Introduction: Low-level Text and Graphics on a TFT SPI Screen With Raspberry PI Zero W
Browsing on Aliexpress, I found this cheap 3'5 TFT SPI touch screen, interested in using it in future projects, I bought one.
As its installation and use were not seamless, I decided to make an instructable about it. I have not used the touch part yet, but that may come later. This is my first instructable and English is not my native language, so please be indulgent.
Supplies
What you will need :
- 3'5 TFT SPI display
- Raspberry pi zero W ( 2B and 3B have the same GPIO and can also be used), with an SD card and power supply.
Step 1: Prepare the Raspberry
This is already well explained here or here.
Take the Raspberry Pi OS Lite, we don't need any desktop here.
You can plug in a USB keyboard and an HDMI screen and go on to the next step, but I prefer to work through SSH, so I enable SSH and connect the raspberry to my WIFI without a monitor, as explained here or here.
At your first login, run
sudo raspi-config
Configure your timezone and hostname, but mainly, in "Interface Options", enable the SPI interface.
Reboot.
sudo reboot
It's often a good idea to upgrade the raspberry before installing a new component, so begin with the two following commands :
sudo apt update
sudo apt upgrade
It will probably upgrade a lot of things.
Reboot again when it's done.
sudo reboot
Step 2: Plug the Screen to the Raspberry
That's pretty straightforward.
Step 3: Install the Driver
Documentation for this step can be found here.
Logged as pi, install git :
sudo apt-get install git
clone the git repository containing the driver :
git clone https://github.com/goodtft/LCD-show.git
Run the driver installation
chmod -R 755 LCD-show
cd LCD-show/
sudo ./LCD35-show
It will install some packages and should reboot at the end.
After the boot, you should see the boot messages on your LCD screen. So far so good: it means your screen is working correctly.
If you have an HDMI screen also connected to the PI, you will see that the HDMI screen display is replicated on the LCD.
That is not what we want. To change this, edit your RC.local file
sudo nano /etc/rc.local
Find a line containing "fbcp & " and comment on it by putting a "#" at the beginning of the line. There may be a sleep just before the fbcp, comment on it.
Reboot.
sudo reboot
After the reboot, the LCD screen should remain black.
Step 4: Display Text and Bitmaps Using the Framebuffer
There are probably other simpler ways to do it, but I chose the low-level approach by writing directly to the screen's framebuffer thanks to this tutorial.
You can list the framebuffers of your raspberry by typing
ls -la /dev/fb*
You should see fb0, your HDMI screen, and fb1, your SPI screen.
The frame buffer is a memory buffer mapping all the pixels of the screen. Our screen's resolution is 480x320, and each color is coded on 2 bytes, so it's simply a 480x320x2 bytes buffer, so we have to write the pixel color at the proper buffer position to have this pixel displayed on the screen.
The fbcp command we commented on in the previous step was "simply" copying fb0 to fb1.
As said, the screen displays only 65536 colors, so each pixel is coded on 16 bits, using the RGB565 format ( 5 bits for red, 6 bits for green, 5 bits for blue ), here is the way to convert standard 24 bits RGB to 16 bits RGB565 :
uint16_t RGB16(uint8_t r, uint8_t g, uint8_t b )
{
uint16_t c = b>>3; // blue
c |= ((g>>2)<<5); // green
c |= ((r>>3)<<11); // red
return c;
}
I found a nice 8x8 pixels font here and made a few executables to use in scripts. You'll find my code attached, or you can simply clone it from GitHub and build the various executables :
git clone https://github.com/SamuelF94/fbdisplay
cd fbdisplay
make
the make will give you
- clearscreen => fills the framebuffer with 0
- fbtext: displays a simple text
- fbbmp: reads a 480x320 24 bits bitmap and displays it on screen.
you can try
./fbtext --t "Hello !" --f 6
./clearscreen
./fbbmp sample.bmp
./clearscreen
Step 5: Display Animated GIFs
I found the beautiful work made by Larry Bank, I forked his repository to remove his SPI library because I only needed the framebuffer part.
You can clone the code from GitHub and build it :
git clone https://github.com/SamuelF94/gif_play
cd gif_play
make
The make will give you an executable named GP, and there is a sample animated gif in the repository you can try the display by typing :
./gp --c --in sample.gif
Step 6: That's It
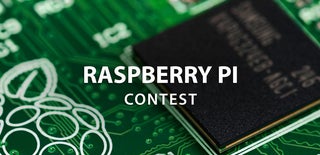
Participated in the
Raspberry Pi Contest