program the OLED let’s install a library. Open the Library Manager and search for Adafruit_SSD1306. This library will also ask to install Adafruit_GFX at the same time, so install both.
Run this sample sketch to display Hello World on the screen:
#include <Wire.h>
#include “Adafruit_GFX.h”
#include “Adafruit_SSD1306.h”
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 64
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
void setup() {
Serial.begin(9600);
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000);
oled.clearDisplay();
oled.setTextSize(3);
oled.setTextColor(WHITE);
oled.setCursor(0, 10);
oled.println("Hello World!");
oled.display();
}
The OLED should display Hello World.
Step 5: Combining the Color Sensor and OLED
Make sure both components are connected (the color sensor and the OLED) and then upload the combined code. I've combined it here:
#include <Wire.h> //include Wire.h to be able to communicate through I2C on Arduino board
#include "Adafruit_TCS34725.h" //Colour sensor library
#include "Adafruit_GFX.h"
#include "Adafruit_SSD1306.h"
//Create colour sensor object declaration, to see effects of different integration time and gain
//settings, check the datatsheet of the Adafruit TCS34725.
Adafruit_TCS34725 tcs = Adafruit_TCS34725(TCS34725_INTEGRATIONTIME_50MS, TCS34725_GAIN_4X);
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
// declare an SSD1306 display object connected to I2C
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
//Start-up colour sensor
if (tcs.begin()) {
Serial.println("Found sensor");
} else {
Serial.println("No TCS34725 found ... check your connections");
while (1); // halt!
}
}
void loop() {
uint16_t clear, red, green, blue;
//Collect raw data from integrated circuit using interrupts
tcs.setInterrupt(false); // turn on LED
delay(60); // takes 50ms to read
tcs.getRawData(&red, &green, &blue, &clear);
tcs.setInterrupt(true); // turn off LED
// Figure out some basic hex code for visualization
uint32_t sum = clear;
float r, g, b;
r = red;
r /= sum;
g = green;
g /= sum;
b = blue;
b /= sum;
r *= 256; g *= 256; b *= 256;
Serial.print("HEX: \t");
Serial.print((int)r, HEX); Serial.print((int)g, HEX); Serial.print((int)b, HEX);
Serial.print("\t \t RGB: \t");
Serial.print((int)r );
Serial.print(" ");
Serial.print((int)g);
Serial.print(" ");
Serial.println((int)b );
Serial.println();
oled.clearDisplay(); // clear displa
oled.setTextSize(2); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(2, 10); // position to display
oled.println("HEX:");
oled.setCursor(50, 10); // position to display
oled.println((int)r, HEX);
oled.setCursor(74, 10); // position to display
oled.println((int)g, HEX);
oled.setCursor(98, 10); // position to display
oled.println((int)b, HEX); // text to display
oled.display(); // show on OLED
delay(5000);
oled.clearDisplay(); // clear display
oled.setTextSize(2); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(4, 10); // position to display
oled.println("reading...");
oled.display(); // show on OLED
delay(1000);
}
Upload it to your Flora/Circuit Playground, then run it. Test it by holding something with color to your color sensor.
We now have an input that is receiving data and creating an output based on that data!
Step 6: Adding and Connecting NeoPixels
Connect your NeoPixels. Mine have their data pin connected to pin 10.
In the Arduino IDE add the Adafruit NeoPixel library. This will install sample files for us that we will use to test we've connected it correctly and the NoePiexls are working.
Open the file strandtest which is in File > Examples > Adafruit NeoPixel > strandtest.
Change the line of code #define LED_PIN 6 to have the same value as your pin, I chose pin 10.
#define LED_PIN 10
Secondly, the line after that one is a count of the number of NeoPixel LEDs used. Count the lights you are using in your strip, strand, or shape, and put this number in. Mine looks like this:
#define LED_COUNT 16
Upload, and you should see a display of different colors and effects.
Step 7: Combine the Code for These 3 Features
Now that we have added and checked all 3 components, let's put the code together.
#include <Wire.h> //include Wire.h to be able to communicate through I2C on Arduino board
#include "Adafruit_TCS34725.h" //Colour sensor library
#include "Adafruit_GFX.h"
#include "Adafruit_SSD1306.h"
#include "Adafruit_NeoPixel.h"
//Create colour sensor object declaration, to see effects of different integration time and gain
//settings, check the datatsheet of the Adafruit TCS34725.
Adafruit_TCS34725 tcs = Adafruit_TCS34725(TCS34725_INTEGRATIONTIME_50MS, TCS34725_GAIN_4X);
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define LED_PIN 10
#define LED_COUNT 16
Adafruit_NeoPixel strip(LED_COUNT, LED_PIN, NEO_GRB + NEO_KHZ800);
// declare an SSD1306 display object connected to I2C
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
//Start-up colour sensor
if (tcs.begin()) {
Serial.println("Found sensor");
} else {
Serial.println("No TCS34725 found ... check your connections");
while (1); // halt!
