Introduction: Interfacing a Digital Micrometer to a Arduino & VGA Monitor
Update: Igaging Origin Series use a Mitutoyo cable and output the Mitutoyo 52 bit datastream. The code and schematics below work with SPC Digimatic Calipers, Micrometers, Dial indicators, and Scales from both companies.
Discuss a variety of manufacturer's data formats and interfacing for all microcontrollers and SPC enabled measuring systems, like scales, micrometers, calipers, dial indicators, and more.
https://groups.yahoo.com/neo/groups/spc_dro/
We had a project that required connection to a digital micrometer with a data output jack. The idea was to connect a microcontroller to the micrometer, to read the measurements and make decisions based on the readings. The micrometers that we used are made by Mitutoyo, and have a funky 52 character data stream in reverse bit order. The microcontroller we chose is the Arduino, and we used a 4D systems uVGA-II to take serial output from the Arduino and display it on a VGA monitor.
Breadboard
male shrouded header to fit mitutoyo cable - https://amzn.to/2SIIeSL
PN2222A - https://amzn.to/2Mk8XEf
(2) 10k Ohm Resistors - https://amzn.to/2YnbCU7
6 pin male header (wires to Arduino) - https://amzn.to/32VdaEf
optional momentary push-button switch - https://amzn.to/2JWBeyS
Major Components:
Mitutoyo 293-335 Coolant Proof LCD Micrometer, Friction Thimble, 0-1"/0-25.4mm Range, 0.001mm/0.00005" Graduation, +/-0.00005" Accuracy, SPC Output
and
Mitutoyo 05CZA662, Digimatic Cable, 40", With Data Switch for Coolant Proof Micrometers
OR
OR
Mitutoyo 500-171-30 Advanced Onsite Sensor Absolute Scale Digital Caliper, 0-6" Range
OR
Mitutoyo 572-211-20, Horizontal Digimatic Scale Unit, 0 -6" X .0005"/0.01mm, With Output
µVGA-II(SGC) PICASO QVGA/VGA/WVGA Graphics Controller
Arduino Mega or compatible
Protoshield recommended
2 PN2222A transistors
four 10k Ohm resistors
2x5 shrouded header
one momentary pushbutton
Step 1: Mitutoyo Cable Schematic
This is a diagram showing how the Mitutoyo cable is wired. There is a red "data" button on the micrometer end of the cable that we were not using in this application, so we decided to use it as a "menu" button.
Step 2: Connecting the Cable to the Arduino
The Arduino connects to the Mitutoyo cable with a few components. A 2x5 shrouded header that mates to the female plug on the cable, a PN2222A transistor, and two 10k Ohm resistors. One resistor is used with the PN2222A to protect the micrometer (or caliper) from excessive voltage, the other to bias the "menu" button to +5vdc.
I've included datasheets for the PN2222A and drivers for the CH340 based Arduino Nano that we now use.
