Introduction: Iron Man's Arc Reactor & Voice Home Automation
Why Did We Build This?
I've always been a huge fan of Tony Stark aka Iron man. I was inspired to make a smart voice interaction device that could help me control the light, and fan or to dispense the accessories in the hotel. In today's world, Voice control has numerous applications on accessibility to various tasks. This project is used to interact with digital devices using voice recognition which enables you to use voice commands to perform actions using the trained models. With this solution, we can improve the traditional way of dispensing accessories such as soap, paste, brush, towel by the server. These can be automated using a voice AI.
Step 1: Hardware Build
Hardware components
- MATRIX Labs MATRIX Voice × 1
- MATRIX Labs MATRIX Creator × 1
- Raspberry Pi 3 Model B+ × 1
- SG90 Micro-servo motor × 2
- Jumper wires (generic) × 10
Software apps and online services
- Snips AIR
Step 2: Getting Started With MATRIX Voice (Content From MATRIX Labs)
MATRIX Voice is a development board for building sound driven behaviors and interfaces. MATRIX Voice was built with a mission to give every maker, tinkerer, developer, and student around the world a complete, affordable, and user-friendly tool for simple to complex Internet of Things (IoT) voice app creation. With the ESP32 module, you are able to run the MATRIX Voice standalone, communicate with other devices, and transmit voice data via Bluetooth and/or WiFi.
Components present in this Matrix Voice board are listed below
- FPGA
- Xilinx Spartan 6 XC6SLX9
- Microphone Array
- 8 MEMS MP34DB02 audio sensor digital microphones
- Everloop18 RGBW LEDs
- RAM
- 64 MByte 132MHz SDRAM
- FLASH
- 64Mbit Flash Memory
- ESP32 (MCU w/WiFi/BT)
- Wifi 2.4Ghz 802.11bgn - BT 4.0 LE - Microcontroller Tensilica Xtensa dual-core 32-bit LX6
Hardware: Raspberry Pi B+ or newer, Raspberry Pi Zero
Software: Raspbian, Debian
Step 3: Interfacing With MATRIX Voice (Basic Project)
- Power the kit by the default power adapter in the kit or use a 5V-2A DC adapter with a Micro USB connector.
- The next step is installing the Snips assistant into the Raspberry Pi. To save your setup time, the MicroSD card in the kit has been flashed with the fully functional system image, which means the Snips assistant is ready for your trigger words.
- We strongly suggest you study the step by step installation guide by visiting: Iron Man Arc Reactor with MATRIX Device and Snips.ai by the Team MATRIX Labs.
- Trigger the assistant by saying “JARVIS”, and follow the command.
Python script for Rainbow LED sequence
/*
* Everloop rainbow example
*/
/////////////////////////
// INCLUDE STATEMENTS //
///////////////////////
// System calls
#include <unistd.h>
// Input/output streams and functions
#include <iostream>
// Included for sin() function.
#include <cmath>
// Interfaces with Everloop
#include "matrix_hal/everloop.h"
// Holds data for Everloop
#include "matrix_hal/everloop_image.h"
// Communicates with MATRIX device
#include "matrix_hal/matrixio_bus.h"
int main() {
////////////////////
// INITIAL SETUP //
//////////////////
// Create MatrixIOBus object for hardware communication
matrix_hal::MatrixIOBus bus;
// Initialize bus and exit program if error occurs
if (!bus.Init()) return false;
/////////////////
// MAIN SETUP //
///////////////
// Holds the number of LEDs on MATRIX device
int ledCount = bus.MatrixLeds();
// Create EverloopImage object, with size of ledCount
matrix_hal::EverloopImage everloop_image(ledCount);
// Create Everloop object
matrix_hal::Everloop everloop;
// Set everloop to use MatrixIOBus bus
everloop.Setup(&bus);
// Variables used for sine wave rainbow logic
float counter = 0;
const float freq = 0.375;
// 10 sec loop for rainbow effect 250*40000 microsec = 10 sec
for (int i = 0; i <= 250; i++) {
// For each led in everloop_image.leds, set led value
for (matrix_hal::LedValue &led : everloop_image.leds) {
// Sine waves 120 degrees out of phase for rainbow
led.red =
(std::sin(freq * counter + (M_PI / 180 * 240)) * 155 + 100) / 10;
led.green =
(std::sin(freq * counter + (M_PI / 180 * 120)) * 155 + 100) / 10;
led.blue = (std::sin(freq * counter + 0) * 155 + 100) / 10;
// If MATRIX Creator, increment by 0.51
if (ledCount == 35) {
counter = counter + 0.51;
}
// If MATRIX Voice, increment by 1.01
if (ledCount == 18) {
counter = counter + 1.01;
}
}
// Updates the LEDs
everloop.Write(&everloop_image);
// If i is 0 (first run)
if (i == 0) {
// Output everloop status to console
std::cout << "Everloop set to rainbow for 10 seconds." << std::endl;
}
// If i is cleanly divisible by 25
if ((i % 25) == 0) {
std::cout << "Time remaining (s) : " << 10 - (i / 25) << std::endl;
}
// Sleep for 40000 microseconds
usleep(40000);
}
// Updates the Everloop on the MATRIX device
everloop.Write(&everloop_image);
// For each led in everloop_image.leds, set led value to 0
for (matrix_hal::LedValue &led : everloop_image.leds) {
// Turn off Everloop
led.red = 0;
led.green = 0;
led.blue = 0;
led.white = 0;
}
// Updates the Everloop on the MATRIX device
everloop.Write(&everloop_image);
return 0;
}
You can find the video of the example using the MATRIX Voice interaction kit powered by SNIPS.