}
strip.begin(); // INITIALIZE NeoPixel strip object (REQUIRED)
strip.show(); // Turn OFF all pixels ASAP
strip.setBrightness(50); // Set BRIGHTNESS to about 1/5 (max = 255)
}
void loop() {
uint16_t clear, red, green, blue;
//Collect raw data from integrated circuit using interrupts
tcs.setInterrupt(false); // turn on LED
delay(60); // takes 50ms to read
tcs.getRawData(&red, &green, &blue, &clear);
tcs.setInterrupt(true); // turn off LED
// Figure out some basic hex code for visualization
uint32_t sum = clear;
float r, g, b;
r = red;
r /= sum;
g = green;
g /= sum;
b = blue;
b /= sum;
r *= 256; g *= 256; b *= 256;
Serial.print("HEX: \t");
Serial.print((int)r, HEX); Serial.print((int)g, HEX); Serial.print((int)b, HEX);
Serial.print("\t \t RGB: \t");
Serial.print((int)r );
Serial.print(" ");
Serial.print((int)g);
Serial.print(" ");
Serial.println((int)b );
Serial.println();
colorWipe(strip.Color(r, g, b), 50);
oled.clearDisplay(); // clear displa
oled.setTextSize(2); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(2, 10); // position to display
oled.println("HEX:");
oled.setCursor(50, 10); // position to display
oled.println((int)r, HEX);
oled.setCursor(74, 10); // position to display
oled.println((int)g, HEX);
oled.setCursor(98, 10); // position to display
oled.println((int)b, HEX); // text to display
oled.display(); // show on OLED
delay(5000);
}
void colorWipe(uint32_t color, int wait) {
for(int i=0; i<strip.numPixels(); i++) { // For each pixel in strip...
strip.setPixelColor(i, color); // Set pixel's color (in RAM)
strip.show(); // Update strip to match
delay(wait); // Pause for a moment
}
}
When the code is working:
- you’ll see flashing on the color sensor, this means it is taking a reading
- then the HEX code is written to the OLED screen, and
- the color is sent as RGB values to the NeoPixels
- NeoPixels light up with the color sensed (close to it)
You can check the HEX code displayed on the screen, type the value into a color hex website, https://www.color-hex.com/ to check if the value is close to what your expecting.
Step 8: Let's Get Sewing!
To make it into a wearable glove, you can sew the components to a glove that you already have. If you have felt, you can use that to create a quick prototype fingerless glove. You need a piece of felt big enough for our hand, front and back.
Place your hand on top of the felt, and measure across where your knuckles are.
This will be the widest part of the glove. The material needs to cover that part of your hand, and account for the thickness of your hand.
See the image which shows the area we need to measure, and the felt draped over the hand to be sure there is enough of the fabric.
Once you’ve cut the felt, fold it over your hand to be sure there is overlap so will fit once it is sewn (or glued) together.
You can use safety pins to hold the shape in place. Then, put the glove on to feel where the components will fit. Keep in mind – that the purpose is this is to be used by someone typing on a computer because we want to help them with finding HEX code. So, make sure you can hold a mouse easily when the components are on it.
Once you are satisfied with the placement, put the components on the felt and glue them into place.
Step 9: Sew or Solder Your Components
Now you need to firmly attach through sewing with conductive thread or soldering the connections.
We will have a USB cable going back to the computer, so make sure your USB port on your Flora / Circuit Playground is facing it comfortably.
Don't forget to add your conductive fabric in a place that is convenient and easy for the wearer to touch to activate the transfer of HEX code to their computer. (To test the code though you only need to touch the pin we defined - 9 for Flora, and 12 for CircuitPlayground and it will work.)
The sensor is just out of view on mine because I want it near my pinky, on the underside for easy scanning. Once the glue is dry you can sew the components into place.
- I map out my connections before I sew to be sure I know where the threads might overlap. This rapid prototype in felt is a good way to test your wearable and find if there are usability issues.
I’m going to transfer my circuit to a glove design made from scuba-style fabric neoprene for a stretchable fit. This would become a prototype iteration to test a more permanent solution because I like the functionality of this wearable!
Step 10: Final Complete Code to Add HEX Sending to Your Computer Function
Here is the final code. It's also available on GitHub in case there are revisions.
For all boards, including Flora: https://github.com/cmoz/instructables/tree/main/HandHEX_AllBoards
For Circuit Playground: https://github.com/cmoz/instructables/blob/main/HandHEX_CP/HandHEX_CP.ino
Step 11: Enjoy!!
Put your mouse cursor where you want the HEX code to be written, a text file, word document, graphic design program etc. Enjoy!
To see more of my projects and wearables, visit http://christinefarion.com, follow me on Instagram, and Twitter is great if you have any questions or comments!
Wearable Electronics items purchased from Tinker Tailor.
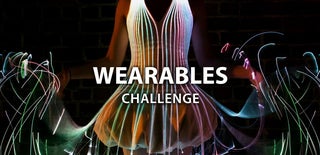
Participated in the
Wearables Challenge