Introduction: Making Countdown Alarm Using Arduino
Welcome, fellow creators and sonic visionaries, to the epicenter of innovation – an instructable poised to unravel the secrets of crafting a symphony of noise and technology. In the spirit of the "Make Some Noise Contest," we embark on a journey where Arduino Uno becomes our maestro, a servo motor our percussionist, and a metal bell our instrument of choice. Nestled within the folds of this instructable lies the art of engineering sound, amplified by the visual allure of an OLED display counting down to the crescendo of your imagination.
In this step-by-step guide, we'll delve into the intricacies of melding code, circuitry, and craftsmanship to birth an Arduino-based countdown alarm. But this is no ordinary alarm – it's a mechanical marvel that harmonizes the digital pulse of the microcontroller with the rhythmic clangor of a metal bell. The countdown, rendered on a mesmerizing OLED display, becomes the overture to an auditory spectacle, captivating not only the ears but also the eyes.
As a special twist, we'll explore the realms of Tinkercad and Fusion 360, leveraging these design titans to encapsulate our creation in a bespoke 3D-printed casing. Your noise-making device won't merely be heard; it will be a visual and auditory symphony, an ode to the convergence of technology and art.
Whether you're a seasoned tinkerer or a novice navigating the circuitry for the first time, this instructable welcomes you. Let's transcend the boundaries of silence, break free from the mundane, and craft a noise-making opus that stands as a testament to your creativity. Get ready to immerse yourself in the marriage of code, circuits, and craftsmanship – for in this instructable, noise isn't just a byproduct; it's a celebration, an innovation, and a form of expression. Let the instructions commence, and may your noise reverberate through the halls of ingenuity!
Supplies
- Arduino Uno
- Servo Motor
- Metal Bell with Screw
- OLED Display
- Jumper Wires
- Breadboard
- Resistors (220 ohms)
- Pushbutton
- Piezo Buzzer
- 10k Potentiometer
- Screws and Nuts
- Screwdriver
Step 1: Set Up the Circuit
- Connect the OLED display to the Arduino using the I2C protocol.
- Connect the servo motor to a PWM pin on the Arduino.
- Connect the pushbutton to the Arduino for starting the countdown.
Step 2: Assemble the Metal Bell
Step 3: Write the Arduino Code
// Include necessary libraries
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <Servo.h>
// Define OLED reset pin
#define OLED_RESET 4
Adafruit_SSD1306 display(OLED_RESET);
Servo alarmServo;
// Define pin for start button
const int buttonPin = 2;
// Define pins for servo
const int servoPin = 9;
int countdown = 10; // Initial countdown time in seconds
void setup() {
// Initialize OLED display
display.begin(SSD1306_I2C, 0x3C);
display.display();
delay(2000);
display.clearDisplay();
// Set up pins
pinMode(buttonPin, INPUT_PULLUP);
pinMode(servoPin, OUTPUT);
// Attach servo
alarmServo.attach(servoPin);
}
void loop() {
// Display countdown on OLED
display.clearDisplay();
display.setTextSize(2);
display.setTextColor(SSD1306_WHITE);
display.setCursor(10, 10);
display.print("Countdown: ");
display.print(countdown);
display.display();
// Check if the start button is pressed
if (digitalRead(buttonPin) == LOW) {
countdownTimer();
}
}
void countdownTimer() {
// Countdown loop
for (int i = countdown; i > 0; i--) {
display.clearDisplay();
display.setTextSize(2);
display.setTextColor(SSD1306_WHITE);
display.setCursor(10, 10);
display.print("Countdown: ");
display.print(i);
display.display();
delay(1000);
}
// Activate alarm
activateAlarm();
}
void activateAlarm() {
// Swing the metal bell with the servo
for (int angle = 0; angle <= 90; angle += 5) {
alarmServo.write(angle);
delay(50);
}
delay(2000);
// Return the metal bell to the initial position
for (int angle = 90; angle >= 0; angle -= 5) {
alarmServo.write(angle);
delay(50);
}
}
Step 4: Test Your Alarm
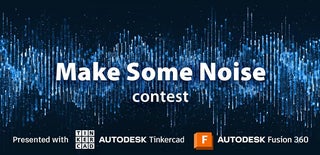
Participated in the
Make Some Noise Contest