Step 3: Reading the Mitutoyo Output
The heavy lifting part of the code (thanks to Mark Burmistrak for his modifications from my original), that reads the data stream, reassembles it in correct order and prints a measurement is as follows:
int req = 5; //mic REQ line goes to pin 5 through q1 (arduino high pulls request line low)
int dat = 2; //mic Data line goes to pin 2
int clk = 3; //mic Clock line goes to pin 3
int i = 0;
int j = 0;
int k = 0;
int signCh = 8;
int sign = 0;
int decimal;
float dpp;
int units;
byte mydata[14];
String value_str;
long value_int; //was an int, could not measure over 32mm
float value;
void setup() {
Serial.begin(9600);
pinMode(req, OUTPUT);
pinMode(clk, INPUT_PULLUP);
pinMode(dat, INPUT_PULLUP);
digitalWrite(req,LOW); // set request at high
}
void loop() {
digitalWrite(req, HIGH); // generate set request
for( i = 0; i < 13; i++ ) {
k = 0;
for (j = 0; j < 4; j++) {
while( digitalRead(clk) == LOW) {
} // hold until clock is high
while( digitalRead(clk) == HIGH) {
} // hold until clock is low
bitWrite(k, j, (digitalRead(dat) & 0x1));
}
mydata[i] = k;
}
sign = mydata[4];
value_str = String(mydata[5]) + String(mydata[6]) + String(mydata[7]) + String(mydata[8] + String(mydata[9] + String(mydata[10]))) ;
decimal = mydata[11];
units = mydata[12];
value_int = value_str.toInt();
if (decimal == 0) dpp = 1.0;
if (decimal == 1) dpp = 10.0;
if (decimal == 2) dpp = 100.0;
if (decimal == 3) dpp = 1000.0;
if (decimal == 4) dpp = 10000.0;
if (decimal == 5) dpp = 100000.0;
value = value_int / dpp;
if (sign == 0) {
Serial.println(value,decimal);
}
if (sign == 8) {
Serial.print("-"); Serial.println(value,decimal);
}
digitalWrite(req,LOW);
delay(100);
}
Attachments
Step 4: A Few More Auxiliary Schematics
There are a couple more external connections that we added. First, a pair of "sample" buttons (foot and finger) for taking sample measurements of 3 points of a gear before averageing and using the final average number (and rejecting if the samples are too far apart). Second, a reset circuit for the uVGA Card.
Step 5: Output to VGA
We used a uVGA-II (SGC) module from 4D to take the serial output of our Arduino, and display it on a typical vga monitor. The code to do this can be seen in the final step of this instructable. A special thanks to Rei Vilo for his assistance in this section - https://github.com/rei-vilo and http://reivilohobbies.weebly.com/
This has been replaced with the uVGA-III.
In your Arduino sketch, when you want to send data to the uvga module, use a statement like this:
uvga("N", value);
where N is the name of the variable on the uvga, and value is a variable containing the value you want passed.
At the end of your sketch (after the closing bracket of void loop) you have the following function:
int uvga(char* x, int y){
delay(50);
Serial3.print("$");
Serial3.print(x);
Serial3.println(y, DEC);
}
I'm using Serial3 on a Mega 2560, but you can use softserial and a UNO.
The code running on the uvga accepts that serial "packet", and puts the value passed into the variable that was passed, where it can then be displayed on the screen. Example code for the uvga is attached. It's a plain text file. You will need 4D's Workshop software and a USB to TTL cable to upload code to the uvga.
Attachments
Step 6: Complete Code With Measurement Sampling and Vga Output
If you would like to see how we applied this particular solution, we are using it to generate bin numbers for gear sets, based on measurements. Attached is our completed code, which was developed with the assistance of many others from the Arduino, 4D, and Arduinohome forums.
More details are available at http://tech.groups.yahoo.com/group/arduinohome/files/Arduino%20-%20VGA%20-%20Micrometer/
And http://arduinotronics.blogspot.com/2012/03/arduino-to-vga.html
The smaller file is just the code needed to display the raw readings from the micrometer. Divide the variable "num" by 1000 to get output in mm.
Step 7: Now in GFX Mode (4DGL)
We just did a major upgrade to the project. The screen output looks the same, but all the graphics processing has been moved to the uVGA-II. We bought a programming cable from 4D systems, and uploaded the new PmmC file which switches the card from SGC mode (dumb mode) to GFX (co-processor mode). This has greatly sped up the operation of the program. We also integrated a "packet" serial transmission function which sends data to the uVGA-II in serial packets, with a start symbol, a packet ID (designating which variable on the receiving end the data is destined for), and a end of packet symbol). This makes multiple destination data passing very reliable.
Details on this phase of the project can be found at http://4d.websitetoolbox.com/post?id=5858304, and we are grateful for the input from 4DSYSFAN and others.
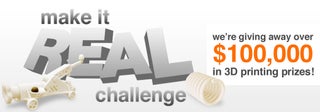
Participated in the
Make It Real Challenge