Introduction: Intro to Python
Python is a very useful and flexible computer programming language created by Guido van Rossum. Python is also an application on the Raspberry Pi that interprets the code you write into something the Raspberry Pi can understand and put to use. On your desktop computer you would need to install Python, but it already comes bundled with Raspbian so it's ready for you to use.
You may think that Python is named after the type of snake but it was actually named after the Monty Python's Flying Circus TV show. For those of you who don't know, this was a British comedy show that was first broadcasted in the 1970s. I encourage you to watch the Ministry of Silly Walks sketch :)
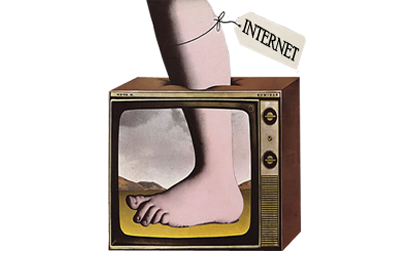
The above image was created in a Terry Gilliam fashion by Allison Parten and found under the creative commons attribution license.
Compared to other computer languages, Python is easy to read and understand which makes it great for beginners. But make no mistake, Python is also very powerful and is used to make complex, creative and commercial software. These reasons and more make Python the number one language to start with when writing programs for the Raspberry Pi (although several languages can be used).
Like any language, Python has a grammar with rules for how to order words and punctuation. The term used for these fundamental rules of structuring a language is called syntax. I will point out Python syntax throughout this lesson along with example code for your to try out. In a class of this size, it's impossible to go over everything Python can do, but this lesson and the next, titled Use theGPIOs and Go Further with Python, will get you started with your first programs. As you read and work through the examples, you’ll learn about some of the fundamental principles of Python and programming in general.
Step 1: Python 2 or 3?
There are two versions of Python that are currently being used and taught. While I will not get into the nitty-gritty of how they are different, it is important to know that there is a difference. To know this is to save yourself a lot of head scratching when examples found online don't work.
Python 2 is slated to be officially retired by 2020 as stated by the Python Software Foundation. They say this on their site, "Being the last of the 2.x series, 2.7 will have an extended period of maintenance. The current plan is to support it for at least 10 years from the initial 2.7 release. This means there will be bugfix releases until 2020." There is even a countdown clock all in good fun or perhaps so people can plan a going away party to finalize the end of Python 2.
In this class, we use both. Normally, I would look to the future and use Python 3 but something happened while creating examples for this class. A piece of software I wanted to use for the final Python program had not been updated to support Python 3 yet. Which actually perfectly illustrates my point on how it's worth mentioning both at the moment.
Step 2: Python Interactive Vs Script
When working with the Python application, you have two modes to choose from: interactive and script.
Interactive mode uses the Python shell to interpret Python code instantly after it's typed and you hit Enter. It's great for learning and testing pieces of a program in. We will co over a couple of ways to access the Python shell in this lesson.
When you write a python script, also called a program, you do not use the interactive shell but instead a text editor. This way you can save, edit, and later run multiple lines of Python code when you need.
