Introduction: Internet Remote Controlled Robot Car
In this Instructables, I'm gonna try to tell you how to make your robot car controlled on a website over the Internet. With this, as long as you have internet access, you can access your robot everywhere. This project has also been a learning for me to know how IoT devices work.
A little background here, All this time I want to build something that can be controlled through the internet. It's really fascinating, the idea to control something far away from it. But, in search of building it I cannot seems to find the right source to build it. Youtube and other sites always refer me to "How to build it" instead with some kind of Bluetooth or local network. But, the idea to me is already mainstream enough and what I want is something new
In my journey of building it, I also get confused why almost in Youtube learning materials there is no tutorial about internet-based communication but when so much it comes to how to set up a Bluetooth connection or having set it up in the same area network. Of course, you can say "But you can connect them through Arduino cloud or Blynk?". Of course, but to me in this project, you'll learn the basics of how to set up the connection from scratch and learn lot of things regarding remote control over a long distance.
So, after finally searching the methods of how to build a low-latency remote controlled through the Internet, I finally find the answer. Which is using WebSocket communication protocol to make the communication between the microcontroller and the server. Not only that, it can be implemented in local network as well, so you can enjoy that low-latency even further.
To build this, all you need to have is some chasis robot car, an ESP-32, and knowledge about coding in implementing the server so you can deploy them(in my case I use Heroku). So without further ado, let's go!
Note that, I assume you know basic stuff on how to set up ESP32 on Arduino UNO. Because we will use that to program our ESP32 and I will skip ESP32 setup on Arduino Uno. You can see here how to set up your ESP32 devices.
Supplies
The main component for this remote control to work over the internet is a microcontroller that has a WiFi Module on it. I use ESP32, but you can use ESP8266 or anything that has module WiFi on it.
The server and client-side control will be built using Node.js server and some basic front-end stuff. So, you need to know some basics about Javascript. But, if you knew another language program, you can make the server in your environment. In order to make it, you'll need :
The Hardware Components
- 1x 2WD Smart Car Chassis Smart Balance Robot Acrylic Case
- 1x ESP32
- 1x TB6612FNG Motor Driver
- 7 - 12V DC power supply (2x 18650 Batteries 6000mAh 3.7V)
- Two Motors and its wheels (Included on 2WD Smart Car Chassis kit)
- 2x Breadboard
The Software Components
- Arduino IDE
- Visual Studio Code
- Heroku Account
Step 1: System Overview
First, let me tell you briefly how this thing works and intended to be. So our motors will be connected through ESP32 to be controlled when there is the command to move from our website. But here's the catch, how do we achieve that? It's using WebSocket communication protocol. The great thing about WebSockets (similarly to sockets) is to create a permanent connection that enables to the server to push data when it has data at its disposal. The advantage is that the client does not need to ask for data regularly and there is no redundant network traffic. The disadvantage of the WebSockets can be seen in increasing the number of connections. With that, we can achieve real-time connection with it and do not need to ask for data regularly like HTTP or long pooling. So, on the website there will be some pad, where when we click it it will send a command to ESP32 and ESP32 will correspond it to the specific action needed.
Step 2: Build the Car Kit!
In this remote controlled car we will be build, I'm using ready made kit so that we don't have to bother about the chasis and ready to implement the aim of the project, which is build remote controlled internet robot car. It's easy to find this robot car kit and if you have difficulities assembly the kit, you can watch the assembly kit tutorial here.
Step 3: Wiring the Circuits and Assembly It Together
The wiring parts should been easy, cause we just need to connect ESP32 with the TB6612FNG driver and motors to the power supply. Here's the summary of the pin I used to connected the to each other
ESP32 :
- D13 to AIN1
- D33 to AIN2
- D27 to BIN1
- D26 to BIN2
- D32 to PWMA
- D25 to PWMB
- D23 to STBY
- GND to TB6612FNG GND
- 3.3V to VCC
- VIN to 7.4V Battery Supplies
TB6612FNG :
- VM to 7.4V Battery Supplies
- GND to 7.4V Battery Supplies
- AO1,AO2, BO1, BO2 to Motors
Make sure to ground stay all the same cause we don't want to burn the parts and STBY PIN tie to the ESP32 or nothing will work. Here you see how to connect them by seeing the schematic that I created.
Step 4: Why Used TB6612FNG?, Not L298N
If you often build robot cars using L298N you must be thinking why use this instead? Well, I'm making this project also pay attention to the cost required to make it. If we make it using L298N, I don't have the resources or budget to buy 12V batteries LiPo to connect it to the L298N driver. When I tried to connect it using 7.4V batteries, it run slow compared to when using TB6612FNG.
So, with this, we can make it cheaper to build and easy to implement. Here is a good explanation about TB6612FNG and its hookup.
Step 5: Make the Website Controller (Frontend Side)
The Websocket will be broken apart into three parts, which are the frontend side, the backend side, and the ESP32 side. In this part, we will make our website controller using only basic HTML, CSS, and javascript and deploy it to the server so we can access it everywhere. The frontend side consists of
- index.html
- style.css used for styling the web
- index.js for our DOM and managing a WebSocket connection to a server, as well as for sending and receiving data on the connection.
Where you will see that when we click the pad control it will send a command to the server to catch it, and broadcast it to the rest of the client that is currently connected(Which is ESP32).
