Introduction: Electronic Puzzle Box With Secret Compartment
The goal of this instructable is to create a simple lockable box that can be opened by solving a puzzle. The puzzle must be interesting, easy to understand and it must be possible to solve it with the help of a pen and paper (or straight from the head). The system will be controlled by arduino.
Step 1: Intro and Inspiration
This project is inspired by the game Submachine 1: The Basement, created by Mateusz Skutnik. It's a logical adventure, released in 2005 and I've enjoyed the whole series since I was a kid.
Original version is very simple. There are four bells and four cubes in the room. The ringing of each bell interacts with a different combination of cubes. If a cube is down, it will rise when the bell rings, and if it is raised, it will fall. (So the bell changes the state of the assigned cubes.) The goal is to find a combination that picks up all the cubes and then, the secret compartment opens. In my variant, the bells will be replaced by buttons and cubes will be replaced by LEDs. Contestants who approach will receive a single instruction - use the buttons to light up all 9 LEDs!
Step 2: Parts List (BOM)
9x LED
9x LED holder
9x 1k resistor
6x push switch
6x 10k resistor
arduino nano
9v battery
9v battery connector
2x 1n4148 diode (or 1n4001)
spst switch
dc connector (3 pin)
cable wires 5v relay (Omron G5V-1)
solenoid, electromagnet or servo
dcdc buck converter
(if you want to use bicolor leds, you will need an extra 9x 2n3906 transistor and another 18x 1k resistors)
Step 3: Schematics
The system will be powered by a 9V battery that will power the electromagnet directly. The one I used did not work with a voltage of 5V, the plate was attracted when I applied more than 6.4V. DC connector is used to connect an external power supply in case the battery runs out. Without it, the box could only be opened by force and thus damage it.
I chose the standard 3 pin DC connector, so that when the male connector is connected, the contact with the battery opens so that no current flows into it (and therefore does not damage it).
The voltage for powering the Arduino will be provided by the dcdc buck converter. It is necessary to set the value of 5V at the output with the trimmer before connecting the Arduino. The buttons are connected to ground using pull down resistors and a current limiting resistor is added in series with each LED. Pin D2 is reserved for a relay whose coil is bridged by a diode, for overvoltage protection. The electromagnet is also protected by a diode.
If you want to use two-color LEDs instead of single-color ones, there is a driver schematics in the lower left part. You will need additional nine pnp transistors 2n3906 and 18x 1k resistors.
Step 4: Choosing a Wooden Box
I wanted to integrate the puzzle into a wooden box. Wooden boxes are widely accessible, and easy to paint with stains, but it is also possible to use a plastic box or 3d print a custom one. It is important that the box is openable in some way, so that it will be possible to design a locking mechanism. The LEDs and buttons will be located on the top of the box.
Step 5: Designing Space for Electronics
The box will need to fit a 9v battery, arduino, solenoid (or servomotor), dcdc converter and wires. The Arduino nano can also be placed on the openable part of the box, but the other parts must be placed in the lower part. It will be separated from the storage part by a wooden partition, which needs to be cut out.
I measured the inner dimension of the box and then I cut the partition and sanded the edges a bit.
Step 6: Design of Stand for the Electromagnet
The electromagnet I used is from an old printer. On one side there is a plate which is held by a spring. If current begins to flow through the coil, an electromagnetic field is created which attracts the plate and holds it. When the current flow is interrupted, the plate is released to its original position.
First, it is necessary to design a stand for the electromagnet itself, so that it can be screwed to the box and held firmly. I designed the holder in Fusion360, which is free for hobbyists and students.
Step 7: Design of the Locking Mechanism
For the design of the lock we need to construct some L-shape, which will be mounted under the top of the box. After closing the box, the plate hits the L-shape and thus mechanically does not allow the box to open until current begins to flow through the electromagnet.
This piece is again 3d printed and I fastened it to the box with two small screws.
Step 8: Drilling Holes
I covered the top of the box with masking tape and used a ruler to indicate the places to drill. I decided to place the LEDs in a combination of 3x3 on the left and the buttons on the right. The layout of the parts is up to you as you like.
I also covered a small piece of the rear wall, there will be an on / off switch and a connector for external DC voltage.
Step 9: Wood Staining
I painted the wood with mahogany stain. To achieve a darker shade, two coats should suffice. I think mahogany goes well with red scattered LEDs, but it is also possible to use dark oak or leave the box in its original color. After painting, the wood should be allowed to dry for a few hours.
Step 10: Mounting the Components
After drying, I installed LED holders with LEDs and push buttons in the sanded holes. I secured the holders with a glue gun so that they would hold firmly. Subsequently, I soldered a common cathode to the LEDs and also a common 5V branch for all buttons.
Step 11: Wiring the Arduino
On the Arduino I soldered a 1k current limiting resistor to each digital output that is assigned to one LED. Then I soldered pull down resistors to the analog inputs. Then, I soldered all the LEDs and buttons to the corresponding pins of the Arduino.