Step 3: IDLE: Interactive
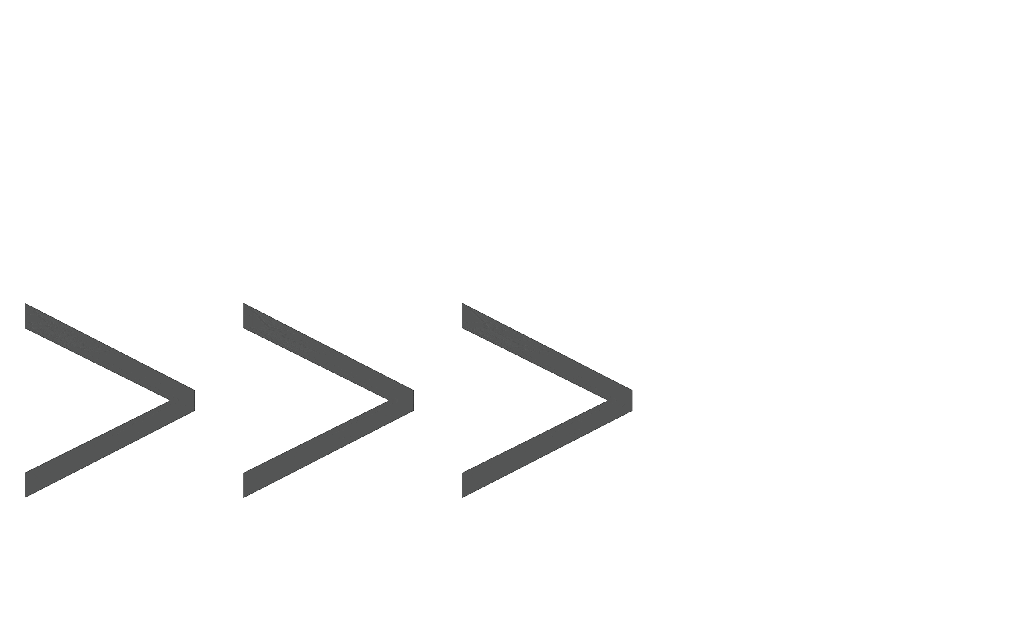
With the installed Python software comes Python's standard development environment called IDLE (Integrated DeveLopment Environment). This is where you will get started writing your first Python programs!
There are two parts to IDLE:
1) The Python shell window, which gives you access to Python in interactive mode.
2) A file editor that lets you create and edit existing Python scripts, also referred to as script mode.
Open Python 3 (IDLE) from Menu > Programming. The window you see is called the Python interpreter or shell window. The three greater-than characters ">>>" are called the prompt. When you see the prompt that means Python is waiting for you to tell it to do something. Let's give it some code!
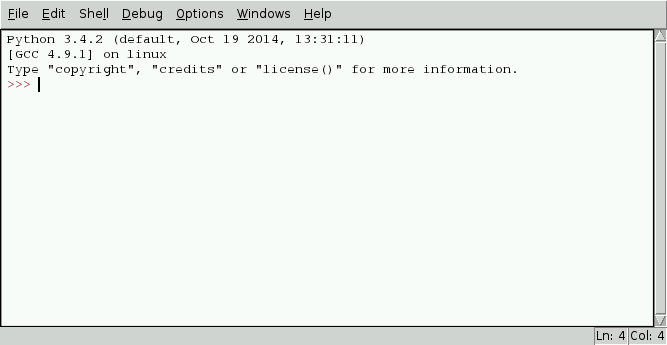
Type the following statement to give a shout out to the city you live in. I live in San Francisco so my statement be:
print("Hello, San Francisco!")
Hit enter and whatever you put in between the quotes will print in the shell under the prompt. The print() statement differs between Python 2 and Python 3. In Python 2 parenthesis are not used and looks like this:
print "Hello, San Francisco!"
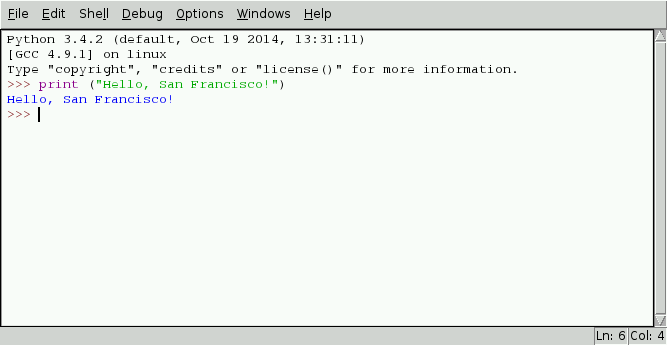
You have just performed the basic "hello, world" of Python but with a small (super small) twist. Let's stop here and identify what it is you just typed.
Functions
Print() is known as a function. A function cuts down on repetition and helps to keep a program organized by executing a block of code each time it is called. When you type print(), you call the print function, which then runs lines of code behind the scenes that gives the computer instruction to display the words you put between the parenthesis. Print() is a built-in function you can call anytime in Python but you can write your own functions too.