The code can be accessed on my GitHub here on the website folder. Don't worry if when you open the index.html and see the style doesn't work, because the path is the problem and where on the backend side, we will fix how we can connect them together using express static method so in Heroku we can style them. If you want to see the result of the page now, you can change the path. But don't forget to change it back, cause we will fix it in next step
href = "style/style.css"
to
href = "public/style/style.css"
and
src = "js/index.js"
to
href = "public/js/index.js"
Step 6: Make the Server! (backend Side)
So, we are in the server. This is where we will connect our frontend side (controller) to the ESP32, managing the WebSocket server to maintain a connection to all clients that connect, when it receive data it will broadcast the command to the existing client that is currently connected to the server! We will make the backend using Node.js and with its library which is express and ws. You can first download Node.js here. After finishing the installation, you can install the library needed through your terminal in your current folder
npm install ws npm install express
ws is the WebSocket library that we need for the WebSocket and express is used for making the server. The server code can be accessed on GitHub, so if you want to know more about the code, you can read it in my code on Github, where I explain in the comment what the syntax does.
server.js source code
const PORT = process.env.PORT || 5000; // Defining the PORT we used for communication server const INDEX = '/index.html' // Page for the website const express = require('express') // Node.js Application Framework const WebSocket = require('ws') // Websocket Protocol Library // Opening up the server using basic express library const server = express() .use(express.static( __dirname + '/public')) // This is basically static, heroku does't support file storage in their //system so we have to make it static so style.css and index.js can be accessed in our website .get('/', (req,res) =>{ res.sendFile(INDEX, {root : __dirname})}) //Basic Routing when website hit '/', respond serve index.html .listen(PORT, ()=> console.log(`Listening on ${PORT}`)) // Opening or upgrade server to websocket server const { Server } = require('ws') // Implement Websocket Connection, the handskae are put in here const wss = new Server({ server }) // This will now upgrade the listener to websocket connection whenever we hit '/' page index at website // Implementing when handsake completed wss.on('connection', function connection(ws,req){ // Taking the event, when handsake completed go console.log("A Client has connected") console.log(`Now on Port ${PORT}`) ws.on('message', function incoming (data){ // Taking the event data incoming message, what to do going below console.log(data.toString('utf-8')) wss.clients.forEach(function each(client){ // If ws detect message, take all current clients connected if(client != ws && client.readyState === WebSocket.OPEN){ //Ready state constant CONNECTION = 0 | OPEN = 1 | CLOSING = 2 | CLOSED = 3 client.send(data.toString('utf8')) // If it's true some client open and sending message, send that data to all } }) }) }) // Implementing when websocket connection in this client close wss.on('close', function close(){ console.log("Web Socket Closing") }) // Which is impossible, because server always running, in this case heroku has implemented if there is no activities in // Duration of 6 hours, server will sleep
Step 7: Sign Up Heroku and Deploy
After we finish building the frontend and backend side, we now can deploy them to the server. In this instructable, we will be using Heroku service, which is free and easy to deploy. The folder that we will deploy to Heroku is website folder
For this, you will need git to push your code into Heroku. You can download git here.
- Go to Heroku Website: https://www.heroku.com/
- Sign up
- On the dashboard page, click on New and then Create new app
- Type in a name for the app, it can be anything. You might need to type in something different and available. Consequently, hit the “Create app” button.
- Download and install the Heroku CLI.
- Go to your dashboard app and deploy page
- Follow the instruction on how to Deploy them using Git
- Open App to see your Website Running!
If you see the site is running, congratulation now your website is running and online, take the website URL because you will need it for connecting the WebSocket from our website to our ESP32.
Step 8: Programming Your ESP32
Now open up your Arduino Uno. Here, we will be programming on how to connect to your network and connect it to our website server that we just deployed.
- Download the WebSocket library source code at Github: https://github.com/gilmaimon/ArduinoWebsockets
- Open ESP32Client.ino in Arduino IDE available at here.
- Connect your ESP32 to your PC
- Select your ESP32 Dev Module Board type and Port at Tools Menu
- Upload
Noted that, you need to replace your SSID and password to the respective network that you using. Remember the last step where I said now you have successfully uploaded your server? Now take the URL, and paste them into the websockets_server. Mine was https://esp32-ws.herokuapp.com/. So,
websockets_server = "ws://esp32-ws.herokuapp.com/"
Just wait for the upload to be completed, now you are ready to be connected!
Attachments
Step 9: Internet Robot Car Completed!
It's done! If you follow each step, now you can made your own internet robot car. All that left is to connect the power supply to the breadboard, wait for some time to connect to your WiFi and connect to server and you are ready to remote control it over the internet xD
Step 10: Issues and Improvement
Well, there are some issues regarding this type of control. Well, I'm happy with the results but I think it can be improved even further. You can see data in the image above, where the latency is reached about 500-700ms but my target is 200ms below to reach a real-time connection. Other issues such as :
- Websocket close connection if 55s idle or don't send data to ESP32. This means that we need to restart the device so we can connect back again to the server. This issue comes from Heroku policy where after the initial response, each byte that we sent will have resets a rolling 55-second window. Where if no data is sent within 55s limit time, then the connection will be terminated.
- All this time, I check whether our ESP32 connects to our server through Heroku server log commands, I think it can be made that if our ESP32 successfully connects to our server, we can add some green LED for visual agreement in the breadboard.
- We can make the website prettier, but I'm not a styling master so this has been an issue for me :')
You can see the result of the implementation where I use a dummy web to make the request to the robot car. It's text-based control, here.
Overall this is a good experience and learning material for me. Although the wiring and the look of robo car need some improvement, mb. If you could have any improvements to this project, feel free to make an impact in the comments below. If you have any problems regarding difficulties in making the project also feel free to reach me in the comments below. I hope you enjoy reading this. Adios!
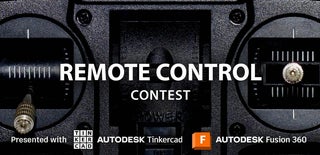
Runner Up in the
Remote Control Contest