Introduction: Make a RC Car You Can Control With Any Remote
In this instructables, I'm going to show you how you can make an easy car you can make yourself.
The best thing about this car is that, with slight modification, you can control it with almost any remote control you can imagine
For example, you can control it using:
- An App (Which I will show you soon ;) )
- A RC remote control (which I'm using here)
- A IR remote
- ...
Lets get started!
Step 1: Parts
For making the car, you'll need several parts.
- A car kit, with the motors and the frame
- A H-bridge to control the motor. (speed and direction)
- A arduino, I'm using a mega here, but you can use any type of arduino
- Some type of remote control (I'm using the Spektrum DX6i, but you can use almost any remote)
Optional:
- A 12V to 6V converter (Only if you're using a 12V battery instead of the 4AA cells)
- A HC-05 Bluetooth module (For controlling the car for a phone or PC)
- Other accessories
Step 2: What's Included in the Car Kit
With the car kit you get many parts. They are:
- 4 wheels with rubber tires
- Acrylic top and bottom plate
- 4 Motors with gearboxes, so the output shaft turns slower than the motor shaft, but with more torque.
- Battery box for 4AA batteries, I'm not using it, because I rather use a lipo battery
- 2 wires for every motor (red and black)
- Enough screws and nuts for putting it together and some extra for mounting the controller
- 6 Spacers for between the top and bottom plate
- 8 Motor holders, 2 for each motor
- 4 Encoder disks for if you want to measure the motor speed
- A screwdriver
- A build instructions
Step 3: Assembling the Car
The first step is to peel of the protecting paper from all the acrylic pieces. (Top and bottom plate, motor mounts, encoder disks)
After that, you can start assembling the chassis using the pictures on the instructions. Leave the top plate off.
After that, you can shorten the wires, and solder them crosswise to the four motors. (As seen in the last picture)
Step 4: Installing the Electronics
First place the H bridge between the 4 motors and connect it to the motors.
After that, you can connect the voltage regulator, if used, otherwise connect the battery pack to the H-bridge, to VCC and GND.
Also add 2 wires (red and blue) for powering the arduino. You can use the 5V regulator on the H bridge.
Also add for female to male or female to female jumper wires to the H bridge control pins.
if you are using a lipo battery, connect a battery connector to the voltage regulator input.
Now screw on the top plate. And mount the arduino on top.
Connect the red and blue wire to 5V and GND of the arduino.
Also connect the 4 control pins from the H bridge to pin 10 - 13.
This is the part for the car. The next part is for the remote. If you use another sort (bluetooth,...) , you need to change here.
For a RC controller, just connect the 5V and GND to a 5V and GND connection of the arduino. Also connect channel 2 and 3 (for driving) to pins 3 and 4.
That's it for the hardware side. Now we go to the software side.
Step 5: Arduino Software
The software of the arduino isn't that difficult. I've added comments, but if something isn't clear, please let me know.
#define Motor1_Dir 13 // Motor 1 direction control pin #define Motor2_Dir 11 // Motor 2 direction control pin #define Motor1_PWM 12 // Motor 1 speed control pin #define Motor2_PWM 10 // Motor 2 speed control pin int channel[4] = {0, 0, 0, 0}; // A variable to save the remote control values int pwm[4] = {0, 0, 0, 0}; // A variable to save the remote values from -255 to 255 int prev_pwm[4] = {0, 0, 0, 0}; // A variable to check if the state changed int pwm_m1 = 0; // A variable to save the speed of the first motor int pwm_m2 = 0; // A variable to save the speed of the second motor int reverse = 0; // A variable to check if the car needs to drive backwards int prev_reverse = 0; // A variable to check if the state of reverse changed const int pins[4] = {2, 3, 4, 5}; // A const variable for the pins of the remote void setup() { pinMode(2, INPUT); //Ch1 pinMode(3, INPUT); //Ch2 pinMode(4, INPUT); //Ch3 pinMode(5, INPUT); //Ch4 pinMode(6, INPUT); //Ch5 pinMode(7, INPUT); //Ch6 pinMode(Motor1_Dir, OUTPUT); pinMode(Motor2_Dir, OUTPUT); pinMode(Motor1_PWM, OUTPUT); pinMode(Motor2_PWM, OUTPUT); digitalWrite(Motor1_Dir, LOW); // Motor 1 forward digitalWrite(Motor2_Dir, LOW); // Motor 2 forward } void loop() { for (int i = 1; i < 3; i++) // check channel 2 and 3 (from 0 to 3) { channel[i] = pulseIn(pins[i], HIGH, 25000); // Read the channel pulse channel[i] = (channel[i] == 0) ? 990 : channel[i]; // If there is an error the value is 0 /***************************************** * Same as this but shorter: * if(channel[i] == 0) * { * channel[i] = 990; * } * else * { * channel[i] = channel[i]; * } *****************************************/ pwm[i] = map(channel[i], 990, 2000, -255, 255); // Convert from (990 - 2000) to (-255 - 255) if (i == 2) // If channel 3 is checked (vertical movement) { reverse = (pwm[i] < 2) ? 1 : 0; // Forward or backward pwm[i] = (pwm[i] < 0) ? (255 + pwm[i]) : pwm[i]; // if backward, instead of (-255 to 0) => (0 to 255) } if ((prev_pwm[i] + 4 < pwm[i]) || (prev_pwm[i] - 4 > pwm[i])) // If there is a minimal movement of 4 in one direction { /** Define motor 1 speed **/ pwm_m1 = pwm[2] - pwm[1]; pwm_m1 = (pwm_m1 < 0)? 0: pwm_m1; // Minimum 0, not negative pwm_m1 = (pwm_m1 > 255)? 255: pwm_m1; // Maximum 255, no more /** Define motor 2 speed **/ pwm_m2 = pwm[2] + pwm[1]; pwm_m2 = (pwm_m2 < 0)? 0: pwm_m2; // Minimum 0, not negative pwm_m2 = (pwm_m2 > 255)? 255: pwm_m2; // Maximum 255, no more analogWrite(Motor1_PWM, pwm_m1); // Write speed to the motors analogWrite(Motor2_PWM, pwm_m2); prev_pwm[i] = pwm[i]; // Place here to check for minimal movement of 4 (see up) } if (reverse != prev_reverse) // If the direction is changed { digitalWrite(Motor1_Dir, reverse); // Write it to the H bridge digitalWrite(Motor2_Dir, reverse); // Write it to the H bridge prev_reverse = reverse; // Define for later checking } } delay(100); // sleep a small time }
So that's all the code.
Attachments
Step 6: Resume
So that's it, you've made a car that you can control with almost every remote.
Enjoy making and driving it!
If you liked it, please vote for it and share it.
If you have any ideas for me what to do next, please share them!
If you have questions, let them know in the comments.
And a Happy New year!
Laurens
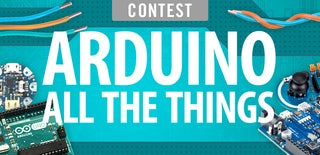
Participated in the
Arduino All The Things! Contest