Let's make the interaction between you and Python more dynamic by adding user input. In a new window, type the following:
name = input("Hello, what's your name? ")
The input() function takes user input from the keyboard and gives you the option of prompting the user with a message. In this case, that message is a greeting and question asking the user (you) what your name is. After hitting enter, the question will print and wait for your response. Go ahead and answer with your name.
The name = to the left of the input() function is called a variable.
Variables
Like functions, variables are also a core element of any programming language. A variable acts like an empty container that you can put a piece of data in. When you drop data in, you give it a name as if you are writing it on the outside of the container. The unique name you give it can then be used to reference the data inside throughout your program. You can name a variable almost anything, but it should be as descriptive as possible. This makes your program easier to understand when you read it later. Data stored inside a variable can change; you will see an example of this in the next lesson.
Your name was stored in the variable called name (try calling it something else). You can now use the name variable in the print() function and add it to a message using the "+" character:
print("It's nice to meet you, " + name)
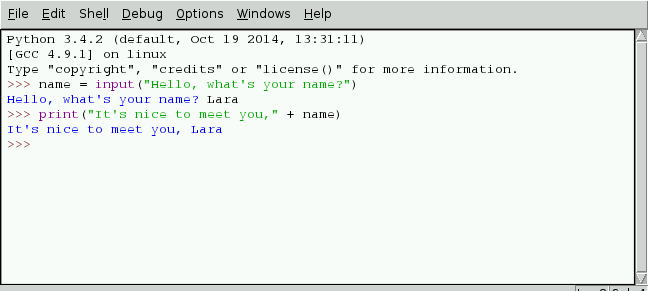
Let's keep practicing getting user input, storing it into variables, and printing the data to simulate a conversation between you and your computer.
city = input("What city do you live in? ")
print("I have heard of " + city + ". What do you think of " + city + “, ” + name + "?")
Calling the input() function on its own still waits for user input but does it without printing a message.
answer = input()
Because computers do our bidding, I will make the computer agree. You can make it disagree if you like. That's the great thing about programming, it's up to you.
print("I agree with you. " + answer)
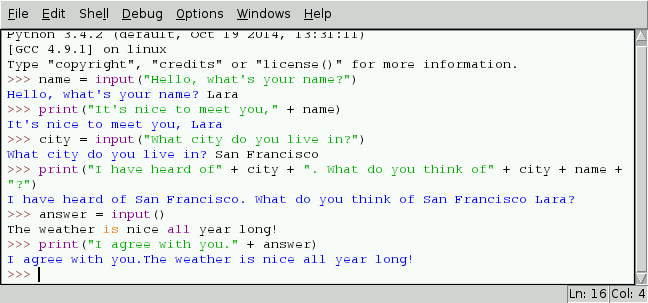
It's ok if you see an error at any time in the Python shell. Your data will stay stored in your variables as long as you don't close the session.
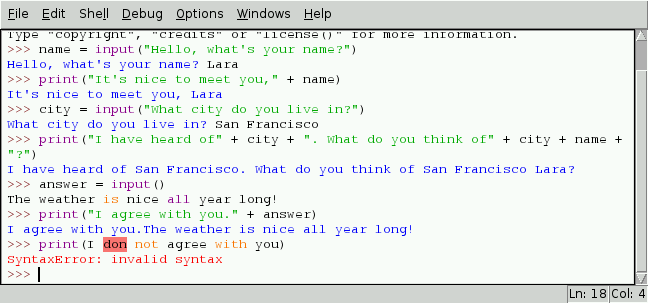
The interactive shell is perfect for testing commands and seeing what works. But it does not save your program so you can run it later. Your session can be saved but Python also saves the prompts, errors, and everything else you see in the shell window. These will all cause errors if you try to have Python run it as a program later on.
To experiment (and as a class exercise), add two more lines to this program to continue the conversation between you and your computer. Create a variable and print out a statement using your new variable. Take a screenshot or save what you have done in the shell for reference. You will use it in the next step.
Step 4: IDLE: Script
Unlike the shell, an editor is used so you can save and edit your Python programs. Although there are several editors you can use, IDLE comes with one so let's start with that.
Create a new file in IDLE by pressing Ctrl + N or navigate to File > New.