Tutorial from Team MATRIX Labs helped me to do this project.
Step 4: Extended Project
J.A.R.V.I.S (Just A Rather Very Intelligent System) This project demonstrates the use of different technologies and their integration to build an intelligent system which will interact with a human and support in their day to day tasks.
The Parts which we need are :
- Arduino
- Relay Breakout board
- Bluetooth Module - HC-05
- Jumper Cables
Step 5: BT Connections
*Arduino -> BT MODULE*
TX -> RX
RX -> TX
VCC -> 3.3v
GND -> GND
Step 6: Relay Connections
*Arduino -> Relay Board*
IN1 -> D2
IN2 -> D3
IN3 -> D4
IN4 -> D5
VCC -> VCC
GND -> GND
Step 7: The Code
You can find the sample code below.
//Coded By: Angelo Casimiro (4/27/14)
//Voice Activated Arduino (Bluetooth + Android) //Feel free to modify it but remember to give creditString voice;
int led1 = 2, //Connect LED 1 To Pin #2
led2 = 3, //Connect LED 2 To Pin #3
led3 = 4, //Connect LED 3 To Pin #4
led4 = 5, //Connect LED 4 To Pin #5
led5 = 6; //Connect LED 5 To Pin #6
//--------------------------Call A Function-------------------------------//
void allon()
{ digitalWrite(led1, HIGH);
digitalWrite(led2, HIGH);
digitalWrite(led3, HIGH);
digitalWrite(led4, HIGH);
digitalWrite(led5, HIGH); }
void alloff()
{ digitalWrite(led1, LOW);
digitalWrite(led2, LOW);
digitalWrite(led3, LOW);
digitalWrite(led4, LOW);
digitalWrite(led5, LOW); }
//-----------------------------------------------------------------------//
void setup() {
Serial.begin(9600);
pinMode(led1, OUTPUT);
pinMode(led2, OUTPUT);
pinMode(led3, OUTPUT);
pinMode(led4, OUTPUT);
pinMode(led5, OUTPUT); }
//-----------------------------------------------------------------------//
void loop() {
while (Serial.available()){ //Check if there is an available byte to read
delay(10); //Delay added to make thing stable
char c = Serial.read(); //Conduct a serial read
if (c == '#') {break;} //Exit the loop when the # is detected after the word
voice += c; //Shorthand for voice = voice + c
} if (voice.length() > 0) {
Serial.println(voice);
//-----------------------------------------------------------------------// //----------Control Multiple Pins/ LEDs----------//
if(voice == "*all on") {allon();} //Turn Off All Pins (Call Function)
else if(voice == "*all off"){alloff();} //Turn On All Pins (Call Function) //----------Turn On One-By-One----------// else if(voice == "*TV on") {digitalWrite(led1, HIGH);}
else if(voice == "*fan on") {digitalWrite(led2, HIGH);}
else if(voice == "*computer on") {digitalWrite(led3, HIGH);}
else if(voice == "*bedroom lights on") {digitalWrite(led4, HIGH);}
else if(voice == "*bathroom lights on") {digitalWrite(led5, HIGH);} //----------Turn Off One-By-One----------//
else if(voice == "*TV off") {digitalWrite(led1, LOW);}
else if(voice == "*fan off") {digitalWrite(led2, LOW);}
else if(voice == "*computer off") {digitalWrite(led3, LOW);}
else if(voice == "*bedroom lights off") {digitalWrite(led4, LOW);}
else if(voice == "*bathroom lights off") {digitalWrite(led5, LOW);}
//-----------------------------------------------------------------------//
voice="";}} //Reset the variable after initiating
Step 8: Application
For now, we will use the ready-made application. In the following days, I will publish the application once after the certificates are processed.
BT Voice Control for Arduino created by SimpleLabsIN: https://apkpure.com/bt-voice-control-for-arduino/robotspace.simplelabs.amr_voice/download?from=details
Step 9: Working of This Project
If you faced any issues in building this project, feel free to ask me. Please do suggest new projects that you want me to do next. Share this video if you like.
Blog - https://rahulthelonelyprogrammer.blogspot.in/
Github - https://github.com/Rahul24-06
Happy to have you subscribed: https://www.youtube.com/c/rahulkhanna24june?sub_confirmation=1
Thanks for reading!
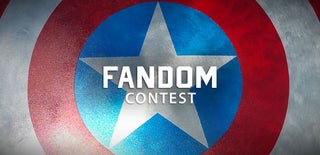
Participated in the
Fandom Contest