Introduction: MoonLight
This project uses an RGB LED to display the phases of the moon. The light is off for a new moon. For a waxing the light is white and gets brighter every day. On the full moon the light is magenta. Then for a waning moon the light is yellow and gets dimmer every day.
Parts list:
Step 1: Build It
Follow the photo and the diagram to build the circuit.
The longest lead on the LED is the anode. It connects to red wire.
The 330 Ohm resistors connect to the red and green leads and the 680 Ohm resistor connects to the blue lead.
This RGB LED is a common anode LED meaning the anode needs to be connected to positive voltage and the cathodes are connected to the pins. Take care obtaining the LED because there are also common Cathode RGB LEDs available. I used the common anode variety because I think they are more common.
Step 2:
Copy the following code into the Arduino IDE:
/*********************************************************** * Filename: MoonLight.ino by JRV31 * * Uses an RGB LED to display the correct phase of the moon. * Off = new moon * White = waxing moon * Magenta = full moon * Yellow = waning moon * * To find current day in the moon's cycle go to * http://www.calendar-365.com/moon/moon-calendar.html * and count from zero starting at the prevoius new moon * until today's date. * Your answer goes in the day variable. * (The first line of the code.) * ***********************************************************/ int day = 0; // Day in moon cycle, new moon = 0. int time = 86400000; // 24 hours = 86400000 int led[3] = {9,10,11}; // Array for the RGB LED. int brit[29] = {255,254,253,252,251,249,247,243,239,231,223,207,191,159, 96,0,96,127,159,191,207,223,231,239,243,247,249,252,254}; void setup() { for(int i = 0; i < 3; i++) { pinMode(led[i], OUTPUT); // RGB LED setup for output. } } void loop() { if(day==15) { analogWrite(led[0], brit[day]); //magenta analogWrite(led[2], brit[day]); } else if(day>15) { analogWrite(led[0], brit[day]); //yellow analogWrite(led[1], brit[day]); } else { analogWrite(led[0], brit[day]); //white analogWrite(led[1], brit[day]); analogWrite(led[2], brit[day]); } delay(time); for(int i=0; i<3; i++) digitalWrite(led[i], HIGH); // all off day++; if(day==29) day=0; }
To find current day in the moon's cycle go to
http://www.calendar-365.com/moon/moon-calendar.html
and count from zero starting at the prevoius new moon until today's date. Your answer goes in the day variable. (The first line of the code.)
It takes a full cycle of the moon to go through the program, so to test it change the time variable (the second line of the code) to 500 to test.
Attachments
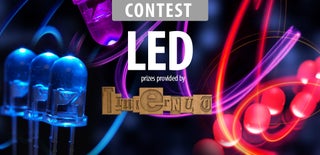
Participated in the
LED Contest