Introduction: Esp32 Air Monitor
In this tutorial, you will build an air monitor that monitors air temperature, humidity and pressure, all using Blynk, an esp32, a DHT22 and a BMP180.
Supplies
- esp32 Microcontroller
- DHT22
- BMP180
Step 1: Setup Blynk
You will need Blynk for this project so that you can see the results in real time anywhere in the world. You can see how to setup Blynk in my previous tutorial.
Step 2: Install the Libraries
The first library you will need to install is the SparkFun RHT03 Arduino Library, you can download this from https://learn.sparkfun.com/tutorials/rht03-dht22-humidity-and-temperature-sensor-hookup-guide?_ga=2.53575016.1755727564.1559404402-688583549.1496066940#library-installation. After downloading it open the Arduino IDE and go into Sketch > Include Library > Add .ZIP Library... and select the .zip file you just downloaded.
The second library you need to install is the Adafruit BMP085 Library, you can install this by going into Sketch > Include Library > Manage Libraries... then search for 'BMP085'.
Step 3: Wire Up the Circuit
Now you need to wire up the circuit, it is a pretty easy circuit. See the circuit schematics above.
Step 4: Build the Application for Blynk
You will need the application in Blynk so that you can receive the data and have it shown to you in the app, graphically. To build it use the pictures above.
Widgets:
- 2x Gauges
- 1x Horizontal level
Temp Gauge Settings:
- Name: Temperature
- Colour: Orange/Yellow
- Input: V5 0-100
- Label: /pin/°C
Refresh Interval: 1sec
Humidity Gauge Settings:
- Name: Humidity
- Colour: Light Blue
- Input V6 0-100
- Label: /pin/%
- Refresh Interval: 1sec
Pressure Level Settings
- Name: Pressure
- Colour: Orange/Yellow
- Input: V7 950-1050
- Flip Axis: Off
- Refresh Interval: 1sec
Step 5: Upload the Code
Now we are ready for the code. Before uploading the code you will need to make a few changes, find the line char auth[] = "YourAuthToken"; and replace YourAuthToken with the Auth Token you wrote down earlier and if you are using wifi find the line char ssid[] = "YourNetworkName"; and replace YourNetworkName with your network name and find the line char pass[] = "YourPassword"; and replace YourPassword with your Wifi password. After doing this you can now upload the code.
#define BLYNK_PRINT Serial
#include <WiFi.h>#include <WiFiClient.h>
#include <BlynkSimpleEsp32.h>
#include <SparkFun_RHT03.h>
#include <Wire.h>
#include <Adafruit_BMP085.h>
///////////////////// // Pin Definitions // ///////////////////// const int DHT22_DATA_PIN = 27; // DHT22 data pin const int FLAME_SENSOR_DATA_PIN = 32; // Flame Sensor data pin /////////////////////////// // RHT03 Object Creation // /////////////////////////// RHT03 rht; // This creates a RTH03 object, which we'll use to interact with the sensor /////////////////////////// // BMP180/BMP085 Object Creation // /////////////////////////// Adafruit_BMP085 bmp; // You should get Auth Token in the Blynk App. // Go to the Project Settings (nut icon). char auth[] = "YourAuthToken"; // Your WiFi credentials. // Set password to "" for open networks. char ssid[] = "YourNetworkName"; char pass[] = "YourPassword"; BlynkTimer timer; void sendSensor() { int updateRet = rht.update(); if (updateRet == 1) { // The humidity(), tempC(), and tempF() functions can be called -- after // a successful update() -- to get the last humidity and temperature // value float latestHumidity = rht.humidity(); float latestTempC = rht.tempC(); float latestTempF = rht.tempF(); float latestPressure = bmp.readPressure()/100; Blynk.virtualWrite(V5, latestTempC); Blynk.virtualWrite(V6, latestHumidity); Blynk.virtualWrite(V7, latestPressure); } else { // If the update failed, try delaying for RHT_READ_INTERVAL_MS ms before // trying again. delay(RHT_READ_INTERVAL_MS); } } void setup() { // Debug console Serial.begin(9600); Blynk.begin(auth, ssid, pass); // You can also specify server: //Blynk.begin(auth, ssid, pass, "blynk-cloud.com", 80); //Blynk.begin(auth, ssid, pass, IPAddress(192,168,1,100), 8080); rht.begin(DHT22_DATA_PIN); if (!bmp.begin()) { Serial.println("Could not find a valid BMP085/BMP180 sensor, check wiring!"); while (1) {} } // Setup a function to be called every second timer.setInterval(1000L, sendSensor); } void loop() { Blynk.run(); timer.run(); }
Step 6: Finished
Well done, the circuit is now complete and can now be placed in a location where it is powered and will send temperature, humidity and pressure data to your phone!
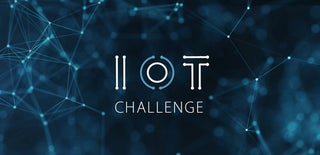
Participated in the
IoT Challenge