Notice how the window you see doesn't have the ">>>" prompt. You are now in the editor and ready to write, save, and run a Python program. Write out the program you created in the shell including the two new lines you came up with in the last section. As an example, here is my final script:
name = input("Hello, what's your name? ") print("It's nice to meet you, " + name) city = input("What city do you live in? ") print("I have heard of " + city + ". What do you think of " + city + “, ” + name + "? ") answer = input() print("I agree with you, " + answer) favSpot = input("What is your favorite spot? ") print("Never been, but I would love to go sometime to " + favSpot)
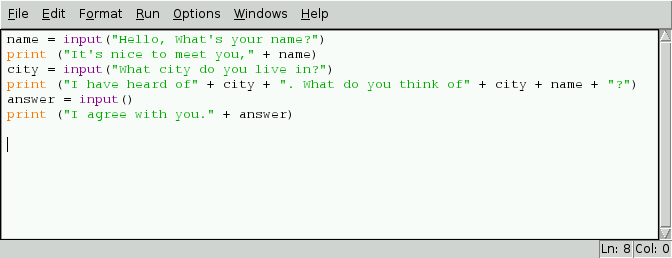
Save it as city.py. The default location is your home directory.
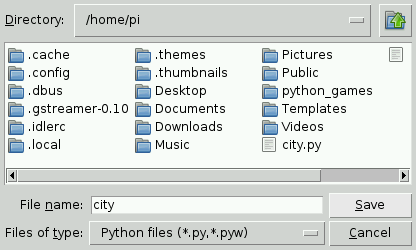
Step 5: Running a Python Program
Your program is saved and ready to run. There are a couple different ways to run a Python program on the Raspberry Pi. Let's go over two. Choose one to run your program and take a screenshot after you have finished your conversation.
1) Run from IDLE
Press F5 or go up to the toolbar and click Run > Run Module. Python will print the results in the shell window. To stop the program press Ctrl+F6 or go to Shell > Restart Shell.
2) Run from Linux Shell
Programs saved to the Raspberry Pi can run from the all powerful Linux shell, too. To run a Python program from the command-line, your program needs to be in the current working directory. LXTerminal starts you in pi's home directory where python automatically saves, so you should already be in the folder with your file. To check, type:
ls
You should see your saved city.py program listed.
To run a Python program command-line style type python3 plus the name of your script:
python3 city.py
For a script written in Python 2 you would use python instead of python3:
python nameOfScript.py
The first line of the program will execute waiting for you to type in your name. It will continue to execute from top to bottom until it gets to the last print() function.
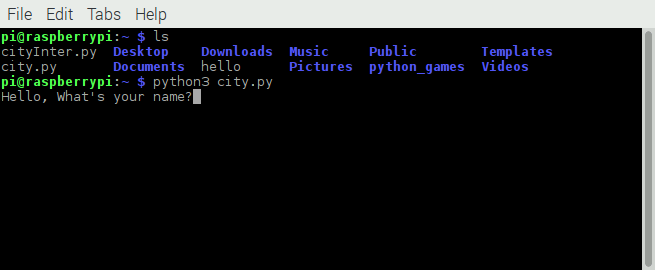
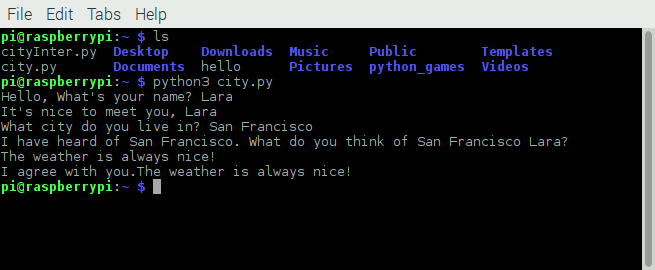
Stopping a Python Program
To stop a running Python program in command-line press Ctrl + Z.
Step 6: Python + Linux Shell
Although Python will be the main language you use to program the Raspberry Pi, sometimes you will want to use a command-line tool or application to get something accomplished. You can combine command-line and Python to use your favorite commands using a module called os.