Step 12: Wiring the Battery, Power Switch and DC Plug
According to the schematics, I soldered the 9V battery clip and the output of the DC connector via the on / off switch and then to the dcdc buck converter. I set the output of the converter to 5V with a trimmer and prepared a longer cable for connection with arduino. I attached the relay that will turn on the electromagnet, directly to the dcdc converter.
Step 13: Placing the Battery
I mounted the solenoid holder to the bottom of the box and also secured it with the glue gun, I offset the dcdc converter from the bottom using a 2x2x2 cm 3d printed calibration cube. The 9V battery was inserted in the gap on the left. There is free space at the bottom so that the solenoid plate can move.
Step 14: Programming Arduino
The code for arduino is very simple. First, the digital pins are set as outputs and the analog pins as inputs. The LEDs are assigned to pins D4 to D12 and the buttons to pins A1 to A6. One pin - D2 is reserved for the electromagnet. The program cycles to see if any of the buttons are pressed. If so, the following function is called, which simply swaps the logic state of the output (LED):
void toggle(int lednumber){ digitalWrite(lednumber, !digitalRead(lednumber)); }
At the end of the cycle, a simple if condition verifies if all LEDs are lit. If so, pin D2 is set to the logic level HIGH and the solenoid turns on.
How to design the combination?
We must first choose a combination of presses that will open the box. If we look at each button separately, it doesn't matter how many times it is pressed. All that matters is whether the button has been pressed an odd or even number of times.
Let the correct combination be achieved by pressing four buttons. First, select any two buttons from the correct combination and assign them a combination of LEDs at random or according to any pattern you like. Then match the combinations for the remaining two buttons so that the puzzle can be assembled by pressing all four buttons. Assign the same LEDs to several buttons so that while pressing the correct combination (one button after another), it does not seem to light up all 9 LEDs at first glance.
As for the other buttons (those that should not be pressed, or can be pressed an even number of times - which gives the same result), thus assign random combinations to confuse the competitors.
Attachments
Step 15: Project Completed
The project is completed. To cover the components, the inside of the box can be additionally covered with a layer of foam or cardboard or paper. I decided to leave it as it is. The hinges will still need a drop of oil when they creak.
Now you can hide something in the box, use it just for fun or as one of the parts of the escape room game.
Have fun solving the puzzle.
Step 16: How to Solve This Puzzle
One of the goals of this puzzle was to be able to solve it using a pen and paper (or straight from the head).
Contestants who approach have a single instruction - use the buttons to light up all 9 LEDs! When they first look at the box and start pressing the buttons, they will realize two important things:
- Each of the buttons simply does not turn on or off certain LEDs, but changes their state. (From off to on and from on to off.)
- It doesn't matter how many times the button was pressed. All that matters is whether the button has been pressed an odd or even number of times.
If these two things are clarified, competitors should sketch a simple layout of the device (first picture) and mark the individual LEDs and switches in some way. Let's say the LEDs are marked with the letters A to E and the buttons with the numbers 1 to 6.
The next step is to find out for each LED which buttons affect it. The state of some LEDs is switched by two, others by up to three buttons.The corresponding button numbers are then written to the letters (second picture).
Let's look at the LED marked with the letter A. Its state is changed only by buttons 3 and 4. This means that in order to be lit, either button 3 or button 4 must be pressed, but certainly not both (and also neither of them).
In this step, the contestants must choose a precondition. Suppose they choose the pressing of button 4 as correct. This also means that button 3 must not be pressed:
__34__
Let's look at the LED marked with the letter C. If the button number 4 was pressed to make this LED light on, the button 1 must not be pressed at the same time, because it would light this led back off:
1_34__
As in the previous step, let's now look at the LED marked D. If button number 1 must not be pressed, button 5 must be pressed for LED D to light up:
1_345_
Now let's look at the LEDs marked E and G. Both are lit because we concluded that button 5 must be pressed. This means that buttons 2 and 6 must not be pressed, as LEDs E and G would turn off:
123456
At this point, the key finding is coming. Competitors find that since buttons 1, 2 and 6 cannot be pressed, it is not possible to turn on the LED marked H in any other way. This means that the original assumption (Button 4 must be pressed) was incorrect.Button 4 must therefore not be pressed:
___4__
Based on LEDs A and C, we find that if button 4 must not be pressed, button 3 and button 1 must be pressed:
1_34__
The letter D indicates that if button 1 has been pressed, button 5 must not be pressed:
1_345_
Finally, the letters E and G indicate that if button 5 cannot be pressed, buttons 2 and 6 must be pressed:
123456
Hooray. The states of all buttons are detected and can be verified by pressing directly on the box (or from the second picture). The box opens and the contestants find a hidden item or key to the next task :)
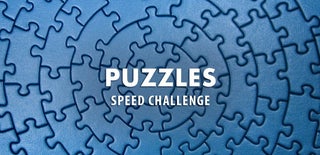
Participated in the
Puzzles Speed Challenge