Introduction: #MyoCraft: Myo Armband With Node.js on Intel Edison Board
In this insctructable, I will show you how to control your Intel Edison Board with the movements of your hand and your arm, thanks to your Myo Armband.
The different steps described below use bluetooth protocol which connects Myo and Edison Board through Node.js.
In order to help you, I created a Github project that you can find below :
https://github.com/katlea/node-myo-edison
Step 1: Requirements
- A Myo Armband : https://store.myo.com/?utm_source=Instructables&u...
- Update to the latest Myo Firmware !
- An Intel Edison Board
- SSH or Serial access to your edison board
Step 2: Configure Intel Edison Board
First you need to start wifi on Edison board :
configure_edison --wifi
then follow the instructions on screen.
Optional : to enable SFTP access you need to activate a password on the board
configure_edison --password
then follow the instructions on screen.
Create a directory for the Node.js project
cd ~ mkdir myo cd myo
Install Noble package in the project directory
npm install noble
We need to update the repository list on the Edison (if you have already not done that).
Start 'vi' editor to edit the file /etc/opkg/base-feeds.conf
vi /etc/opkg/base-feeds.conf
Then insert the content below :
src/gz all http://repo.opkg.net/edison/repo/all
src/gz edison http://repo.opkg.net/edison/repo/edison
src/gz core2-32 http://repo.opkg.net/edison/repo/core2-32
Now update opkg and then install Bluez package
Bluez package is for Bluetooth Low Energy control.
opkg update
opkg install bluez5-dev
Step 3: Configure Bluetooth Low Energy on Start
Original instructions to activate Bluetooth:
rfkill unblock bluetooth killall bluetoothd hciconfig hci0 up
You need to create a file in "/etc/init.d" to activate Bluetooth Low Energy on startup.
The file is called "ble".
Create a directory
cd /etc mkdir init.d cd init.d
Then edit "ble" file.
vi ble
Copy the file content below into "ble" file.
#!/bin/sh start(){ rfkill unblock bluetooth killall bluetoothd hciconfig hci0 up echo "ble up" } stop(){ hciconfig hci0 down echo "ble down" } case "$1" in start) start ;; stop) stop ;; restart) stop start ;; esac exit 0
Enable "ble" file to be executable :
chmod +x ble
Then launch the file:
/etc/init.d/ble start
To launch the file on board startup:
update-rc.d ble defaults
Step 4: Install Script
Go into your project directory
cd ~ cd myo
Download myo.js from my github repository
https://github.com/katlea/node-myo-edison
Or from myo.js attachment
Then send it to the project directory (via SFTP for example)
Step 5: Enjoy Node.js Action
Create a file "app.js" in project directory.
And use the documentation below to fill it.
Then run :
node app.js
Usage
var myo = require('./myo.js');
CONNECT
Quick Connect
myo.quickConnect(function(err, id){
console.log('myo unique id : ', id); });
Classic Connect
myo.scan.start(function(err, data){
console.log(err, data); }); myo.event.on('ready', function(id){ console.log('myo unique id : ', id); });
DISCONNECT
myo.connected[id]..disconnect();
INITIATE
Initiate Myo to receive stream and data
myo.connected[id].unlock("hold", function() {
// lock - time (will lock after inactivity) - hold myo.connected[id].sleepMode("forever", function () { // normal - forever (never sleep) myo.connected[id].setMode('send', 'all', 'enabled', function () { // emg : none - send - raw // imu : none - data - events - all - raw // classifier : enabled - disabled console.log('initiated'); }); }); });
INTERACTION
Get Name
myo.connected[id].generic.getName(function (err, data){ // Get device name
console.log(err, data); });
Set Name
myo.connected[id].generic.setName('Myo NAME', function (err, data){ // Set device name
console.log(err, data); });
Battery Info
myo.connected[id].battery(function(err, data) {
console.log("battery : " + data + " %"); // data => battery in percent });
Vibrate classic
myo.connected[id].vibrate("strong"); // light, medium, strong
Vibrate custom
myo.connected[id].vibrate2(1500, 255); // time in milliseconds, power 0 - 255
Vibrate notify
myo.connected[id].notify(); // notify : short and light vibration
Deep sleep
myo.connected[id].deepSleep(function(){}); // go into deep sleep
Basic Info
myo.connected[id].info(function(err, data){
console.log(err, data); });
Firmware Info
myo.connected[id].firmware(function(err, data){
console.log(err, data); });
STREAM
Set "true" to get stream on events or "false" to disable.
imu (motion)
myo.connected[id].imu(true);
Classifier
myo.connected[id].classifier(true);
emg
myo.connected[id].emg(true);
EVENTS
myo.event.on('discover', function(id){
console.log('discover', id); }); myo.event.on('connect', function(id){ console.log('connect', id); }); myo.event.on('disconnect', function(id){ console.log('disconnect', id); }); myo.event.on('ready', function(id){ console.log('peripheral ready :', id); }); myo.event.on('imu', function(data){ console.log('imu', data); }); myo.event.on('classifier', function(data){ console.log('classifier', data); }); myo.event.on('emg4', function(data){ console.log('emg', data); });
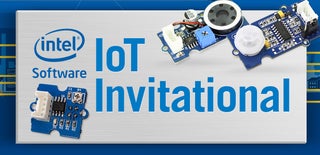
Participated in the
Intel® IoT Invitational