A module is a collection of prewritten code that you can use to add functionality to your programs. Using a module can also save you the hassle of figuring out how to write some pretty complex stuff. For example, say you wanted to track planets and study their orbits with your Raspberry Pi. Instead of figuring out the complex math behind pinning down the position of the Sun, you can use a module* that already has that figured out.
In order to use os, you will need to first import it. Go ahead and follow along in the Python shell:
import os
Load a command you want to run in the Linux shell into a variable. Here we are using the command-line video player application omxplayer to play a test video that comes with Raspbian:
playVideo = "omxplayer /opt/vc/src/hello_pi/hello_video/test.h264"
Use os.system() to send the command the Linux shell:
os.system(playVideo)
* There really is a Python module that can track planets, it's called PyEphem.
Step 7: Take a Photo and Play Audio With Python
Picamera
So far you have used Raspistill a command-line application to take a series of photos with. There is a Python module available called Picamera you can use instead that also has more features that will be helpful when building your final photo booth program. The steps below may cover your screen, so keep in mind that to stop the process, type Ctrl + F6.
Open up the Python shell and type the following lines:
import picamera
camera = picamera.PiCamera()
camera.resolution = (640, 480)
camera.start_preview()
Use the live preview to aid in positioning your camera to capture a photo of yourself or your desk. The camera preview may take over most of your screen. Repeat the first three commands, then skip to capturing and saving an image:
camera.capture('testImage.jpg')
Try changing the brightness of the camera using the brightness attribute. It can be set to any number between 0 and 100 and the default is 50. Try setting it to another number, then capture a new photo to replace the first:
camera.brightness = 60
camera.capture('testImage.jpg')
Make sure to take some time to check out the Picamera documentation.
Using os module
If you want to use a command-line application like Raspistill you can use the os module. Type Cmd + F6 to quit the running process and release the camera from Picamera. Then type the following commands:
import os
takePhoto = "raspistill -o testImage.jpg"
os.system(takePhoto)
Pygame.mixer
A simple and robust way of playing sound files is to use Pygame. Pygame is a set of Python modules that comes bundled with Raspbian so there is no need to install it.
Pygame is popular and fun so there is a lot of support and development around it. Make sure to check out the website for examples and documentation. Besides being used to create games, it's an easy way to play sounds, display images and more. To play sounds use the Sound object in the pygame.mixer module.
Open the Python 3 interpreter and type the following lines:
import pygame.mixer
from pygame.mixer import Sound
pygame.mixer.init()
bass = Sound('bass3.wav')
bass.play()
Step 8: Using Python Outside of IDLE
Desktop Editors
IDLE is a great way to start writing Python programs but you do not have to use it. You can create a Python program using any text editor as long as it's saved with .py at the end. IDLE is one example of a desktop graphical text editor. Another one that comes bundled with Raspbian is called Leafpad. You can find Leafpad under Accessories in the start Menu.
Command-Line Editor
You have already been introduced to Nano the command-line text editor. Just like a graphical editor, you can use nano to create a script. After opening Nano save the new file with the .py suffix. Saving it as a python file first will ensure the editor provides python syntax highlighting as you type.
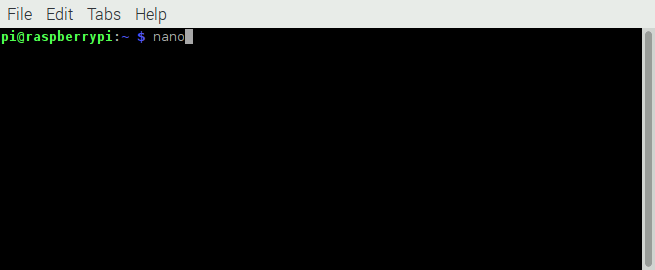
Without syntax highlighting before saving file name with .py suffix.
With syntax highlighting.
Python Interpreter from Command-line
IDLE's not the only place you can use the interactive Python interpreter. It can be invoked from the Linux shell too! Simply type:
python3
Or for the Python 2 interpreter:
python
To exit the interpreter press Ctrl + D or type:
quit()
Step 9: Screenshot of Program
Show off your Python programming powers! Upload a screenshot after your final city.py program finishes running and tell us if you ran it from IDLE or the Linux shell.